Fork of Smoothie to port to mbed non-LPC targets.
Fork of Smoothie by
FirmConfigSource.cpp
00001 /* 00002 This file is part of Smoothie (http://smoothieware.org/). The motion control part is heavily based on Grbl (https://github.com/simen/grbl). 00003 Smoothie is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version. 00004 Smoothie is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. 00005 You should have received a copy of the GNU General Public License along with Smoothie. If not, see <http://www.gnu.org/licenses/>. 00006 */ 00007 00008 #include "libs/Kernel.h" 00009 #include "ConfigValue.h" 00010 #include "FirmConfigSource.h" 00011 #include "ConfigCache.h" 00012 00013 00014 using namespace std; 00015 #include <string> 00016 00017 // we use objdump in the Makefile to import your config.default file into the compiled code 00018 // Since the two symbols below are derived from the filename, we need to change them if the filename changes 00019 extern char _binary_config_default_start; 00020 extern char _binary_config_default_end; 00021 00022 00023 FirmConfigSource::FirmConfigSource(uint16_t name_checksum){ 00024 this->name_checksum = name_checksum; 00025 } 00026 00027 // Transfer all values found in the file to the passed cache 00028 void FirmConfigSource::transfer_values_to_cache( ConfigCache* cache ){ 00029 00030 char* p = &_binary_config_default_start; 00031 // For each line 00032 while( p != &_binary_config_default_end ){ 00033 process_char_from_ascii_config(*p++, cache); 00034 } 00035 } 00036 00037 // Return true if the check_sums match 00038 bool FirmConfigSource::is_named( uint16_t check_sum ){ 00039 return check_sum == this->name_checksum; 00040 } 00041 00042 // Write a config setting to the file *** FirmConfigSource is read only *** 00043 void FirmConfigSource::write( string setting, string value ){ 00044 //THEKERNEL->streams->printf("ERROR: FirmConfigSource is read only\r\n"); 00045 } 00046 00047 // Return the value for a specific checksum 00048 string FirmConfigSource::read( uint16_t check_sums[3] ){ 00049 00050 string value = ""; 00051 00052 char* p = &_binary_config_default_start; 00053 // For each line 00054 while( p != &_binary_config_default_end ){ 00055 value = process_char_from_ascii_config(*p++, check_sums); 00056 if (value.length()) 00057 return value; 00058 } 00059 00060 return value; 00061 } 00062
Generated on Tue Jul 12 2022 20:09:01 by
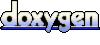