
This code will read the data from the X,Y and Z axis of the MLX90393 Magnetometer and display it on a serial monitor. Remember to check the solder links on the I2C lines on your Nucleo as this can interfere on certain boards.
main.cpp
00001 #include "mbed.h" 00002 00003 int addr = 0x0C <<1; // 8bit I2C address 00004 00005 I2C i2c(PB_9 , PB_8); //sda, scl 00006 00007 Serial pc(PA_2, PA_3); //Tx/Rx 00008 00009 int main() 00010 { 00011 char config [4]; 00012 char data[7] = {0}; 00013 00014 config[0] = 0x60; 00015 config[1] = 0x00; 00016 config[2] = 0x5C; 00017 config[3] = 0x00; 00018 00019 i2c.write(addr, config, 4, false); 00020 00021 i2c.read(addr, data, 1); 00022 00023 config[0] = 0x60; 00024 config[1] = 0x02; 00025 config[2] = 0xB4; 00026 config[3] = 0x02; 00027 00028 i2c.write(addr, config, 4, false); 00029 00030 i2c.read(addr, data, 1); 00031 00032 wait(0.25); 00033 00034 while (1) { 00035 00036 config[0] = 0x3E; // Single measurement mode, ZYX enabled 00037 00038 i2c.write(addr, config, 1, false); 00039 i2c.read(addr, data, 1); 00040 00041 wait(0.1); 00042 00043 config[0] = 0x4E; 00044 00045 i2c.write(addr, config, 1, false); // Read command, followed by ZYX bits set 00046 i2c.read(addr, data, 7); 00047 00048 int xMag = ((data[1] * 256) + data[2]); 00049 int yMag = ((data[3] * 256) + data[4]); 00050 int zMag = ((data[5] * 256) + data[6]); 00051 00052 printf("X Axis = %d \n", xMag); 00053 printf("Y Axis = %d \n", yMag); 00054 printf("Z Axis = %d \n", zMag); 00055 00056 wait(5); 00057 } 00058 }
Generated on Sat Jul 16 2022 23:33:07 by
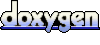