
xrocusOS_ADXL355 version
Dependencies: mbed SDFileSystem
TimeManager.cpp
00001 #include "TimeManager.h" 00002 #include "global.h" 00003 00004 00005 /* Cyclic handler (for tick) */ 00006 void flip() { 00007 pTM->tick(); 00008 } 00009 00010 /* Constractor */ 00011 TimeManager::TimeManager(void) 00012 { 00013 current.tm_sec = 0; 00014 current.tm_min = 0; 00015 current.tm_hour = 0; 00016 current.tm_mday = 1; 00017 current.tm_mon = 1; 00018 current.tm_year = 0; 00019 baseclock.attach(flip, 1.0); 00020 } 00021 00022 /* tick (currenttime++) */ 00023 void TimeManager::tick(void) 00024 { 00025 int daysInMonth = getDaysInMonth(current.tm_mon, current.tm_year); 00026 current.tm_sec++; 00027 if (current.tm_sec >= 60) { 00028 current.tm_sec = 0; 00029 current.tm_min++; 00030 } else { return; } 00031 if (current.tm_min >= 60) { 00032 current.tm_min = 0; 00033 current.tm_hour++; 00034 } else { return; } 00035 if (current.tm_hour >= 24) { 00036 current.tm_hour = 0; 00037 current.tm_mday++; 00038 } else { return; } 00039 if (current.tm_mday > daysInMonth) { 00040 current.tm_mday = 0; 00041 current.tm_mon++; 00042 } else { return; } 00043 if (current.tm_mon > 12) { 00044 current.tm_mon = 1; 00045 current.tm_year++; 00046 } else { return; } 00047 } 00048 00049 /* copy Struct Time to arg */ 00050 void TimeManager::getCurrentTime(struct tm *ret) 00051 { 00052 *ret = current; 00053 } 00054 00055 /* get string of timestamp */ 00056 int TimeManager::getTimeStamp(char *dst) 00057 { 00058 char dummy[TimeStampLength + 1]; 00059 sprintf(dummy, "%04d-%02d-%02d_%02d-%02d-%02d", 00060 current.tm_year + 1900, current.tm_mon, current.tm_mday, 00061 current.tm_hour, current.tm_min, current.tm_sec); 00062 memcpy(dst, dummy, TimeStampLength); 00063 return TimeStampLength; 00064 } 00065 00066 /* set time parameters */ 00067 bool TimeManager::setCurrentTime(int selector, int set) 00068 { 00069 bool finish = false; 00070 int daysInMonth = getDaysInMonth(current.tm_mon, current.tm_year); 00071 dbgprintf("setCurrentTime:%d-%d(%d)\n", selector, set, daysInMonth); 00072 switch (selector) { 00073 case SetTimeMethod::Year: 00074 if (set >= 0) { 00075 current.tm_year = set; 00076 finish = true; 00077 } 00078 break; 00079 case SetTimeMethod::Month: 00080 if (set > 0 && set <= 12) { 00081 current.tm_mon = set; 00082 finish = true; 00083 } 00084 break; 00085 case SetTimeMethod::Day: 00086 if (set > 0 && set <= daysInMonth) { 00087 current.tm_mday = set; 00088 finish = true; 00089 } 00090 break; 00091 case SetTimeMethod::Hour: 00092 if (set >= 0 && set < 24) { 00093 current.tm_hour = set; 00094 finish = true; 00095 } 00096 break; 00097 case SetTimeMethod::Min: 00098 if (set >= 0 && set < 60) { 00099 current.tm_min = set; 00100 finish = true; 00101 } 00102 break; 00103 case SetTimeMethod::Sec: 00104 if (set >= 0 && set < 60) { 00105 current.tm_sec = set; 00106 finish = true; 00107 } 00108 break; 00109 }; 00110 return finish; 00111 } 00112 00113 /* calcurate Number of Days in month */ 00114 int TimeManager::getDaysInMonth(int month, int year) 00115 { 00116 int daysInMonth; 00117 bool leap; 00118 year += 1900; 00119 leap = (year % 400 == 0 || (year % 4 == 0 && year % 100 != 0))? true : false; 00120 00121 if (((month % 2 == 1) && (month <= 7)) || 00122 ((month % 2 == 0) && (month >= 8))) { 00123 /* 31days/month */ 00124 daysInMonth = 31; 00125 } else if (month == 2) { 00126 /* 28days OR 29days/month */ 00127 if (leap) { 00128 daysInMonth = 29; 00129 } else { 00130 daysInMonth = 28; 00131 } 00132 } else { 00133 /* 30days/month */ 00134 daysInMonth = 30; 00135 } 00136 return daysInMonth; 00137 }
Generated on Sun Jul 17 2022 15:09:45 by
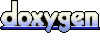