Fork of Smoothie to port to mbed non-LPC targets.
Fork of Smoothie by
FPointer.h
00001 /* 00002 Copyright (c) 2011 Andy Kirkham 00003 Permission is hereby granted, free of charge, to any person obtaining a copy 00004 of this software and associated documentation files (the "Software"), to deal 00005 in the Software without restriction, including without limitation the rights 00006 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00007 copies of the Software, and to permit persons to whom the Software is 00008 furnished to do so, subject to the following conditions: 00009 The above copyright notice and this permission notice shall be included in 00010 all copies or substantial portions of the Software. 00011 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00012 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00013 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00014 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00015 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00017 THE SOFTWARE. 00018 */ 00019 00020 #ifndef AJK_FPOINTER_H 00021 #define AJK_FPOINTER_H 00022 00023 #ifndef NULL 00024 #define NULL 0 00025 #endif 00026 00027 #include <cstdint> 00028 00029 namespace AjK { 00030 00031 class FPointerDummy; 00032 00033 /** FPointer - Adds callbacks that take and return a 32bit uint32_t data type. 00034 * 00035 * The Mbed library supplies a callback using the FunctionPointer object as 00036 * defined in FunctionPointer.h However, this callback system does not allow 00037 * the caller to pass a value to the callback. Likewise, the callback itself 00038 * cannot return a value. 00039 * 00040 * FPointer operates in the same way but allows the callback function to be 00041 * passed one arg, a uint32_t value. Additionally, the callback can return 00042 * a single uint32_t value. The reason for using uint32_t is that the Mbed 00043 * and the microcontroller (LPC1768) have a natural data size of 32bits and 00044 * this means we can use the uint32_t as a pointer. See example1.h for more 00045 * information. This example passes an "int" by passing a pointer to that 00046 * int as a 32bit value. Using this technique you can pass any value you like. 00047 * All you have to do is pass a pointer to your value cast to (uint32_t). Your 00048 * callback can the deference it to get the original value. 00049 * 00050 * example2.h shows how to do the same thing but demostrates how to specify 00051 * the callback into a class object/method. 00052 * 00053 * Finally, example3.h shows how to pass multiple values. In this example we 00054 * define a data structure and in the callback we pass a pointer to that 00055 * data structure thus allowing the callback to again get the values. 00056 * 00057 * Note, when passing pointers to variables to the callback, if the callback 00058 * function/method changes that variable's value then it will also change the 00059 * value the caller sees. If C pointers are new to you, you are strongly 00060 * advised to read up on the subject. It's pointers that often get beginners 00061 * into trouble when mis-used. 00062 * 00063 * @see example1.h 00064 * @see example2.h 00065 * @see example3.h 00066 * @see http://mbed.org/handbook/C-Data-Types 00067 * @see http://mbed.org/projects/libraries/svn/mbed/trunk/FunctionPointer.h 00068 */ 00069 class FPointer { 00070 00071 protected: 00072 00073 //! C callback function pointer. 00074 uint32_t (*c_callback)(uint32_t); 00075 00076 //! C++ callback object/method pointer (the object part). 00077 FPointerDummy *obj_callback; 00078 00079 //! C++ callback object/method pointer (the method part). 00080 uint32_t (FPointerDummy::*method_callback)(uint32_t); 00081 00082 public: 00083 00084 /** Constructor 00085 */ 00086 FPointer() { 00087 c_callback = NULL; 00088 obj_callback = NULL; 00089 method_callback = NULL; 00090 } 00091 00092 /** attach - Overloaded attachment function. 00093 * 00094 * Attach a C type function pointer as the callback. 00095 * 00096 * Note, the callback function prototype must be:- 00097 * @code 00098 * uint32_t myCallbackFunction(uint32_t); 00099 * @endcode 00100 * @param A C function pointer to call. 00101 */ 00102 void attach(uint32_t (*function)(uint32_t) = 0) { c_callback = function; } 00103 00104 /** attach - Overloaded attachment function. 00105 * 00106 * Attach a C++ type object/method pointer as the callback. 00107 * 00108 * Note, the callback method prototype must be:- 00109 * @code 00110 * public: 00111 * uint32_t myCallbackFunction(uint32_t); 00112 * @endcode 00113 * @param A C++ object pointer. 00114 * @param A C++ method within the object to call. 00115 */ 00116 template<class T> 00117 void attach(T* item, uint32_t (T::*method)(uint32_t)) { 00118 obj_callback = (FPointerDummy *)item; 00119 method_callback = (uint32_t (FPointerDummy::*)(uint32_t))method; 00120 } 00121 00122 /** call - Overloaded callback initiator. 00123 * 00124 * call the callback function. 00125 * 00126 * @param uint32_t The value to pass to the callback. 00127 * @return uint32_t The value the callback returns. 00128 */ 00129 uint32_t call(uint32_t arg) { 00130 if (c_callback != NULL) { 00131 return (*c_callback)(arg); 00132 } 00133 else { 00134 if (obj_callback != NULL && method_callback != NULL) { 00135 return (obj_callback->*method_callback)(arg); 00136 } 00137 } 00138 return (uint32_t)NULL; 00139 } 00140 00141 /** call - Overloaded callback initiator. 00142 * 00143 * Call the callback function without passing an argument. 00144 * The callback itself is passed NULL. Note, the callback 00145 * prototype should still be <b>uint32_t callback(uint32_t)</b>. 00146 * 00147 * @return uint32_t The value the callback returns. 00148 */ 00149 uint32_t call(void) { 00150 if (c_callback != NULL) { 00151 return (*c_callback)((uint32_t)NULL); 00152 } 00153 else { 00154 if (obj_callback != NULL && method_callback != NULL) { 00155 return (obj_callback->*method_callback)((uint32_t)NULL); 00156 } 00157 } 00158 return (uint32_t)NULL; 00159 } 00160 }; 00161 00162 }; // namespace AjK ends 00163 00164 using namespace AjK; 00165 00166 #endif
Generated on Tue Jul 12 2022 20:09:01 by
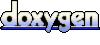