iothub_ll_telemetry_sample
Embed:
(wiki syntax)
Show/hide line numbers
iothub_ll_telemetry_sample.c
00001 // Copyright (c) Microsoft. All rights reserved. 00002 // Licensed under the MIT license. See LICENSE file in the project root for full license information. 00003 00004 // CAVEAT: This sample is to demonstrate azure IoT client concepts only and is not a guide design principles or style 00005 // Checking of return codes and error values shall be omitted for brevity. Please practice sound engineering practices 00006 // when writing production code. 00007 00008 #include <stdio.h> 00009 #include <stdlib.h> 00010 00011 #include "iothub.h" 00012 #include "iothub_device_client_ll.h" 00013 #include "iothub_client_options.h" 00014 #include "iothub_message.h" 00015 #include "azure_c_shared_utility/threadapi.h" 00016 #include "azure_c_shared_utility/crt_abstractions.h" 00017 #include "azure_c_shared_utility/shared_util_options.h" 00018 00019 #ifdef SET_TRUSTED_CERT_IN_SAMPLES 00020 #include "certs.h" 00021 #endif // SET_TRUSTED_CERT_IN_SAMPLES 00022 00023 /* This sample uses the _LL APIs of iothub_client for example purposes. 00024 Simply changing the using the convenience layer (functions not having _LL) 00025 and removing calls to _DoWork will yield the same results. */ 00026 00027 // The protocol you wish to use should be uncommented 00028 // 00029 #define SAMPLE_MQTT 00030 //#define SAMPLE_MQTT_OVER_WEBSOCKETS 00031 //#define SAMPLE_AMQP 00032 //#define SAMPLE_AMQP_OVER_WEBSOCKETS 00033 //#define SAMPLE_HTTP 00034 00035 #ifdef SAMPLE_MQTT 00036 #include "iothubtransportmqtt.h" 00037 #endif // SAMPLE_MQTT 00038 #ifdef SAMPLE_MQTT_OVER_WEBSOCKETS 00039 #include "iothubtransportmqtt_websockets.h" 00040 #endif // SAMPLE_MQTT_OVER_WEBSOCKETS 00041 #ifdef SAMPLE_AMQP 00042 #include "iothubtransportamqp.h" 00043 #endif // SAMPLE_AMQP 00044 #ifdef SAMPLE_AMQP_OVER_WEBSOCKETS 00045 #include "iothubtransportamqp_websockets.h" 00046 #endif // SAMPLE_AMQP_OVER_WEBSOCKETS 00047 #ifdef SAMPLE_HTTP 00048 #include "iothubtransporthttp.h" 00049 #endif // SAMPLE_HTTP 00050 00051 #ifdef SET_TRUSTED_CERT_IN_SAMPLES 00052 #include "certs.h" 00053 #endif // SET_TRUSTED_CERT_IN_SAMPLES 00054 00055 /* Paste in the your iothub connection string */ 00056 static const char* connectionString = "[device connection string]"; 00057 #define MESSAGE_COUNT 5 00058 static bool g_continueRunning = true; 00059 static size_t g_message_count_send_confirmations = 0; 00060 00061 static void send_confirm_callback(IOTHUB_CLIENT_CONFIRMATION_RESULT result, void* userContextCallback) 00062 { 00063 (void)userContextCallback; 00064 // When a message is sent this callback will get envoked 00065 g_message_count_send_confirmations++; 00066 (void)printf("Confirmation callback received for message %zu with result %s\r\n", g_message_count_send_confirmations, ENUM_TO_STRING(IOTHUB_CLIENT_CONFIRMATION_RESULT, result)); 00067 } 00068 00069 static void connection_status_callback(IOTHUB_CLIENT_CONNECTION_STATUS result, IOTHUB_CLIENT_CONNECTION_STATUS_REASON reason, void* user_context) 00070 { 00071 (void)reason; 00072 (void)user_context; 00073 // This sample DOES NOT take into consideration network outages. 00074 if (result == IOTHUB_CLIENT_CONNECTION_AUTHENTICATED) 00075 { 00076 (void)printf("The device client is connected to iothub\r\n"); 00077 } 00078 else 00079 { 00080 (void)printf("The device client has been disconnected\r\n"); 00081 } 00082 } 00083 00084 int main(void) 00085 { 00086 IOTHUB_CLIENT_TRANSPORT_PROVIDER protocol; 00087 IOTHUB_MESSAGE_HANDLE message_handle; 00088 size_t messages_sent = 0; 00089 const char* telemetry_msg = "test_message"; 00090 00091 // Select the Protocol to use with the connection 00092 #ifdef SAMPLE_MQTT 00093 protocol = MQTT_Protocol; 00094 #endif // SAMPLE_MQTT 00095 #ifdef SAMPLE_MQTT_OVER_WEBSOCKETS 00096 protocol = MQTT_WebSocket_Protocol; 00097 #endif // SAMPLE_MQTT_OVER_WEBSOCKETS 00098 #ifdef SAMPLE_AMQP 00099 protocol = AMQP_Protocol; 00100 #endif // SAMPLE_AMQP 00101 #ifdef SAMPLE_AMQP_OVER_WEBSOCKETS 00102 protocol = AMQP_Protocol_over_WebSocketsTls; 00103 #endif // SAMPLE_AMQP_OVER_WEBSOCKETS 00104 #ifdef SAMPLE_HTTP 00105 protocol = HTTP_Protocol; 00106 #endif // SAMPLE_HTTP 00107 00108 // Used to initialize IoTHub SDK subsystem 00109 (void)IoTHub_Init(); 00110 00111 IOTHUB_DEVICE_CLIENT_LL_HANDLE device_ll_handle; 00112 00113 (void)printf("Creating IoTHub Device handle\r\n"); 00114 // Create the iothub handle here 00115 device_ll_handle = IoTHubDeviceClient_LL_CreateFromConnectionString(connectionString, protocol); 00116 if (device_ll_handle == NULL) 00117 { 00118 (void)printf("Failure createing Iothub device. Hint: Check you connection string.\r\n"); 00119 } 00120 else 00121 { 00122 // Set any option that are neccessary. 00123 // For available options please see the iothub_sdk_options.md documentation 00124 00125 bool traceOn = true; 00126 IoTHubDeviceClient_LL_SetOption(device_ll_handle, OPTION_LOG_TRACE, &traceOn); 00127 00128 #ifdef SET_TRUSTED_CERT_IN_SAMPLES 00129 // Setting the Trusted Certificate. This is only necessary on system with without 00130 // built in certificate stores. 00131 IoTHubDeviceClient_LL_SetOption(device_ll_handle, OPTION_TRUSTED_CERT, certificates); 00132 #endif // SET_TRUSTED_CERT_IN_SAMPLES 00133 00134 #if defined SAMPLE_MQTT || defined SAMPLE_MQTT_WS 00135 //Setting the auto URL Encoder (recommended for MQTT). Please use this option unless 00136 //you are URL Encoding inputs yourself. 00137 //ONLY valid for use with MQTT 00138 //bool urlEncodeOn = true; 00139 //IoTHubDeviceClient_LL_SetOption(iothub_ll_handle, OPTION_AUTO_URL_ENCODE_DECODE, &urlEncodeOn); 00140 #endif 00141 00142 // Setting connection status callback to get indication of connection to iothub 00143 (void)IoTHubDeviceClient_LL_SetConnectionStatusCallback(device_ll_handle, connection_status_callback, NULL); 00144 00145 do 00146 { 00147 if (messages_sent < MESSAGE_COUNT) 00148 { 00149 // Construct the iothub message from a string or a byte array 00150 message_handle = IoTHubMessage_CreateFromString(telemetry_msg); 00151 //message_handle = IoTHubMessage_CreateFromByteArray((const unsigned char*)msgText, strlen(msgText))); 00152 00153 // Set Message property 00154 /*(void)IoTHubMessage_SetMessageId(message_handle, "MSG_ID"); 00155 (void)IoTHubMessage_SetCorrelationId(message_handle, "CORE_ID"); 00156 (void)IoTHubMessage_SetContentTypeSystemProperty(message_handle, "application%2fjson"); 00157 (void)IoTHubMessage_SetContentEncodingSystemProperty(message_handle, "utf-8");*/ 00158 00159 // Add custom properties to message 00160 (void)IoTHubMessage_SetProperty(message_handle, "property_key", "property_value"); 00161 00162 (void)printf("Sending message %d to IoTHub\r\n", (int)(messages_sent + 1)); 00163 IoTHubDeviceClient_LL_SendEventAsync(device_ll_handle, message_handle, send_confirm_callback, NULL); 00164 00165 // The message is copied to the sdk so the we can destroy it 00166 IoTHubMessage_Destroy(message_handle); 00167 00168 messages_sent++; 00169 } 00170 else if (g_message_count_send_confirmations >= MESSAGE_COUNT) 00171 { 00172 // After all messages are all received stop running 00173 g_continueRunning = false; 00174 } 00175 00176 IoTHubDeviceClient_LL_DoWork(device_ll_handle); 00177 ThreadAPI_Sleep(1); 00178 00179 } while (g_continueRunning); 00180 00181 // Clean up the iothub sdk handle 00182 IoTHubDeviceClient_LL_Destroy(device_ll_handle); 00183 } 00184 // Free all the sdk subsystem 00185 IoTHub_Deinit(); 00186 00187 printf("Press any key to continue"); 00188 getchar(); 00189 00190 return 0; 00191 }
Generated on Sun Jul 17 2022 07:32:11 by
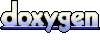