
xrocusOS_ADXL355 version
Dependencies: mbed SDFileSystem
CommandParser.cpp
00001 #include "global.h" 00002 00003 /* UartLineHandler (start parsing) */ 00004 static void lineHandler(char *line) 00005 { 00006 pCP->parse(line); 00007 } 00008 00009 /* constructor */ 00010 CommandParser::CommandParser(UartReceiver *setUartReceiver, int setDeviceID, 00011 CmdParseRule *setRuleTable, int setRuleTableLen) 00012 { 00013 pR = setUartReceiver; 00014 pR->setLineHandler(lineHandler); 00015 deviceID = setDeviceID; 00016 ruleTable = setRuleTable; 00017 ruleTableLen = setRuleTableLen; 00018 pUart = pR->getCurrentUart(); 00019 } 00020 00021 /* start parsing */ 00022 void CommandParser::run(void) 00023 { 00024 pR->run(); 00025 } 00026 00027 /* process parsing */ 00028 int CommandParser::parse(char *pStr) 00029 { 00030 int rn; 00031 int len; 00032 char *head; 00033 /** skip - empty command*/ 00034 if (pStr[0] == '\0') { 00035 uprintf("(empty)\n"); 00036 return 0; 00037 } 00038 /* protection for buffer overrun */ 00039 pStr[UartReceiver::MaxStr] = '\0'; 00040 len = strlen(pStr); 00041 00042 /* parsing */ 00043 head = pStr; 00044 //uprintf("%s\n", pStr); 00045 00046 /** Command Format ":0 CMD 0000" */ 00047 if (len != CmdLen) { 00048 this->reply(false, CommandParser::NakCode::NAK_INVAL_LEN); 00049 return -1; 00050 } 00051 /** check Command Header */ 00052 if (head[0] != ':') { 00053 this->reply(false, CommandParser::NakCode::NAK_INVAL_FMT); 00054 return -1; 00055 } 00056 /** check Command DeviceID */ 00057 if ((head[1] - '0') != deviceID) { 00058 return 0; 00059 } 00060 00061 /** search Command */ 00062 head += 3; 00063 for(rn = 0; rn < ruleTableLen; rn++) { 00064 int check; 00065 check = strncmp((const char*)(ruleTable[rn].cmdName), 00066 (const char*)(head),CmdNameLen); 00067 if (check != 0) { 00068 continue; 00069 } 00070 head += 4; 00071 return (*ruleTable[rn].func)(this, head, 00072 ruleTable[rn].exarg); 00073 00074 } 00075 this->reply(false, CommandParser::NakCode::NAK_INVAL_CMD); 00076 return -1; 00077 } 00078 00079 /* get my Device ID */ 00080 int CommandParser::getDeviceID(void) 00081 { 00082 return deviceID; 00083 } 00084 00085 /* generate ACK/NAK with int code */ 00086 void CommandParser::reply(bool ack, int replyCode) 00087 { 00088 char replyStr[] = "0000"; 00089 sprintf(replyStr, "%04d", replyCode); 00090 this->reply(ack, replyStr); 00091 } 00092 00093 /* generate ACK/NAK with String */ 00094 void CommandParser::reply(bool ack, char* replyStr) 00095 { 00096 const char CmdReplyStrDefault[] = ":0 ACK 0004 0000\n"; 00097 const int CmdReplyLen = sizeof(CmdReplyStrDefault) /* include \0 */; 00098 const int CmdReplyDevPos = 1; 00099 const int CmdReplyAckPos = 3; 00100 const int CmdReplyCodePos = 12; 00101 const int CmdReplyEndPos = 16; 00102 00103 char str[CmdReplyLen]; 00104 00105 /* set default handler */ 00106 memcpy(str, CmdReplyStrDefault, CmdReplyLen); 00107 str[CmdReplyDevPos] = '0' + getDeviceID(); 00108 if (!ack) { 00109 memcpy(&str[CmdReplyAckPos], "NAK", 3); 00110 } 00111 memcpy(&str[CmdReplyCodePos], replyStr, 4); 00112 str[CmdReplyEndPos] = '\n'; 00113 uprintf(str); 00114 } 00115 00116 /* get currentUart */ 00117 Serial *CommandParser::getCurrentUart(void) 00118 { 00119 return pUart; 00120 }
Generated on Sun Jul 17 2022 15:09:45 by
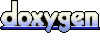