This is an example program that shows how to use timer, ticker and interrupts. It uses LPC1768 board leds and a button to show how interrupts, timers and tickers are implemented to toggle leds.
main.cpp
00001 /* Interrupt, timer and ticker example on LPC1768 00002 * 00003 * @author: Baser Kandehir 00004 * @date: July 9, 2015 00005 * @license: MIT license 00006 * 00007 * Copyright (c) 2015, Baser Kandehir, baser.kandehir@ieee.metu.edu.tr 00008 * 00009 * Permission is hereby granted, free of charge, to any person obtaining a copy 00010 * of this software and associated documentation files (the "Software"), to deal 00011 * in the Software without restriction, including without limitation the rights 00012 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00013 * copies of the Software, and to permit persons to whom the Software is 00014 * furnished to do so, subject to the following conditions: 00015 * 00016 * The above copyright notice and this permission notice shall be included in 00017 * all copies or substantial portions of the Software. 00018 * 00019 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00020 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00021 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00022 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00023 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00024 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00025 * THE SOFTWARE. 00026 * 00027 * @description of the program: 00028 * 00029 * This is an example program that shows how to use timer, ticker and interrupts. 00030 * It uses LPC1768 board leds and a button to show how interrupts, timers and tickers 00031 * are implemented to toggle leds. 00032 * A ticker is an interrupt service routine which calls a function at periodic time intervals. 00033 * There are two tickers in the program. First ticker calls the toggle_led1 function every 100 ms 00034 * and second ticker calls the toggle_led2 function every 500 ms. A button is connected to show 00035 * how external interrupts work on the mbed LPC1768. Whenever user pushes the button, it pulls 00036 * down the pin 18 to 0V and external interrupt occurs at the falling edge of p18. Whenever an external 00037 * interrupt occurs, it calls the led3_debounced function to toggle led3. Also a timer is used to debounce 00038 * the button by counting 20 ms. Debounce is required for buttons in order to prevent false readings. 00039 * 00040 * @connections: Pull up button with 10k resistor is connected to pin 18. 00041 * 00042 */ 00043 00044 #include "mbed.h" 00045 00046 Ticker toggler1; // periodic interrupt routines 00047 Ticker toggler2; 00048 Timer debounce; // define debounce timer 00049 InterruptIn button(p18); // hardware interrupt on button - pin 18 00050 00051 /* IMPORTANT NOTE: any digital input can be an interrupt except pin 19 and pin 20 */ 00052 00053 /* Interesting way of initialize all the 4 leds on the LPC1768 to an array named leds[]. */ 00054 DigitalOut leds[] = {(LED1), (LED2), (LED3), (LED4)}; 00055 00056 /* Function Prototypes */ 00057 void toggle_led1(); 00058 void toggle_led2(); 00059 void led3_debounced(); 00060 00061 int main() 00062 { 00063 /* Leds are initially OFF */ 00064 for(int i=0; i<4 ;i++) 00065 leds[i]=0; 00066 00067 /* Start debounce timer */ 00068 debounce.start(); 00069 00070 /* Attach functions to periodic interrupts */ 00071 toggler1.attach(&toggle_led1,0.1); // toggles led1 every 100 ms 00072 toggler2.attach(&toggle_led2,0.5); // toggles led2 every 500 ms 00073 00074 /* Call toggle_led3 falling edge of the button interrupt */ 00075 button.fall(&led3_debounced); 00076 while(1) 00077 { 00078 00079 } 00080 } 00081 00082 void toggle_led1() {leds[0] = !leds[0];} 00083 void toggle_led2() {leds[1] = !leds[1];} 00084 00085 // whenever user pushes the button, led3 toggles. 00086 // button is debounced by waiting at least 20 ms. 00087 void led3_debounced() 00088 { 00089 if(debounce.read_ms()>20) // only allow toggle after 20 ms 00090 { 00091 leds[2] = !leds[2]; 00092 debounce.reset(); // restart timer after toggle 00093 } 00094 }
Generated on Sat Jul 16 2022 20:10:01 by
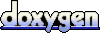