Fork of Smoothie to port to mbed non-LPC targets.
Fork of Smoothie by
Panel.h
00001 /* 00002 This file is part of Smoothie (http://smoothieware.org/). The motion control part is heavily based on Grbl (https://github.com/simen/grbl). 00003 Smoothie is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version. 00004 Smoothie is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. 00005 You should have received a copy of the GNU General Public License along with Smoothie. If not, see <http://www.gnu.org/licenses/>. 00006 */ 00007 00008 #ifndef PANEL_H 00009 #define PANEL_H 00010 00011 #include "Kernel.h" 00012 #include "PanelScreen.h" 00013 #include "panels/LcdBase.h" 00014 #include "Button.h" 00015 00016 #define MENU_MODE 0 00017 #define CONTROL_MODE 1 00018 00019 class PanelScreen; 00020 class Panel : public Module { 00021 public: 00022 Panel(); 00023 virtual ~Panel(); 00024 00025 void on_module_loaded(); 00026 uint32_t button_tick(uint32_t dummy); 00027 void on_idle(void* argument); 00028 void on_main_loop(void* argument); 00029 void on_gcode_received(void* argument); 00030 void enter_screen(PanelScreen* screen); 00031 void reset_counter(); 00032 00033 // Encoder and buttons 00034 uint32_t on_up(uint32_t dummy); 00035 uint32_t on_down(uint32_t dummy); 00036 uint32_t on_back(uint32_t dummy); 00037 uint32_t on_select(uint32_t dummy); 00038 uint32_t on_pause(uint32_t dummy); 00039 uint32_t refresh_tick(uint32_t dummy); 00040 uint32_t encoder_check(uint32_t dummy); 00041 bool counter_change(); 00042 bool click(); 00043 int get_encoder_resolution() const { return encoder_click_resolution; } 00044 00045 // Menu 00046 void enter_menu_mode(); 00047 void setup_menu(uint16_t rows, uint16_t lines); 00048 void setup_menu(uint16_t rows); 00049 void menu_update(); 00050 bool menu_change(); 00051 uint16_t max_screen_lines() { return screen_lines; } 00052 uint16_t get_menu_current_line() { return menu_current_line; } 00053 00054 // Control 00055 bool enter_control_mode(float passed_normal_increment, float passed_pressed_increment); 00056 void set_control_value(float value); 00057 float get_control_value(); 00058 bool control_value_change(); 00059 void control_value_update(); 00060 float get_jogging_speed(char axis) { return jogging_speed_mm_min[axis-'X']; } 00061 float get_default_hotend_temp() { return default_hotend_temperature; } 00062 float get_default_bed_temp() { return default_bed_temperature; } 00063 00064 // file playing from sd 00065 bool is_playing() const; 00066 void set_playing_file(string f); 00067 const char* get_playing_file() { return playing_file; } 00068 00069 string getMessage() { return message; } 00070 bool hasMessage() { return message.size() > 0; } 00071 00072 // public as it is directly accessed by screens... not good 00073 // TODO pass lcd into ctor of each sub screen 00074 LcdBase* lcd; 00075 PanelScreen* custom_screen; 00076 00077 // as panelscreen accesses private fields in Panel 00078 friend class PanelScreen; 00079 00080 private: 00081 // Menu 00082 char menu_offset; 00083 int menu_selected_line; 00084 int menu_start_line; 00085 int menu_rows; 00086 int panel_lines; 00087 bool menu_changed; 00088 bool control_value_changed; 00089 uint16_t menu_current_line; 00090 00091 // Control 00092 float normal_increment; 00093 int control_normal_counter; 00094 float control_base_value; 00095 00096 Button up_button; 00097 Button down_button; 00098 Button back_button; 00099 Button click_button; 00100 Button pause_button; 00101 00102 int* counter; 00103 volatile bool counter_changed; 00104 volatile bool click_changed; 00105 volatile bool refresh_flag; 00106 volatile bool do_buttons; 00107 bool paused; 00108 int idle_time; 00109 bool start_up; 00110 int encoder_click_resolution; 00111 char mode; 00112 uint16_t screen_lines; 00113 00114 PanelScreen* top_screen; 00115 PanelScreen* current_screen; 00116 00117 float jogging_speed_mm_min[3]; 00118 float default_hotend_temperature; 00119 float default_bed_temperature; 00120 00121 char playing_file[20]; 00122 string message; 00123 }; 00124 00125 #endif
Generated on Tue Jul 12 2022 20:09:02 by
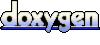