A program designed to run on the microbit. Used for driving a buggy.
Embed:
(wiki syntax)
Show/hide line numbers
buggy_function.h
00001 /********************************************************* 00002 *buggy_functions.h * 00003 *Author: Elijah Orr & Dan Argust * 00004 * * 00005 *A library of functions that can be used to control the * 00006 *RenBuggy. * 00007 *********************************************************/ 00008 00009 /* include guards are used to prevent problems caused by 00010 multiple definitions */ 00011 #ifndef BUGGY_FUNCTIONS_H 00012 #define BUGGY_FUNCTIONS_H 00013 00014 /* mbed.h must be included in this file also */ 00015 00016 00017 /* #define LeftMotorPin P0_3 tells the preprocessor to replace 00018 any mention of LeftMotorPin with P0_3 etc. 00019 This currently used the Large pads labled 0 and 1. 00020 P0_18 is equivelent to pad 8. 00021 P0_16 is equivelent to pad 16. 00022 potential pads could be P0,P1,P8,P12,P2,P16 00023 */ 00024 #define LeftMotorPin P0_18 00025 #define RightMotorPin P0_16 00026 #define follow_Speed 0.3 00027 00028 00029 /*This typedef defines what direction the buggy can go in, it is also used to determine what to display.*/ 00030 typedef enum { 00031 Up, 00032 Down, 00033 Left, 00034 Right, 00035 TopLeft, 00036 TopRight, 00037 BottomLeft, 00038 BottomRight, 00039 Stop, 00040 Clear, 00041 }Direction; 00042 00043 /* these are function prototypes that declare all the functions 00044 in the library.*/ 00045 extern void forward(float); //Move the buggy forward for (float) seconds 00046 extern void left(float); //Turn left for (float) seconds 00047 extern void right(float); //Turn right for (float) seconds 00048 extern void hold(float); //Hold the buggy in an idle state for (float) seconds 00049 extern void bear_Right(float); // Turns the buggy to the right while moving forward 00050 extern void bear_Left(float); // Turns the buggy to the left while moving forward 00051 extern void stop(); //Stop all motors 00052 extern void follow_Line(float); 00053 extern void follow_Line(); 00054 00055 extern void readButton(float); //Similar to hold, but scans for button presses while waiting 00056 extern int rollDice(); //Randomly generate a number a display it on the seven segment display 00057 00058 00059 /* stop() and rollDice() do not need to be giving a float 00060 to be run unlike the other functions which use a (float) 00061 to know how long they should run for */ 00062 00063 #endif // BUGGY_FUNCTIONS_H
Generated on Fri Jul 15 2022 13:57:07 by
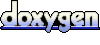