SO1602A Lib. SO1602A is Organic LED, has 16 chars and 2 lines. This lib supports printf() of C-Language. http://akizukidenshi.com/catalog/g/gP-08276/
Dependents: NEW_LineTraceHub NEW_LineTraceHub_2 ColorSensorTest
SO1602A.cpp
00001 #include "SO1602A.h" 00002 00003 SO1602A::SO1602A (PinName sda, PinName scl, char address) 00004 : p_i2c(new I2C(sda, scl)), i2c(*p_i2c), addr(address) 00005 { 00006 init(); 00007 } 00008 SO1602A::SO1602A (I2C &_i2c, char address) 00009 : p_i2c(NULL), i2c(_i2c), addr(address) 00010 { 00011 init(); 00012 } 00013 SO1602A::~SO1602A() 00014 { 00015 if(p_i2c != NULL) 00016 delete p_i2c; 00017 } 00018 00019 bool SO1602A::cmd(char chr) 00020 { 00021 buf[0]= 0x00; 00022 buf[1]= chr; 00023 // if write success:0, other: err code. 00024 int status= i2c.write(addr, buf, 2); 00025 wait_ms(3); 00026 00027 if(status == 0) 00028 return true; 00029 else 00030 return false; 00031 } 00032 00033 int SO1602A::_putc(int val) // for printf() 00034 { 00035 if (val == '\n') { 00036 col = 0; 00037 row = (row + 1) % 2; 00038 } else { 00039 locate(col, row); 00040 buf[0]= 0x40; 00041 buf[1]= val; 00042 i2c.write(addr, buf, 2); 00043 00044 col++; 00045 if (col >= 16) { 00046 col = 0; 00047 row = (row + 1) % 2; 00048 } 00049 } 00050 wait_ms(1); 00051 return val; 00052 } 00053 00054 // for "Stream" 00055 int SO1602A::_getc() 00056 { 00057 return -1; 00058 } 00059 00060 void SO1602A::locate(int _col, int _row) 00061 { 00062 col= _col; 00063 row= _row; 00064 cmd(0x80 + row * 0x20 + col); 00065 return; 00066 } 00067 00068 void SO1602A::init() 00069 { 00070 col= row= 0; 00071 buf[0]= 0x00; 00072 buf[1]= 0x00; 00073 buf[2]= 0x00; 00074 00075 wait_ms(10); 00076 this->clear(); 00077 this->cmd(0x02); //Return Home. 00078 this->setDispFlag(true, true, true); 00079 00080 return; 00081 } 00082 00083 void SO1602A::setContrast(char val) 00084 { 00085 // Cmd of Contrast-setting must be setted Ext-Func-Register RE & SD. 00086 this->setRE(); 00087 this->setSD(); 00088 // Double Byte Command. The contrast has 256 steps, and increase as the value. 00089 this->cmd(0x81); 00090 this->cmd(val); 00091 this->clearSD(); 00092 this->clearRE(); 00093 wait_ms(100); 00094 return; 00095 } 00096 00097 void SO1602A::setDispFlag(bool disp, bool cursor, bool blink) 00098 { 00099 // set On/Off. b3=1, b2:Disp, b1:Cursor, b0:blink. 00100 char tmp= 0x08; 00101 if(disp) 00102 tmp += 0x04; 00103 if(cursor) 00104 tmp += 0x02; 00105 if(blink) 00106 tmp += 0x01; 00107 this->cmd(tmp); 00108 this->cmd(0x01); //Clear Disp. 00109 wait_ms(20); 00110 return; 00111 } 00112 00113 void SO1602A::clear() 00114 { 00115 this->cmd(0x01); 00116 locate(0, 0); 00117 wait_ms(5); 00118 return; 00119 } 00120 00121 // ******************** FUNCTION SET ********************** 00122 // RE & IS func-set -> b7-4: 001*. 00123 // b3: dispLine; 1(2&4), 0(1&3). 00124 // RE: b1, IS: b0. 00125 void SO1602A::setRE() 00126 { 00127 this->cmd(0x2a); 00128 return; 00129 } 00130 void SO1602A::clearRE() 00131 { 00132 this->cmd(0x28); 00133 return; 00134 } 00135 // Extention Register; SD. 00136 // RE setted, 0b 0111 100F. F= Flag; 0: OLED-cmd is disable. 00137 // 1: enable. 00138 void SO1602A::setSD() 00139 { 00140 this->cmd(0x79); 00141 return; 00142 } 00143 void SO1602A::clearSD() 00144 { 00145 this->cmd(0x78); 00146 return; 00147 } 00148 00149 // EOF
Generated on Sun Jul 17 2022 01:40:33 by
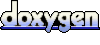