Fork of the Adafruit ST7735R library targeted to the 1.44" TFT with custom high speed monochrome and color drawing routines. Note that this library includes modifications to use a shared SPI module to simplify projects that use the SPI for several peripherals. Read the WIKI to see how to get this library working in your own project.
Fork of Adafruit_ST7735 by
Adafruit_ST7735.h
00001 /*************************************************** 00002 This is a library for the Adafruit 1.8" SPI display. 00003 This library works with the Adafruit 1.8" TFT Breakout w/SD card 00004 ----> http://www.adafruit.com/products/358 00005 as well as Adafruit raw 1.8" TFT display 00006 ----> http://www.adafruit.com/products/618 00007 00008 Check out the links above for our tutorials and wiring diagrams 00009 These displays use SPI to communicate, 4 or 5 pins are required to 00010 interface (RST is optional) 00011 Adafruit invests time and resources providing this open source code, 00012 please support Adafruit and open-source hardware by purchasing 00013 products from Adafruit! 00014 00015 Written by Limor Fried/Ladyada for Adafruit Industries. 00016 MIT license, all text above must be included in any redistribution 00017 ****************************************************/ 00018 00019 #pragma once 00020 00021 #include "mbed.h" 00022 #include "Adafruit_GFX.h" 00023 #include "N3310LCDDefs.h" 00024 #include "board.h" 00025 #define boolean bool 00026 00027 // some flags for initR() :( 00028 #define INITR_GREENTAB 0x0 00029 #define INITR_REDTAB 0x1 00030 00031 #define INITR_GREENTAB 0x0 00032 #define INITR_REDTAB 0x1 00033 #define INITR_BLACKTAB 0x2 00034 00035 #define INITR_18GREENTAB INITR_GREENTAB 00036 #define INITR_18REDTAB INITR_REDTAB 00037 #define INITR_18BLACKTAB INITR_BLACKTAB 00038 #define INITR_144GREENTAB 0x1 00039 00040 #define ST7735_TFTWIDTH 128 00041 #define ST7735_TFTHEIGHT 128 00042 00043 #define ST7735_NOP 0x00 00044 #define ST7735_SWRESET 0x01 00045 #define ST7735_RDDID 0x04 00046 #define ST7735_RDDST 0x09 00047 00048 #define ST7735_SLPIN 0x10 00049 #define ST7735_SLPOUT 0x11 00050 #define ST7735_PTLON 0x12 00051 #define ST7735_NORON 0x13 00052 00053 #define ST7735_INVOFF 0x20 00054 #define ST7735_INVON 0x21 00055 #define ST7735_DISPOFF 0x28 00056 #define ST7735_DISPON 0x29 00057 #define ST7735_CASET 0x2A 00058 #define ST7735_RASET 0x2B 00059 #define ST7735_RAMWR 0x2C 00060 #define ST7735_RAMRD 0x2E 00061 00062 #define ST7735_PTLAR 0x30 00063 #define ST7735_COLMOD 0x3A 00064 #define ST7735_MADCTL 0x36 00065 00066 #define ST7735_FRMCTR1 0xB1 00067 #define ST7735_FRMCTR2 0xB2 00068 #define ST7735_FRMCTR3 0xB3 00069 #define ST7735_INVCTR 0xB4 00070 #define ST7735_DISSET5 0xB6 00071 00072 #define ST7735_PWCTR1 0xC0 00073 #define ST7735_PWCTR2 0xC1 00074 #define ST7735_PWCTR3 0xC2 00075 #define ST7735_PWCTR4 0xC3 00076 #define ST7735_PWCTR5 0xC4 00077 #define ST7735_VMCTR1 0xC5 00078 00079 #define ST7735_RDID1 0xDA 00080 #define ST7735_RDID2 0xDB 00081 #define ST7735_RDID3 0xDC 00082 #define ST7735_RDID4 0xDD 00083 00084 #define ST7735_PWCTR6 0xFC 00085 00086 #define ST7735_GMCTRP1 0xE0 00087 #define ST7735_GMCTRN1 0xE1 00088 00089 // Color definitions 00090 #define ST7735_BLACK 0x0000 00091 #define ST7735_BLUE 0x001F 00092 #define ST7735_RED 0xF800 00093 #define ST7735_GREEN 0x07E0 00094 #define ST7735_CYAN 0x07FF 00095 #define ST7735_MAGENTA 0xF81F 00096 #define ST7735_YELLOW 0xFFE0 00097 #define ST7735_WHITE 0xFFFF 00098 00099 #define BLACK 0x0000 00100 #define BLUE 0x001F 00101 #define RED 0xF800 00102 #define GREEN 0x07E0 00103 #define CYAN 0x07FF 00104 #define MAGENTA 0xF81F 00105 #define YELLOW 0xFFE0 00106 #define WHITE 0xFFFF 00107 00108 00109 00110 00111 class Adafruit_ST7735 : public Adafruit_GFX { 00112 00113 public: 00114 00115 Adafruit_ST7735( PinName CS, PinName RS, PinName RST, PinName BL); 00116 00117 void chipEnable(); 00118 void chipDisable(); 00119 void initB(void); // for ST7735B displays 00120 void initR(uint8_t options = INITR_GREENTAB); // for ST7735R 00121 void backlight( uint8_t setting ); 00122 void writeString( int x, int row, char * str, int flag ); 00123 void writeString( int x, int row, char * str, int flag, char size ); 00124 void setAddrWindow(uint8_t x0, uint8_t y0, uint8_t x1, uint8_t y1); 00125 void pushColor(uint16_t color); 00126 00127 void fillScreen(uint16_t color); 00128 void drawPixel(int16_t x, int16_t y, uint16_t color); 00129 void drawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color); 00130 void drawFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color); 00131 void fillRect(int16_t x, int16_t y, int16_t w, int16_t h, uint16_t color); 00132 void invertDisplay(boolean i); 00133 00134 00135 void bitmap( int16_t x, int16_t y, int16_t w, int16_t h, const unsigned char *map ); 00136 void bitmapBW(int16_t x, int16_t y, const unsigned char *map, int16_t w, int16_t h, int16_t foreground, int16_t background ); 00137 00138 void setRotation( uint8_t r); 00139 uint16_t Color565( uint8_t r, uint8_t g, uint8_t b ); 00140 00141 00142 private: 00143 00144 void spiwrite(uint8_t), 00145 writecommand(uint8_t c), 00146 writedata(uint8_t d), 00147 commandList(uint8_t *addr), 00148 commonInit(uint8_t *cmdList); 00149 00150 uint8_t colstart, rowstart; // some displays need this changed 00151 00152 // SPI lcdPort; // does SPI MOSI, MISO and SCK 00153 DigitalOut _cs; // does SPI CE 00154 DigitalOut _rs; // register/date select 00155 DigitalOut _rst; // does 3310 LCD_RST 00156 DigitalOut _bl; // backlight 00157 };
Generated on Thu Jul 14 2022 05:40:27 by
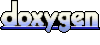