Fork of Smoothie to port to mbed non-LPC targets.
Fork of Smoothie by
FATFileSystem.cpp
00001 /* mbed Microcontroller Library - FATFileSystem 00002 * Copyright (c) 2008, sford 00003 */ 00004 00005 #include "FATFileSystem.h" 00006 00007 #include "mbed.h" 00008 00009 #include "FileSystemLike.h" 00010 #include "FATFileHandle.h" 00011 #include "FATDirHandle.h" 00012 #include "ff.h" 00013 //#include "Debug.h" 00014 #include <stdio.h> 00015 #include <stdlib.h> 00016 00017 DWORD get_fattime (void) { 00018 return 999; 00019 } 00020 00021 namespace mbed { 00022 00023 #if FFSDEBUG_ENABLED 00024 static const char *FR_ERRORS[] = { 00025 "FR_OK = 0", 00026 "FR_NOT_READY", 00027 "FR_NO_FILE", 00028 "FR_NO_PATH", 00029 "FR_INVALID_NAME", 00030 "FR_INVALID_DRIVE", 00031 "FR_DENIED", 00032 "FR_EXIST", 00033 "FR_RW_ERROR", 00034 "FR_WRITE_PROTECTED", 00035 "FR_NOT_ENABLED", 00036 "FR_NO_FILESYSTEM", 00037 "FR_INVALID_OBJECT", 00038 "FR_MKFS_ABORTED" 00039 }; 00040 #endif 00041 00042 FATFileSystem *FATFileSystem::_ffs[_DRIVES] = {0}; 00043 00044 FATFileSystem::FATFileSystem(const char* n) : FileSystemLike(n) { 00045 FFSDEBUG("FATFileSystem(%s)\n", n); 00046 for(int i=0; i<_DRIVES; i++) { 00047 if(_ffs[i] == 0) { 00048 _ffs[i] = this; 00049 _fsid = i; 00050 FFSDEBUG("Mounting [%s] on ffs drive [%d]\n", _name, _fsid); 00051 f_mount(i, &_fs); 00052 return; 00053 } 00054 } 00055 error("Couldn't create %s in FATFileSystem::FATFileSystem\n",n); 00056 } 00057 00058 FATFileSystem::~FATFileSystem() { 00059 for(int i=0; i<_DRIVES; i++) { 00060 if(_ffs[i] == this) { 00061 _ffs[i] = 0; 00062 f_mount(i, NULL); 00063 } 00064 } 00065 } 00066 00067 FileHandle *FATFileSystem::open(const char* name, int flags) { 00068 FFSDEBUG("open(%s) on filesystem [%s], drv [%d]\n", name, _name, _fsid); 00069 char n[64]; 00070 sprintf(n, "%d:/%s", _fsid, name); 00071 00072 /* POSIX flags -> FatFS open mode */ 00073 BYTE openmode; 00074 if(flags & O_RDWR) { 00075 openmode = FA_READ|FA_WRITE; 00076 } else if(flags & O_WRONLY) { 00077 openmode = FA_WRITE; 00078 } else { 00079 openmode = FA_READ; 00080 } 00081 if(flags & O_CREAT) { 00082 if(flags & O_TRUNC) { 00083 openmode |= FA_CREATE_ALWAYS; 00084 } else { 00085 openmode |= FA_OPEN_ALWAYS; 00086 } 00087 } 00088 00089 FIL_t fh; 00090 FRESULT res = f_open(&fh, n, openmode); 00091 if(res) { 00092 FFSDEBUG("f_open('w') failed (%d, %s)\n", res, FR_ERRORS[res]); 00093 return NULL; 00094 } 00095 if(flags & O_APPEND) { 00096 f_lseek(&fh, fh.fsize); 00097 } 00098 return new FATFileHandle(fh); 00099 } 00100 00101 int FATFileSystem::remove(const char *filename) { 00102 FRESULT res = f_unlink(filename); 00103 if(res) { 00104 FFSDEBUG("f_unlink() failed (%d, %s)\n", res, FR_ERRORS[res]); 00105 return -1; 00106 } 00107 return 0; 00108 } 00109 00110 int FATFileSystem::format() { 00111 FFSDEBUG("format()\n"); 00112 FRESULT res = f_mkfs(_fsid, 0, 512); // Logical drive number, Partitioning rule, Allocation unit size (bytes per cluster) 00113 if(res) { 00114 FFSDEBUG("f_mkfs() failed (%d, %s)\n", res, FR_ERRORS[res]); 00115 return -1; 00116 } 00117 return 0; 00118 } 00119 00120 DirHandle *FATFileSystem::opendir(const char *name) { 00121 DIR_t dir; 00122 FRESULT res = f_opendir(&dir, name); 00123 if(res != 0) { 00124 return NULL; 00125 } 00126 return new FATDirHandle(dir); 00127 } 00128 00129 int FATFileSystem::mkdir(const char *name, mode_t mode) { 00130 FRESULT res = f_mkdir(name); 00131 return res == 0 ? 0 : -1; 00132 } 00133 00134 } // namespace mbed
Generated on Tue Jul 12 2022 20:09:01 by
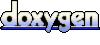