Fork of Smoothie to port to mbed non-LPC targets.
Fork of Smoothie by
ControlScreen.cpp
00001 /* 00002 This file is part of Smoothie (http://smoothieware.org/). The motion control part is heavily based on Grbl (https://github.com/simen/grbl). 00003 Smoothie is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version. 00004 Smoothie is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. 00005 You should have received a copy of the GNU General Public License along with Smoothie. If not, see <http://www.gnu.org/licenses/>. 00006 */ 00007 00008 #include "libs/Kernel.h" 00009 #include "libs/SerialMessage.h" 00010 #include "Panel.h" 00011 #include "PanelScreen.h" 00012 #include "MainMenuScreen.h" 00013 #include "ControlScreen.h" 00014 #include "libs/nuts_bolts.h" 00015 #include "libs/utils.h" 00016 #include <string> 00017 #include "modules/robot/RobotPublicAccess.h" 00018 using namespace std; 00019 00020 ControlScreen::ControlScreen() 00021 { 00022 this->control_mode = NULL_CONTROL_MODE; 00023 } 00024 00025 void ControlScreen::on_enter() 00026 { 00027 this->panel->enter_menu_mode(); 00028 this->panel->setup_menu(4); 00029 get_current_pos(this->pos); 00030 this->refresh_menu(); 00031 this->pos_changed = false; 00032 } 00033 00034 // called in on_idle() 00035 void ControlScreen::on_refresh() 00036 { 00037 if ( this->panel->menu_change() ) { 00038 this->refresh_menu(); 00039 } 00040 00041 if (this->control_mode == AXIS_CONTROL_MODE) { 00042 00043 if ( this->panel->click() ) { 00044 this->enter_menu_control(); 00045 this->refresh_menu(); 00046 00047 } else if (this->panel->control_value_change()) { 00048 this->pos[this->controlled_axis - 'X'] = this->panel->get_control_value(); 00049 this->panel->lcd->setCursor(0, 2); 00050 this->display_axis_line(this->controlled_axis); 00051 this->pos_changed = true; // make the gcode in main_loop 00052 } 00053 00054 } else { 00055 if ( this->panel->click() ) { 00056 this->clicked_menu_entry(this->panel->get_menu_current_line()); 00057 } 00058 } 00059 } 00060 00061 // queuing gcodes needs to be done from main loop 00062 void ControlScreen::on_main_loop() 00063 { 00064 // change actual axis value 00065 if (!this->pos_changed) return; 00066 this->pos_changed = false; 00067 00068 set_current_pos(this->controlled_axis, this->pos[this->controlled_axis - 'X']); 00069 } 00070 00071 void ControlScreen::display_menu_line(uint16_t line) 00072 { 00073 // in menu mode 00074 switch ( line ) { 00075 case 0: this->panel->lcd->printf("Back"); break; 00076 case 1: this->display_axis_line('X'); break; 00077 case 2: this->display_axis_line('Y'); break; 00078 case 3: this->display_axis_line('Z'); break; 00079 } 00080 } 00081 00082 void ControlScreen::display_axis_line(char axis) 00083 { 00084 this->panel->lcd->printf("Move %c %8.3f", axis, this->pos[axis - 'X']); 00085 } 00086 00087 00088 void ControlScreen::clicked_menu_entry(uint16_t line) 00089 { 00090 switch ( line ) { 00091 case 0: this->panel->enter_screen(this->parent ); break; 00092 case 1: this->enter_axis_control('X'); break; 00093 case 2: this->enter_axis_control('Y'); break; 00094 case 3: this->enter_axis_control('Z'); break; 00095 } 00096 } 00097 00098 void ControlScreen::enter_axis_control(char axis) 00099 { 00100 this->control_mode = AXIS_CONTROL_MODE; 00101 this->controlled_axis = axis; 00102 this->panel->enter_control_mode(this->jog_increment, this->jog_increment / 10); 00103 this->panel->set_control_value(this->pos[axis - 'X']); 00104 this->panel->lcd->clear(); 00105 this->panel->lcd->setCursor(0, 2); 00106 this->display_axis_line(this->controlled_axis); 00107 } 00108 00109 void ControlScreen::enter_menu_control() 00110 { 00111 this->control_mode = NULL_CONTROL_MODE; 00112 this->panel->enter_menu_mode(); 00113 } 00114 00115 void ControlScreen::get_current_pos(float *cp) 00116 { 00117 void *returned_data; 00118 00119 bool ok = THEKERNEL->public_data->get_value( robot_checksum, current_position_checksum, &returned_data ); 00120 if (ok) { 00121 float *p = static_cast<float *>(returned_data); 00122 cp[0] = p[0]; 00123 cp[1] = p[1]; 00124 cp[2] = p[2]; 00125 } 00126 } 00127 00128 void ControlScreen::set_current_pos(char axis, float p) 00129 { 00130 // change pos by issuing a G0 Xnnn 00131 char buf[32]; 00132 int n = snprintf(buf, sizeof(buf), "G0 %c%f F%d", axis, p, (int)round(panel->get_jogging_speed(axis))); 00133 string g(buf, n); 00134 send_gcode(g); 00135 }
Generated on Tue Jul 12 2022 20:09:00 by
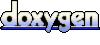