
BETZtechnik openPnP feeder driver firmware
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 // JUMPERS REQUIRED ON MOTOR DRIVER STBY PINS ! PULL TO 3V3. 00004 // Feeder message is FxxTx(L or R) F= feeder ID, T= teethRequested, (L for left or R for right) 00005 // ************************************************* 00006 // FEEDER ID !! Set 10's and 1's (for ID= 13, tens = 1 and ones = 3) USE CHAR! 00007 char tens = '0'; 00008 char ones = '1'; 00009 00010 00011 int teethRequested = 0; // how many teeth advance has been selected? 00012 int index = 0; // for parsing serial 00013 00014 00015 Timer timer; 00016 00017 InterruptIn lOpto(P1_31); 00018 InterruptIn lButton(P1_25); 00019 InterruptIn rOpto(P1_29); 00020 InterruptIn rButton(P1_26); 00021 00022 //LEDs 00023 DigitalOut lLed(P0_18); 00024 DigitalOut rLed(P1_16); 00025 00026 //Left Tape 00027 DigitalOut lTapeIn1(P1_20); 00028 DigitalOut lTapeIn2(P1_21); 00029 PwmOut lTapePWM(P1_24); 00030 AnalogIn tapePot(P0_12); // will be 0 -1024 00031 int tapePotADC; // after math will be 0-9 for PWMwrite 00032 00033 //Left motor 00034 DigitalOut lMotorIn1(P0_15); 00035 DigitalOut lMotorIn2(P0_16); 00036 PwmOut lMotorPWM(P1_24); 00037 int lMotorSet = 5; // use 1-10 (whole numbers) to set PWM, it is /10 in the function to be float (lMotorSet / 10) 00038 00039 // Right Tape 00040 DigitalOut rTapeIn1(P0_22); 00041 DigitalOut rTapeIn2(P0_21); 00042 PwmOut rTapePWM(P1_24); 00043 00044 //Right motor 00045 DigitalOut rMotorIn1(P0_23); 00046 DigitalOut rMotorIn2(P1_15); 00047 PwmOut rMotorPWM(P1_24); 00048 int rMotorSet = 5; // use 1-10 (whole numbers) to set PWM, it is /10 in the function to be float (lMotorSet / 10) 00049 00050 ////////////////////////////////////////////////////////// 00051 00052 void lFeed(){ 00053 if (teethRequested == 0){ // this is just for the button, if over serial then teethrequested has already been set to > 0 00054 teethRequested = 1; // change to how many teeth you want the button to feed 00055 } 00056 00057 //Tape 00058 lTapeIn1 = 0; // reverse 1's and 0's if rotation is backwards 00059 lTapeIn2 = 1; 00060 lTapePWM.write((tapePotADC / 10)); // from POT , max 0.9 with this code as ADC value is being divided by 103 00061 00062 //Motor 00063 lMotorIn1 = 0; 00064 lMotorIn2 = 1; 00065 lMotorPWM.write(lMotorSet / 10); 00066 } 00067 00068 void rFeed(){ 00069 if (teethRequested == 0){ // this is just for the button, if over serial then teethrequested has already been set to > 0 00070 teethRequested = 1; // change to how many teeth you want the button to feed 00071 } 00072 00073 //Tape 00074 rTapeIn1 = 0; // reverse 1's and 0's if rotation is backwards 00075 rTapeIn2 = 1; 00076 rTapePWM.write((tapePotADC / 10)); // from POT , max 0.9 with this code as ADC value is being divided by 103 00077 00078 //Motor 00079 rMotorIn1 = 0; 00080 rMotorIn2 = 1; 00081 rMotorPWM.write(lMotorSet / 10); 00082 } 00083 00084 void lStop(){ 00085 if (teethRequested == 1){ // If only one (or one more) tooth needed to be driven 00086 00087 //tape 00088 lTapeIn1 = 0; 00089 lTapeIn2 = 0; 00090 lTapePWM.write(0.0f); //PWM off 00091 00092 //motor 00093 lMotorIn1 = 0; 00094 lMotorIn2 = 0; 00095 lMotorPWM.write(0.0f); //PWM off 00096 teethRequested = 0; // set teeth requested to zero so it will ignore further opto interrupts. 00097 } 00098 00099 if (teethRequested <=2){ // If more than one tooth needs to be driven, skip this interrupt and decrement 00100 teethRequested = (teethRequested - 1); 00101 } 00102 } 00103 00104 void rStop(){ 00105 if (teethRequested == 1){ // If only one (or one more) tooth needed to be driven 00106 00107 //tape 00108 rTapeIn1 = 0; 00109 rTapeIn2 = 0; 00110 rTapePWM.write(0.0f); //PWM off 00111 00112 //motor 00113 rMotorIn1 = 0; 00114 rMotorIn2 = 0; 00115 rMotorPWM.write(0.0f); //PWM off 00116 teethRequested = 0; // set teeth requested to zero so it will ignore further opto interrupts. 00117 } 00118 00119 if (teethRequested <=2){ // If more than one tooth needs to be driven, skip this interrupt and decrement 00120 teethRequested = (teethRequested - 1); 00121 } 00122 } 00123 00124 // SERIAL 00125 00126 Serial device(P1_13, P1_14); // RS 485 00127 00128 00129 int main() { 00130 00131 lOpto.mode(PullNone); // external pull up 00132 lButton.mode(PullUp); // button pull up 00133 rOpto.mode(PullNone); 00134 00135 lOpto.fall(&lStop); 00136 lButton.fall(&lFeed); 00137 00138 uint8_t c = 0; 00139 device.printf("Hello World\n"); 00140 00141 while(1) { 00142 00143 // TODO create timer for USB debug messages 00144 00145 while (device.readable()) 00146 { 00147 c = device.getc(); 00148 if (c == 'F'){ 00149 index = 1; 00150 } 00151 00152 if (index == 1){ 00153 if (c == tens){ 00154 index = 2; 00155 } 00156 } 00157 00158 if (index == 2){ 00159 if (c == ones);{ 00160 index = 3; 00161 } 00162 } 00163 00164 if (index == 3){ 00165 if (c == '1'){ 00166 teethRequested = 1; 00167 } 00168 else if (c == '2'){ 00169 teethRequested = 2; 00170 } 00171 else if (c == '3'){ 00172 teethRequested = 3; 00173 } 00174 else if (c == '4'){ 00175 teethRequested = 4; 00176 } 00177 index = 4; 00178 } 00179 00180 if (index == 4){ 00181 if (c == 'L'){ 00182 lFeed(); 00183 index = 0; 00184 } 00185 } 00186 if (c == 'R'){ 00187 rFeed(); 00188 index = 0; 00189 } 00190 } 00191 00192 00193 tapePotADC = ((tapePot)/ 103); // covert 1024 to (1 -9) for the PWM setting. 00194 00195 00196 00197 if(lOpto){ 00198 lLed = 0;} 00199 else{ 00200 lLed = 1; 00201 } 00202 } 00203 00204 }
Generated on Mon Aug 8 2022 08:50:25 by
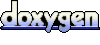