
Test
Dependencies: mbed AccelSensor
main.cpp
00001 #include "mbed.h" 00002 #include "AccelSensor.h" 00003 00004 const int addr = 0x9A; // define the I2C Address for TC74-A0 00005 const int Afficheur_addr = 0xE2; // define the I2C Address for 4*7seg display 00006 const int Accel_addr = 0x3A; // define the I2C Address for the Accelerometer 00007 00008 //Defines for the serial output for debug 00009 #define Output_Temperature true 00010 #define Output_Accel true 00011 #define Output_Accel_debug false 00012 00013 //Creating the desired objetcs 00014 I2C i2c(p9, p10); // sda, scl 00015 Serial pc(USBTX, USBRX); // tx, rx 00016 Serial Damn(p28,p27); // Used as shortcut for printing result on 7seg using printf function as it is not available in I2C 00017 AccelSensor acc(p9,p10); //Should be commented as it was a part of the prototype and test, we may want to use our own function 00018 //Mainly used to calibrate and start the device 00019 00020 void init(void); 00021 char get_Temperature(void); 00022 void Display8888(void); 00023 void Update_Accel(void); 00024 00025 int main() 00026 { 00027 char temp; 00028 00029 init(); 00030 wait(1); //Make sure system is fully initialized 00031 00032 Display8888(); //Display initial text 8888 on the display 00033 00034 00035 while(1) { 00036 pc.printf("\r\n\nStart reading the temperature of TC74 on I2C\r\n"); 00037 temp = get_Temperature(); 00038 Update_Accel(); 00039 00040 wait(2); 00041 00042 } 00043 } 00044 //------------------Accelerometre --------------------------- 00045 00046 void Update_Accel(void) 00047 { 00048 //Variables 00049 char cmd[7]; 00050 00051 //Initialisation 00052 cmd[0] = 0x00; //Command :: Status 00053 00054 //Read all the register for X,Y and Z forces 00055 i2c.write(Accel_addr, cmd, 1); //Issue required command to perform a write of the command 00056 i2c.read(Accel_addr, cmd, 7); //Read the Data from the device 00057 #if Output_Accel_debug 00058 pc.printf("Values :: %d %d %d %d %d %d %d \r\n\n", cmd[0], cmd[1], cmd[2],cmd[3],cmd[4],cmd[5],cmd[6] ); 00059 #endif 00060 //Damn.printf("v"); 00061 long double anglez, anglex,angley; 00062 bool negatif; 00063 //calcul angle Z 00064 if (cmd[5]<0x7F) { 00065 anglez = acos((cmd[5]/62.0))/3.1416*180; 00066 negatif=false; 00067 00068 } else { 00069 cmd[5]=(cmd[5]-256)*-1; 00070 anglez = acos((cmd[5]/62.0))/3.1416*180; 00071 00072 } 00073 00074 //calcul angle Y 00075 if (cmd[3]<0x7F) { 00076 angley = asin((cmd[3]/62.0))/3.1416*180; 00077 00078 } else { 00079 cmd[3]=(cmd[3]-256)*-1; 00080 angley = asin((cmd[3]/62.0))/3.1416*180; 00081 00082 } 00083 // calcul angle X 00084 if (cmd[1]<0x7F) { 00085 anglex = asin((cmd[1]/62.0))/3.1416*180; 00086 00087 } else { 00088 cmd[1]=(cmd[1]-256)*-1; 00089 anglex = asin((cmd[1]/62.0))/3.1416*180; 00090 00091 } 00092 00093 00094 //if (anglez<45 00095 int b = anglez; 00096 #if Output_Accel 00097 pc.printf("TEST: X=%f Y=%f Z=%f \r\n", anglex, angley, anglez ); 00098 #endif 00099 if (negatif==false) 00100 Damn.printf(" "); 00101 else 00102 Damn.printf(" -"); 00103 00104 Damn.printf("%2d", b ); 00105 00106 00107 //-------------------Accel status form Imported Class -------- 00108 #if Output_Accel_debug 00109 int result[3]; 00110 acc.readData(result); 00111 pc.printf("X: %d Y: %d Z: %d \r\n", result[0],result[1],result[2]); 00112 #endif 00113 00114 00115 00116 } 00117 00118 void init(void) 00119 { 00120 //Let's clear the I2C display 00121 00122 i2c.write(Afficheur_addr,"v",1); 00123 00124 00125 acc.init(); 00126 acc.active(); 00127 } 00128 00129 char get_Temperature(void) 00130 { 00131 char cmd[1]; 00132 //Méthode 1 00133 //Utilisation des fonctions bas niveau 00134 /* 00135 i2c.start(); // Start condition 00136 a = i2c.write(addr); // Write Device Address 00137 b = i2c.write(0x00); // Write READ command of TC74 (voir page 8 de la datasheet du TC74) 00138 00139 i2c.start(); //Reissue start condition 00140 //Au lieu de faire Stop condition et Start de nouveau 00141 00142 c= i2c.write(addr|1); //Adresse du Device en mode Lecture 00143 temp = i2c.read(0); //Lecture de la valeur du registre de température 00144 i2c.stop(); //Fermeture de la trame 00145 */ 00146 //Méthode 2 00147 //Utilisation des fonctions haut niveau 00148 00149 cmd[0] = 0x0; //Command :: READ 00150 i2c.write(addr, cmd, 1); //Issue required command to perform a write of the command 00151 i2c.read(addr, cmd, 1); //Read the Data from the device 00152 00153 //-----------------Print out section ---------------------- 00154 //Display device Address and informations 00155 00156 // pc.printf("Device with address 0x%x with\r\n", addr); 00157 00158 //Prints out the result of Method 1 00159 //pc.printf("ACK1 :: %d\n\rACK2 :: %d\n\rACK3 :: %d\n\r", a,b,c); //ACK bits 00160 //pc.printf("Method 1 :: %d\n\r", temp); 00161 00162 //Prints out the Data from Method 2 00163 #if Output_Temperature 00164 pc.printf("Temperature :: %d\r\n\n", cmd[0]); 00165 #endif 00166 00167 return cmd[0]; 00168 } 00169 00170 /* 00171 ** This function display '8888' on the Hex Display using I2C transactions 00172 ** For prototyping purpose, we will use the Serial protocol for transferring the data to the Hex display 00173 */ 00174 void Display8888(void) 00175 { 00176 char cmd[2]; 00177 //--------------Afficheur LCD ------------------------------ 00178 00179 cmd[0] = 0x7b; //Segment 1 00180 cmd[1] = 0x7f; 00181 00182 i2c.write(Afficheur_addr,cmd,2); 00183 00184 wait(0.07); 00185 00186 cmd[0] = 0x7c; //Segment 2 00187 cmd[1] = 0x7f; 00188 00189 i2c.write(Afficheur_addr,cmd,2); 00190 00191 wait(0.07); 00192 00193 cmd[0] = 0x7d; //Segment 3 00194 cmd[1] = 0x7f; 00195 00196 i2c.write(Afficheur_addr,cmd,2); 00197 00198 wait(0.07); 00199 00200 cmd[0] = 0x7e; //Segment 4 00201 cmd[1] = 0x7f; 00202 00203 i2c.write(Afficheur_addr,cmd,2); 00204 }
Generated on Tue Jul 19 2022 02:31:41 by
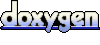