Fork of Smoothie to port to mbed non-LPC targets.
Fork of Smoothie by
MemoryPool.h
00001 #ifndef _MEMORYPOOL_H 00002 #define _MEMORYPOOL_H 00003 00004 #include <stdint.h> 00005 // #include <cstdio> 00006 #include <stdlib.h> 00007 00008 #ifdef MEMDEBUG 00009 #define MDEBUG(...) printf(__VA_ARGS__) 00010 #else 00011 #define MDEBUG(...) do {} while (0) 00012 #endif 00013 00014 #include "StreamOutput.h" 00015 00016 /* 00017 * with MUCH thanks to http://www.parashift.com/c++-faq-lite/memory-pools.html 00018 * 00019 * test framework at https://gist.github.com/triffid/5563987 00020 */ 00021 00022 class MemoryPool 00023 { 00024 public: 00025 MemoryPool(void* base, uint16_t size); 00026 ~MemoryPool(); 00027 00028 void* alloc(size_t); 00029 void dealloc(void* p); 00030 00031 void debug(StreamOutput*); 00032 00033 bool has(void*); 00034 00035 uint32_t free(void); 00036 00037 MemoryPool* next; 00038 00039 static MemoryPool* first; 00040 00041 private: 00042 void* base; 00043 uint16_t size; 00044 }; 00045 00046 // this overloads "placement new" 00047 inline void* operator new(size_t nbytes, MemoryPool& pool) 00048 { 00049 return pool.alloc(nbytes); 00050 } 00051 00052 // this allows placement new to free memory if the constructor fails 00053 inline void operator delete(void* p, MemoryPool& pool) 00054 { 00055 pool.dealloc(p); 00056 } 00057 00058 // this catches all usages of delete blah. The object's destructor is called before we get here 00059 // it first checks if the deleted object is part of a pool, and uses free otherwise. 00060 inline void operator delete(void* p) 00061 { 00062 MemoryPool* m = MemoryPool::first; 00063 while (m) 00064 { 00065 if (m->has(p)) 00066 { 00067 MDEBUG("Pool %p has %p, using dealloc()\n", m, p); 00068 m->dealloc(p); 00069 return; 00070 } 00071 m = m->next; 00072 } 00073 00074 MDEBUG("no pool has %p, using free()\n", p); 00075 free(p); 00076 } 00077 00078 #endif /* _MEMORYPOOL_H */
Generated on Tue Jul 12 2022 20:09:02 by
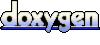