Fork of Smoothie to port to mbed non-LPC targets.
Fork of Smoothie by
PID_Autotuner.h
00001 /** 00002 * Based on https://github.com/br3ttb/Arduino-PID-AutoTune-Library 00003 */ 00004 00005 #ifndef _PID_AUTOTUNE_H 00006 #define _PID_AUTOTUNE_H 00007 00008 #include <stdint.h> 00009 00010 #include "Module.h" 00011 #include "TemperatureControl.h" 00012 #include "StreamOutput.h" 00013 00014 class PID_Autotuner : public Module 00015 { 00016 public: 00017 PID_Autotuner(); 00018 void begin(TemperatureControl *, float, StreamOutput *, int cycles = 8); 00019 void abort(); 00020 00021 void on_module_loaded(void); 00022 uint32_t on_tick(uint32_t); 00023 void on_idle(void *); 00024 void on_gcode_received(void *); 00025 00026 private: 00027 void finishUp(); 00028 00029 TemperatureControl *t; 00030 float target_temperature; 00031 StreamOutput *s; 00032 00033 volatile bool tick; 00034 00035 float *peaks; 00036 int requested_cycles; 00037 float noiseBand; 00038 unsigned long peak1, peak2; 00039 int sampleTime; 00040 int nLookBack; 00041 int lookBackCnt; 00042 int peakType; 00043 float *lastInputs; 00044 int peakCount; 00045 bool justchanged; 00046 float absMax, absMin; 00047 float oStep; 00048 int output; 00049 unsigned long tickCnt; 00050 }; 00051 00052 #endif /* _PID_AUTOTUNE_H */
Generated on Tue Jul 12 2022 20:09:02 by
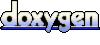