
xrocusOS_ADXL355 version
Dependencies: mbed SDFileSystem
UartReceiver.h
00001 #ifndef _UARTRECEIVER_H_ 00002 #define _UARTRECEIVER_H_ 00003 00004 00005 /** --- Includes --- */ 00006 #include "mbed.h" 00007 00008 /** --- Global Structs --- */ 00009 class UartReceiver 00010 { 00011 private: 00012 /** defines */ 00013 static const int RXBUF_SIZE = 64; 00014 static const int RXBUF_NEST = 4; 00015 static const int BufOpen = 0; 00016 static const int BufFixed = 1; 00017 public: 00018 static const int MaxStr = (RXBUF_SIZE - 1); 00019 00020 private: 00021 /** varialbles */ 00022 Serial *pUart; 00023 void(*pLineHandler)(char*); 00024 char inner_buffer[RXBUF_NEST][RXBUF_SIZE]; 00025 int nesthead; 00026 int nesttail; 00027 int tail; 00028 int head; 00029 00030 private: 00031 /* Constructor(CouldNotUse) */ 00032 UartReceiver(void); 00033 00034 public: 00035 /* Constructor(Accepted) */ 00036 UartReceiver(Serial *pSetUart); 00037 /* Start Receiving */ 00038 void run(void); 00039 /* Stop Receiving */ 00040 void stop(void); 00041 /* set Line Handler */ 00042 void* setLineHandler(void(*setLineHandler)(char*)); 00043 /* Export Fixed Buffer Pointer */ 00044 char* dequeue_str(void); 00045 /* Recv char from UART and Concat to Liquid Buffer */ 00046 void concat(); 00047 /* Get Pointer */ 00048 Serial *getCurrentUart(void); 00049 private: 00050 /* Concat to Liquid Buffer */ 00051 /** return UartReceiver::BufFixed, UartReceiver::BufOpen */ 00052 int enqueue_ch(char ch); 00053 }; 00054 00055 00056 #endif /* _UARTRECEIVER_H_ */
Generated on Sun Jul 17 2022 15:09:45 by
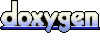