Fork of Smoothie to port to mbed non-LPC targets.
Fork of Smoothie by
LcdBase.h
00001 #ifndef LCDBASE_H 00002 #define LCDBASE_H 00003 00004 #include "stdint.h" 00005 00006 // Standard directional button bits 00007 #define BUTTON_SELECT 0x01 00008 #define BUTTON_RIGHT 0x02 00009 #define BUTTON_DOWN 0x04 00010 #define BUTTON_UP 0x08 00011 #define BUTTON_LEFT 0x10 00012 #define BUTTON_PAUSE 0x20 00013 #define BUTTON_AUX1 0x40 00014 #define BUTTON_AUX2 0x80 00015 00016 // specific LED assignments 00017 #define LED_FAN_ON 1 00018 #define LED_HOTEND_ON 2 00019 #define LED_BED_ON 3 00020 00021 class Panel; 00022 00023 class LcdBase { 00024 public: 00025 LcdBase(); 00026 virtual ~LcdBase(); 00027 virtual void init()= 0; 00028 // only use this to display lines 00029 int printf(const char* format, ...); 00030 00031 void setPanel(Panel* p) { panel= p; } 00032 00033 // Required LCD functions 00034 virtual void home()= 0; 00035 virtual void clear()= 0; 00036 virtual void display()= 0; 00037 virtual void setCursor(uint8_t col, uint8_t row)= 0; 00038 00039 // Returns button states including the encoder select button 00040 virtual uint8_t readButtons()= 0; 00041 00042 // returns the current encoder position 00043 virtual int readEncoderDelta()= 0; 00044 00045 // the number of encoder clicks per detent. this is divided into 00046 // accumulated clicks for control values so one detent is one 00047 // increment, this varies depending on encoder type usually 1,2 or 4 00048 virtual int getEncoderResolution()= 0; 00049 00050 // optional 00051 virtual void setLed(int led, bool onoff){}; 00052 virtual void setLedBrightness(int led, int val){}; 00053 virtual void buzz(long,uint16_t){}; 00054 virtual bool hasGraphics() { return false; } 00055 // on graphics panels, the input bitmap is in X windows XBM format but 00056 // with the bits in a byte reversed so bit7 is left most and bit0 is 00057 // right most. x_offset must by byte aligned if used 00058 virtual void bltGlyph(int x, int y, int w, int h, const uint8_t *glyph, int span= 0, int x_offset=0, int y_offset=0){} 00059 // only used on certain panels 00060 virtual void on_refresh(bool now= false){}; 00061 virtual void on_main_loop(){}; 00062 // override this if the panel can handle more or less screen lines 00063 virtual uint16_t get_screen_lines() { return 4; } 00064 // used to set a variant for a panel (like viki vs panelolou2) 00065 virtual void set_variant(int n) {}; 00066 00067 protected: 00068 Panel* panel; 00069 virtual void write(const char* line, int len)= 0; 00070 00071 }; 00072 00073 #endif // LCDBASE_H 00074
Generated on Tue Jul 12 2022 20:09:02 by
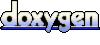