WConstants
Dependents: ZZ_SSL_Main_L476 Sample_program_Font72
WConstants.h
00001 /* $Id: WConstants.h 1156 2011-06-07 04:01:16Z bhagman $ 00002 || 00003 || @author Hernando Barragan <b@wiring.org.co> 00004 || @url http://wiring.org.co/ 00005 || @contribution Brett Hagman <bhagman@wiring.org.co> 00006 || @contribution Alexander Brevig <abrevig@wiring.org.co> 00007 || 00008 || @description 00009 || | Main constant and macro definitions for Wiring. 00010 || | 00011 || | Wiring Common API 00012 || # 00013 || 00014 || @license Please see cores/Common/License.txt. 00015 || 00016 */ 00017 00018 #ifndef WCONSTANTS_H 00019 #define WCONSTANTS_H 00020 00021 #include <stdint.h> 00022 00023 // Wiring API version for libraries 00024 // this is passed in at compile-time 00025 #ifndef WIRING 00026 #define WIRING 101 00027 #endif 00028 00029 // passed in at compile-time 00030 #ifndef F_CPU 00031 //#define F_CPU 16000000L 00032 //#warning "F_CPU was not defined. Default to 16 MHz." 00033 #endif 00034 00035 /************************************************************* 00036 * Constants 00037 *************************************************************/ 00038 00039 #define LOW 0x0 00040 #define HIGH 0x1 00041 //#define HIGH 0xFF 00042 00043 #define INPUT 0x0 00044 #define OUTPUT 0x1 00045 //#define OUTPUT 0xFF 00046 00047 #define CHANGE 1 00048 #define FALLING 2 00049 #define RISING 3 00050 00051 #define LSBFIRST 0x0 00052 #define MSBFIRST 0x1 00053 00054 #define true 0x1 00055 #define false 0x0 00056 #define TRUE 0x1 00057 #define FALSE 0x0 00058 #define null NULL 00059 00060 #define DEC 10 00061 #define HEX 16 00062 #define OCT 8 00063 #define BIN 2 00064 #define BYTE 0 00065 00066 #define PI (3.1415926535897932384626433832795) 00067 #define TWO_PI (6.283185307179586476925286766559) 00068 #define HALF_PI (1.5707963267948966192313216916398) 00069 #define EPSILON (0.0001) 00070 #define DEG_TO_RAD (0.017453292519943295769236907684886) 00071 #define RAD_TO_DEG (57.295779513082320876798154814105) 00072 00073 00074 /************************************************************* 00075 * Digital Constants 00076 *************************************************************/ 00077 00078 #define PORT0 0 00079 #define PORT1 1 00080 #define PORT2 2 00081 #define PORT3 3 00082 #define PORT4 4 00083 #define PORT5 5 00084 #define PORT6 6 00085 #define PORT7 7 00086 #define PORT8 8 00087 #define PORT9 9 00088 00089 00090 /************************************************************* 00091 * Useful macros 00092 *************************************************************/ 00093 00094 /*#define int(x) ((int)(x)) 00095 #define char(x) ((char)(x)) 00096 #define long(x) ((long)(x)) 00097 #define byte(x) ((uint8_t)(x)) 00098 #define float(x) ((float)(x)) 00099 #define boolean(x) ((uint8_t)((x)==0?false:true)) 00100 */ 00101 00102 #define word(...) makeWord(__VA_ARGS__) 00103 00104 #define sq(x) ((x)*(x)) 00105 //#define abs(x) ((x)>0?(x):-(x)) 00106 #define min(a,b) ((a)<(b)?(a):(b)) 00107 #define max(a,b) ((a)>(b)?(a):(b)) 00108 //#define round(x) ((x)>=0?(long)((x)+0.5):(long)((x)-0.5)) 00109 #define radians(deg) ((deg)*DEG_TO_RAD) 00110 #define degrees(rad) ((rad)*RAD_TO_DEG) 00111 #define constrain(amt,low,high) ((amt)<(low)?(low):((amt)>(high)?(high):(amt))) 00112 00113 #define bit(x) (1UL<<(x)) 00114 #define setBits(x, y) ((x)|=(y)) 00115 #define clearBits(x, y) ((x)&=(~(y))) 00116 //#define setBit(x, y) setBits((x), (bit((y)))) 00117 //#define clearBit(x, y) clearBits((x), (bit((y)))) 00118 00119 #define bitsSet(x,y) (((x) & (y)) == (y)) 00120 #define bitsClear(x,y) (((x) & (y)) == 0) 00121 00122 #define bitRead(value, bit) (((value) >> (bit)) & 0x01) 00123 #define bitSet(value, bit) ((value) |= (1UL << (bit))) 00124 #define bitClear(value, bit) ((value) &= ~(1UL << (bit))) 00125 #define bitWrite(value, bit, bitvalue) (bitvalue ? bitSet(value, bit) : bitClear(value, bit)) 00126 00127 #define lowByte(x) ((uint8_t) ((x) & 0x00ff)) 00128 #define highByte(x) ((uint8_t) ((x)>>8)) 00129 00130 00131 #define clockCyclesPerMicrosecond() (F_CPU / 1000000L) 00132 #define clockCyclesToMicroseconds(a) ((a) / clockCyclesPerMicrosecond()) 00133 #define microsecondsToClockCycles(a) ((a) * clockCyclesPerMicrosecond()) 00134 00135 00136 00137 /************************************************************* 00138 * Typedefs 00139 *************************************************************/ 00140 00141 typedef unsigned int word; 00142 typedef uint8_t byte; 00143 typedef uint8_t boolean; 00144 typedef void (*voidFuncPtr)(void); 00145 00146 #endif 00147 // WCONSTANTS_H
Generated on Mon Aug 8 2022 17:24:50 by
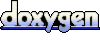