Ambient library. It provides "set" function to set data to a packet and "send" function to send the packet to the Ambient server. It also provides "bulk_send" function to send multiple data. (Japanese: IoT用のクラウドサービス「Ambient」のデーター送信ライブラリーです。Ambientはマイコンから送られたセンサーデーターを受信し、蓄積し、可視化(グラフ化)します。http://ambidata.io)
Dependents: AmbientExampleSITB AmbientHeartRateMonitor AmbientHeartBeat AmbientExampleSITB_ws ... more
Ambient.h
00001 #ifndef Ambient_h 00002 #define Ambient_h 00003 00004 #include "mbed.h" 00005 #include "TCPSocketConnection.h" 00006 00007 #define AMBIENT_WRITEKEY_SIZE 18 00008 #define AMBIENT_MAX_RETRY 5 00009 #define AMBIENT_DATA_SIZE 24 00010 #define AMBIENT_NUM_PARAMS 11 00011 #define AMBIENT_TIMEOUT 3000 // milliseconds 00012 00013 /** Ambient class 00014 * to send data to Ambient service. 00015 * 00016 * Exsample: 00017 * @code 00018 * #include "mbed.h" 00019 * #include "EthernetInterface.h" 00020 * #include "Ambient.h" 00021 * #include "HDC1000.h" 00022 * 00023 * unsigned int channelId = 100; 00024 * const char* writeKey = "ライトキー"; 00025 * Ambient ambient; 00026 * 00027 * HDC1000 hdc1000(p9,p10); 00028 * 00029 * int main() { 00030 * printf("start\r\n"); 00031 * 00032 * EthernetInterface eth; 00033 * eth.init(); //Use DHCP 00034 * eth.connect(); 00035 * 00036 * TCPSocketConnection socket; 00037 * ambient.init(channelId, writeKey, &socket); 00038 * 00039 * printf("Ambient send to ch: %d\r\n", channelId); 00040 * 00041 * while (true) { 00042 * float temp, humid; 00043 * char tempbuf[12], humidbuf[12]; 00044 * 00045 * hdc1000.get(); 00046 * temp = hdc1000.temperature(); 00047 * humid = hdc1000.humidity(); 00048 * 00049 * sprintf(tempbuf, "%2.1f", temp); 00050 * ambient.set(1, tempbuf); 00051 * sprintf(humidbuf, "%2.0f", humid); 00052 * ambient.set(2, humidbuf); 00053 * printf("Temp: %s C, Humid: %s %%\r\n", tempbuf, humidbuf); 00054 * 00055 * ambient.send(); 00056 * 00057 * wait(30.0); 00058 * } 00059 * } 00060 * @endcode 00061 */ 00062 class Ambient 00063 { 00064 public: 00065 /** Create Ambient instance 00066 */ 00067 Ambient(void); 00068 00069 /** Initialize the instance 00070 * @param channelId Initialize the Ambient instance with channelId. 00071 * @param writeKey and writeKey 00072 * @param s and pointer to socket 00073 * @returns 00074 * true on success, 00075 * false on failure 00076 */ 00077 bool init(unsigned int channelId, const char * writeKey, TCPSocketConnection * s, int dev = 0); 00078 00079 /** Set data on field-th field of payload. 00080 * @param field index of payload (1 to 8) 00081 * @param data data 00082 * @returns 00083 * true on success, 00084 * false on failure 00085 */ 00086 bool set(int field, char * data); 00087 00088 /** Set data on field-th field of payload. 00089 * @param field index of payload (1 to 8) 00090 * @param data data 00091 * @returns 00092 * true on success, 00093 * false on failure 00094 */ 00095 bool set(int field, int data); 00096 00097 /** Set data on field-th field of payload. 00098 * @param field index of payload (1 to 8) 00099 * @param data data 00100 * @returns 00101 * true on success, 00102 * false on failure 00103 */ 00104 bool set(int field, double data); 00105 00106 /** Clear data on field-th field of payload. 00107 * @param field index of payload (1 to 8) 00108 * @returns 00109 * true on success, 00110 * false on failure 00111 */ 00112 bool clear(int field); 00113 00114 /** Send data to Ambient 00115 * @returns 00116 * true on success, 00117 * false on failure 00118 */ 00119 bool send(void); 00120 00121 /** Send bulk data to Ambient 00122 * @param buf pointer to the data buffer 00123 * @returns 00124 * the number of written bytes on success (>=0) 00125 * -1 on failure 00126 */ 00127 int bulk_send(char * buf); 00128 00129 /* Delete data stored in this channel 00130 * @param userKey userKey 00131 * @returns 00132 * true on success, 00133 * false on failure 00134 */ 00135 bool delete_data(const char * userKey); 00136 00137 private: 00138 00139 TCPSocketConnection * s; 00140 unsigned int channelId; 00141 char writeKey[AMBIENT_WRITEKEY_SIZE]; 00142 int dev; 00143 char host[18]; 00144 int port; 00145 00146 struct { 00147 int set; 00148 char item[AMBIENT_DATA_SIZE]; 00149 } data[AMBIENT_NUM_PARAMS]; 00150 }; 00151 00152 #endif // Ambient_h
Generated on Sat Jul 16 2022 03:41:26 by
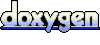