Fork of Smoothie to port to mbed non-LPC targets.
Fork of Smoothie by
HeapRing.h
00001 #ifndef _HEAPRING_H 00002 #define _HEAPRING_H 00003 00004 template<class kind> class HeapRing { 00005 00006 // smoothie-specific friend classes 00007 friend class Planner; 00008 friend class Conveyor; 00009 friend class Block; 00010 00011 public: 00012 HeapRing(); 00013 HeapRing(unsigned int length); 00014 00015 ~HeapRing(); 00016 00017 /* 00018 * direct accessors 00019 */ 00020 kind& head(); 00021 kind& tail(); 00022 00023 void push_front(kind&) __attribute__ ((warning("Not thread-safe if pop_back() is used in ISR context!"))); // instead, prepare(head_ref()); produce_head(); 00024 kind& pop_back(void) __attribute__ ((warning("Not thread-safe if head_ref() is used to prepare new items, or push_front() is used in ISR context!"))); // instead, consume(tail_ref()); consume_tail(); 00025 00026 /* 00027 * pointer accessors 00028 */ 00029 kind* head_ref(); 00030 kind* tail_ref(); 00031 00032 void produce_head(void); 00033 void consume_tail(void); 00034 00035 /* 00036 * queue status 00037 */ 00038 bool is_empty(void); 00039 bool is_full(void); 00040 00041 /* 00042 * resize 00043 * 00044 * returns true on success, or false if queue is not empty or not enough memory available 00045 */ 00046 bool resize(unsigned int); 00047 00048 /* 00049 * provide 00050 * kind* - new buffer pointer 00051 * int length - number of items in buffer (NOT size in bytes!) 00052 * 00053 * cause HeapRing to use a specific memory location instead of allocating its own 00054 * 00055 * returns true on success, or false if queue is not empty 00056 */ 00057 bool provide(kind*, unsigned int length); 00058 00059 protected: 00060 /* 00061 * these functions are protected as they should only be used internally 00062 * or in extremely specific circumstances 00063 */ 00064 kind& item(unsigned int); 00065 kind* item_ref(unsigned int); 00066 00067 unsigned int next(unsigned int); 00068 unsigned int prev(unsigned int); 00069 00070 /* 00071 * buffer variables 00072 */ 00073 unsigned int length; 00074 00075 volatile unsigned int head_i; 00076 volatile unsigned int tail_i; 00077 00078 private: 00079 kind* ring; 00080 }; 00081 00082 #endif /* _HEAPRING_H */
Generated on Tue Jul 12 2022 20:09:02 by
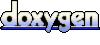