Fork of Smoothie to port to mbed non-LPC targets.
Fork of Smoothie by
MainMenuScreen.cpp
00001 /* 00002 This file is part of Smoothie (http://smoothieware.org/). The motion control part is heavily based on Grbl (https://github.com/simen/grbl). 00003 Smoothie is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version. 00004 Smoothie is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. 00005 You should have received a copy of the GNU General Public License along with Smoothie. If not, see <http://www.gnu.org/licenses/>. 00006 */ 00007 00008 #include "libs/Kernel.h" 00009 #include "Panel.h" 00010 #include "PanelScreen.h" 00011 #include "MainMenuScreen.h" 00012 #include "WatchScreen.h" 00013 #include "FileScreen.h" 00014 #include "ControlScreen.h" 00015 #include "PrepareScreen.h" 00016 #include "libs/nuts_bolts.h" 00017 #include "libs/utils.h" 00018 #include "modules/utils/player/PlayerPublicAccess.h" 00019 00020 00021 #include <string> 00022 using namespace std; 00023 00024 MainMenuScreen::MainMenuScreen() 00025 { 00026 // Children screens 00027 this->jog_screen = (new JogScreen() )->set_parent(this); 00028 this->watch_screen = (new WatchScreen() )->set_parent(this); 00029 this->file_screen = (new FileScreen() )->set_parent(this); 00030 this->prepare_screen = (new PrepareScreen() )->set_parent(this); 00031 this->set_parent(this->watch_screen); 00032 } 00033 00034 void MainMenuScreen::on_enter() 00035 { 00036 this->panel->enter_menu_mode(); 00037 this->panel->setup_menu(5); 00038 this->refresh_menu(); 00039 } 00040 00041 void MainMenuScreen::on_refresh() 00042 { 00043 if ( this->panel->menu_change() ) { 00044 this->refresh_menu(); 00045 } 00046 if ( this->panel->click() ) { 00047 this->clicked_menu_entry(this->panel->get_menu_current_line()); 00048 } 00049 } 00050 00051 void MainMenuScreen::display_menu_line(uint16_t line) 00052 { 00053 switch ( line ) { 00054 case 0: this->panel->lcd->printf("Watch"); break; 00055 case 1: this->panel->lcd->printf(panel->is_playing() ? "Abort" : "Play"); break; 00056 case 2: this->panel->lcd->printf("Jog"); break; 00057 case 3: this->panel->lcd->printf("Prepare"); break; 00058 case 4: this->panel->lcd->printf("Custom"); break; 00059 //case 4: this->panel->lcd->printf("Configure"); break; 00060 //case 5: this->panel->lcd->printf("Tune"); break; 00061 } 00062 } 00063 00064 void MainMenuScreen::clicked_menu_entry(uint16_t line) 00065 { 00066 switch ( line ) { 00067 case 0: this->panel->enter_screen(this->watch_screen ); break; 00068 case 1: this->panel->is_playing() ? abort_playing() : this->panel->enter_screen(this->file_screen); break; 00069 case 2: this->panel->enter_screen(this->jog_screen ); break; 00070 case 3: this->panel->enter_screen(this->prepare_screen ); break; 00071 case 4: this->panel->enter_screen(this->panel->custom_screen ); break; 00072 } 00073 } 00074 00075 void MainMenuScreen::abort_playing() 00076 { 00077 THEKERNEL->public_data->set_value(player_checksum, abort_play_checksum, NULL); 00078 this->panel->enter_screen(this->watch_screen); 00079 } 00080
Generated on Tue Jul 12 2022 20:09:02 by
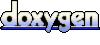