
Init Project for Ping
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* WIZnet IoT Shield Cat.M1 Sample code for Arm MBED 00002 * Copyright (c) 2019 WIZnet Co., Ltd. 00003 * SPDX-License-Identifier: Apache-2.0 00004 */ 00005 00006 /* 00007 * Licensed under the Apache License, Version 2.0 (the "License"); 00008 * you may not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, 00015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * 00017 * See the License for the specific language governing permissions and 00018 * limitations under the License. 00019 * 00020 */ 00021 00022 #include "mbed.h" 00023 00024 #include <string> 00025 00026 #define RET_OK 1 00027 #define RET_NOK -1 00028 #define DEBUG_ENABLE 1 00029 #define DEBUG_DISABLE 0 00030 #define ON 1 00031 #define OFF 0 00032 00033 #define MAX_BUF_SIZE 1024 00034 00035 #define WM01_APN_PROTOCOL_IPv4 1 00036 #define WM01_APN_PROTOCOL_IPv6 2 00037 #define WM01_DEFAULT_TIMEOUT 1000 00038 #define WM01_CONNECT_TIMEOUT 15000 00039 #define WM01_SEND_TIMEOUT 500 00040 #define WM01_RECV_TIMEOUT 500 00041 #define WM01_BOOTING_TIME 15000 00042 00043 #define WM01_APN_PROTOCOL WM01_APN_PROTOCOL_IPv6 00044 #define WM01_DEFAULT_BAUD_RATE 115200 00045 #define WM01_PARSER_DELIMITER "\r\n" 00046 00047 #define CATM1_APN_SKT "lte-internet.sktelecom.com" 00048 00049 #define CATM1_DEVICE_NAME_WM01 "WM01" 00050 #define DEVNAME CATM1_DEVICE_NAME_WM01 00051 00052 #define devlog(f_, ...) if(CATM1_DEVICE_DEBUG == DEBUG_ENABLE) { pc.printf("\r\n[%s] ", DEVNAME); pc.printf((f_), ##__VA_ARGS__); } 00053 #define myprintf(f_, ...) {pc.printf("\r\n[MAIN] "); pc.printf((f_), ##__VA_ARGS__);} 00054 00055 /* Pin configuraiton */ 00056 // Cat.M1 00057 #define MBED_CONF_IOTSHIELD_CATM1_TX D8 00058 #define MBED_CONF_IOTSHIELD_CATM1_RX D2 00059 #define MBED_CONF_IOTSHIELD_CATM1_RESET D7 00060 #define MBED_CONF_IOTSHIELD_CATM1_PWRKEY D9 00061 00062 // Sensors 00063 #define MBED_CONF_IOTSHIELD_SENSOR_CDS A0 00064 #define MBED_CONF_IOTSHIELD_SENSOR_TEMP A1 00065 00066 /* Debug message settings */ 00067 #define WM01_PARSER_DEBUG DEBUG_DISABLE 00068 #define CATM1_DEVICE_DEBUG DEBUG_ENABLE 00069 00070 // Functions: Print information 00071 void printInfo(void); 00072 00073 // Functions: Module Status 00074 void waitCatM1Ready(void); 00075 int8_t setEchoStatus_WM01(bool onoff); 00076 int8_t getUsimStatus_WM01(void); 00077 int8_t getNetworkStatus_WM01(void); 00078 00079 // Functions: PDP context 00080 int8_t setContextActivate_WM01(void); // Activate a PDP Context 00081 int8_t setContextDeactivate_WM01(void); // Deactivate a PDP Context 00082 00083 // Functions: Ping test 00084 void printPingToHost_WM01(char *host, int pingnum); 00085 00086 Serial pc(USBTX, USBRX); // USB debug 00087 00088 UARTSerial *_serial; // Cat.M1 module 00089 ATCmdParser *_parser; 00090 00091 DigitalOut _RESET_WM01(MBED_CONF_IOTSHIELD_CATM1_RESET); 00092 DigitalOut _PWRKEY_WM01(MBED_CONF_IOTSHIELD_CATM1_PWRKEY); 00093 00094 void serialPcInit(void) 00095 { 00096 pc.baud(115200); 00097 pc.format(8, Serial::None, 1); 00098 } 00099 00100 void serialDeviceInit(PinName tx, PinName rx, int baudrate) 00101 { 00102 _serial = new UARTSerial(tx, rx, baudrate); 00103 } 00104 00105 void serialAtParserInit(const char *delimiter, bool debug_en) 00106 { 00107 _parser = new ATCmdParser(_serial); 00108 _parser->debug_on(debug_en); 00109 _parser->set_delimiter(delimiter); 00110 _parser->set_timeout(WM01_DEFAULT_TIMEOUT); 00111 } 00112 00113 void catm1DeviceInit(void) 00114 { 00115 serialDeviceInit( MBED_CONF_IOTSHIELD_CATM1_TX, 00116 MBED_CONF_IOTSHIELD_CATM1_RX, 00117 WM01_DEFAULT_BAUD_RATE); 00118 00119 serialAtParserInit( WM01_PARSER_DELIMITER, 00120 WM01_PARSER_DEBUG); 00121 } 00122 00123 void catm1DeviceReset_WM01(void) 00124 { 00125 _RESET_WM01 = 1; 00126 _PWRKEY_WM01 = 1; 00127 wait_ms(300); 00128 00129 _RESET_WM01 = 0; 00130 _PWRKEY_WM01 = 0; 00131 wait_ms(400); 00132 00133 _RESET_WM01 = 1; 00134 wait_ms(1000); 00135 } 00136 00137 // ---------------------------------------------------------------- 00138 // Main routine 00139 // ---------------------------------------------------------------- 00140 00141 int main() 00142 { 00143 char ping_dest_1st[] = "8.8.8.8"; 00144 char ping_dest_2nd[] = "www.google.com"; 00145 00146 serialPcInit(); 00147 catm1DeviceInit(); 00148 00149 myprintf("Waiting for Cat.M1 Module Ready...\r\n"); 00150 00151 catm1DeviceReset_WM01(); 00152 00153 waitCatM1Ready(); 00154 00155 wait_ms(5000); 00156 00157 myprintf("System Init Complete\r\n"); 00158 00159 printInfo(); 00160 00161 setEchoStatus_WM01(OFF); 00162 00163 getUsimStatus_WM01(); 00164 00165 getNetworkStatus_WM01(); 00166 00167 setContextActivate_WM01(); 00168 00169 myprintf("[Ping] Host : %s\r\n", ping_dest_1st); 00170 printPingToHost_WM01(ping_dest_1st, 4); 00171 00172 wait_ms(2000); 00173 00174 myprintf("[Ping] Host : %s\r\n", ping_dest_2nd); 00175 printPingToHost_WM01(ping_dest_2nd, 4); 00176 00177 setContextDeactivate_WM01(); 00178 } 00179 00180 // ---------------------------------------------------------------- 00181 // Functions: Print information 00182 // ---------------------------------------------------------------- 00183 00184 void printInfo(void) 00185 { 00186 myprintf("WIZnet IoT Shield for Arm MBED"); 00187 myprintf("LTE Cat.M1 Version"); 00188 myprintf("================================================="); 00189 myprintf(">> Target Board: WIoT-WM01 (Woorinet WM-N400MSE)"); 00190 myprintf(">> Sample Code: Ping Test"); 00191 myprintf("=================================================\r\n"); 00192 } 00193 00194 // ---------------------------------------------------------------- 00195 // Functions: Cat.M1 Status 00196 // ---------------------------------------------------------------- 00197 00198 void waitCatM1Ready(void) 00199 { 00200 while(1) 00201 { 00202 if(_parser->send("AT") && _parser->recv("OK")) 00203 { 00204 myprintf("WM01 is Available\r\n"); 00205 00206 return; 00207 } 00208 } 00209 } 00210 00211 int8_t setEchoStatus_WM01(bool onoff) 00212 { 00213 int8_t ret = RET_NOK; 00214 char _buf[10]; 00215 00216 sprintf((char *)_buf, "ATE%d", onoff); 00217 00218 if(_parser->send(_buf) && _parser->recv("OK")) 00219 { 00220 devlog("Turn Echo %s : success\r\n", onoff?"ON":"OFF"); 00221 00222 ret = RET_OK; 00223 } 00224 else 00225 { 00226 devlog("Turn Echo %s : failed\r\n", onoff?"ON":"OFF"); 00227 } 00228 00229 return ret; 00230 } 00231 00232 int8_t getUsimStatus_WM01(void) 00233 { 00234 int8_t ret = RET_NOK; 00235 00236 if(_parser->send("AT$$STAT?") && _parser->recv("$$STAT:READY") && _parser->recv("OK")) 00237 { 00238 devlog("USIM Status : READY\r\n"); 00239 00240 ret = RET_OK; 00241 } 00242 else 00243 { 00244 devlog("Retrieving USIM Status failed\r\n"); 00245 } 00246 00247 return ret; 00248 } 00249 00250 int8_t getNetworkStatus_WM01(void) 00251 { 00252 int8_t ret = RET_NOK; 00253 int val, stat; 00254 00255 if(_parser->send("AT+CEREG?") && _parser->recv("+CEREG: %d,%d", &val, &stat) && _parser->recv("OK")) 00256 { 00257 if((val == 0) && (stat == 1)) 00258 { 00259 devlog("Network Status : attached\r\n"); 00260 00261 ret = RET_OK; 00262 } 00263 else 00264 { 00265 devlog("Network Status : %d, %d\r\n", val, stat); 00266 } 00267 } 00268 else 00269 { 00270 devlog("Network Status : Error\r\n"); 00271 } 00272 00273 return ret; 00274 } 00275 00276 // ---------------------------------------------------------------- 00277 // Functions: Cat.M1 PDP context activate / deactivate 00278 // ---------------------------------------------------------------- 00279 00280 int8_t setContextActivate_WM01(void) // Activate a PDP Context 00281 { 00282 int8_t ret = RET_NOK; 00283 00284 _parser->send("AT*RNDISDATA=1"); 00285 00286 if(_parser->recv("OK")) 00287 { 00288 devlog("Activate a PDP Context\r\n"); 00289 00290 ret = RET_OK; 00291 } 00292 else 00293 { 00294 devlog("PDP Context Activation failed\r\n"); 00295 } 00296 00297 return ret; 00298 } 00299 00300 int8_t setContextDeactivate_WM01(void) // Deactivate a PDP Context 00301 { 00302 int8_t ret = RET_NOK; 00303 00304 _parser->send("AT*RNDISDATA=0"); 00305 00306 if(_parser->recv("OK")) 00307 { 00308 devlog("Deactivate a PDP Context\r\n"); 00309 00310 ret = RET_OK; 00311 } 00312 else 00313 { 00314 devlog("PDP Context Deactivation failed\r\n"); 00315 } 00316 00317 return ret; 00318 } 00319 00320 // ---------------------------------------------------------------- 00321 // Functions: Cat.M1 Ping test 00322 // ---------------------------------------------------------------- 00323 00324 void printPingToHost_WM01(char *host, int pingnum) 00325 { 00326 char resp_str[1024] = {0, }; 00327 00328 if((pingnum < 1) || (pingnum > 10)) 00329 { 00330 devlog("The maximum number of sending Ping request range is 1-10, and the default value is 4\r\n"); 00331 00332 return; 00333 } 00334 00335 _parser->set_timeout(1000 * pingnum); 00336 00337 if(_parser->send("AT*PING=%s,%d", host, pingnum) && _parser->recv("OK")) 00338 { 00339 _parser->read(resp_str, sizeof(resp_str)); 00340 00341 devlog("%s", resp_str); 00342 } 00343 00344 _parser->set_timeout(WM01_DEFAULT_TIMEOUT); 00345 _parser->flush(); 00346 }
Generated on Wed Jul 27 2022 21:12:59 by
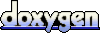