This library is a very low level interface to a transmit IR codes. Other libraries could be built on top of it to transmit different manufactures codes, or a specific code. I use the library to remotely trigger a Nikon DSLR camera the same way an ML3 Nikon remote does.
TxIR.cpp
00001 00002 /****************************************************************************** 00003 * Low level infrared transmission 00004 * This library provides a low level interface to send IR commands. in principle 00005 * other higher level IR type remotes could be build on top of this. I wrote 00006 * this code to send IR commands to a Nikon camera, which it works rather well 00007 * for. 00008 * 00009 * Copyright (C) 2010 Ali Saidi 00010 * 00011 * Permission is hereby granted, free of charge, to any person obtaining a copy 00012 * of this software and associated documentation files (the "Software"), to deal 00013 * in the Software without restriction, including without limitation the rights 00014 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00015 * copies of the Software, and to permit persons to whom the Software is 00016 * furnished to do so, subject to the following conditions: 00017 * 00018 * The above copyright notice and this permission notice shall be included in 00019 * all copies or substantial portions of the Software. 00020 * 00021 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00022 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00023 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00024 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00025 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00026 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00027 * THE SOFTWARE. 00028 */ 00029 00030 #include "TxIR.hpp" 00031 00032 00033 void 00034 TxIR::doAction() 00035 { 00036 if (pos >= len) { 00037 delete [] data; 00038 _inUse = false; 00039 txPin.write(0); 00040 return; 00041 } 00042 00043 delay.attach_us(this, &TxIR::doAction, data[pos++]); 00044 if (high) 00045 txPin.write(0); 00046 else 00047 txPin.write(0.5); 00048 high = !high; 00049 } 00050 00051 bool 00052 TxIR::txSeq(unsigned freq, unsigned _len, const unsigned *_data) 00053 { 00054 // @todo a lock or semaphore should be used here 00055 if (inUse()) 00056 return false; 00057 _inUse = true; 00058 00059 // keep a copy of the data, so it can't change 00060 len = _len; 00061 data = new unsigned[len]; 00062 memcpy(data, _data, len * sizeof(unsigned)); 00063 pos = 0; 00064 00065 // setup the PWM 00066 txPin.write(0.0); 00067 txPin.period_us(freq); 00068 00069 // Get the interrupt ready 00070 delay.detach(); 00071 high = true; 00072 delay.attach_us(this, &TxIR::doAction, data[pos++]); 00073 00074 // Begin 00075 txPin.write(0.5); 00076 00077 return true; 00078 }
Generated on Wed Jul 13 2022 00:19:24 by
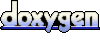