
SmartMesh QSL for STM32F4 version
Fork of COG-AD4050_QSL by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 /* 00003 Copyright (c) 2016, Dust Networks. All rights reserved. 00004 00005 SimplePublish example application for the mbed OS. 00006 00007 \license See attached DN_LICENSE.txt. 00008 */ 00009 00010 #include "mbed.h" 00011 #include "millis.h" 00012 #include "dn_qsl_api.h" // Only really need this include from QSL 00013 #include "dn_debug.h" // Included to borrow debug macros 00014 #include "dn_endianness.h" // Included to borrow array copying 00015 #include "dn_time.h" // Included to borrow sleep function 00016 //#include "ADXL362.h" 00017 00018 #define NETID 0 // Factory default value used if zero (1229) 00019 #define JOINKEY NULL // Factory default value used if NULL (44 55 53 54 4E 45 54 57 4F 52 4B 53 52 4F 43 4B) 00020 #define BANDWIDTH_MS 500 // Not changed if zero (default base bandwidth given by manager is 9 s) 00021 #define SRC_PORT 60000 // Default port used if zero (0xf0b8) 00022 #define DEST_PORT 0 // Default port used if zero (0xf0b8) 00023 #define DATA_PERIOD_MS 500 // Should be longer than (or equal to) bandwidth 00024 00025 // We can use debug macros from dn_debug, as stdio defaults to this serial 00026 Serial serialDebug(USBTX, USBRX); 00027 #define log_info serialDebug.printf 00028 00029 // LED2 is the green LED on the NUCLEO-L053R8; might change for other boards 00030 DigitalOut myled(LED1); 00031 DigitalOut status(LED2); 00032 //ADXL362 Acc(P1_07, P1_08, P1_06, P1_10); 00033 00034 static uint16_t randomWalk(void); 00035 static void parsePayload(const uint8_t *payload, uint8_t size); 00036 00037 //void init_acc(void) 00038 //{ 00039 // Acc.init_spi(); 00040 // Acc.init_adxl362(); 00041 //} 00042 00043 int main() 00044 { 00045 uint8_t payload[4]; 00046 uint8_t inboxBuf[DN_DEFAULT_PAYLOAD_SIZE_LIMIT]; 00047 uint8_t bytesRead; 00048 uint8_t i,e; 00049 //int8_t x0, y0, z0; 00050 00051 myled=1; 00052 status=1; 00053 00054 for(e=0;e<10;e++) 00055 { 00056 myled=!myled; 00057 status=!status; 00058 wait(0.5); 00059 } 00060 00061 myled=1; 00062 status=1; 00063 00064 //init_acc(); 00065 // Configure SysTick for timing 00066 millisStart(); 00067 00068 // Set PC debug serial baudrate 00069 serialDebug.baud(115200); 00070 serialDebug.printf("STM32F4 SmartMesh Demo\n"); 00071 00072 log_info("Initializing..."); 00073 dn_qsl_init(); 00074 00075 // Flash LED to indicate start-up complete 00076 for (i = 0; i < 10; i++) 00077 { 00078 myled = !myled; 00079 dn_sleep_ms(50); 00080 } 00081 myled=0; 00082 00083 while(TRUE) { 00084 if (dn_qsl_isConnected()) 00085 { 00086 //Acc.ACC_GetXYZ8(&x0, &y0, &z0); 00087 //payload[0]=x0; 00088 //payload[1]=y0; 00089 //payload[2]=z0; 00090 uint16_t val = randomWalk(); 00091 static uint8_t count = 0; 00092 myled = 1; // Turn off LED during send/read 00093 status = 0; 00094 dn_write_uint16_t(payload, val); 00095 payload[0] = count; 00096 00097 if (dn_qsl_send(payload, sizeof (payload), DEST_PORT)) 00098 { 00099 log_info("Sent message nr %u: %u", count, val); 00100 count++; 00101 status=1; 00102 } else 00103 { 00104 log_info("Send failed"); 00105 status=0; 00106 } 00107 00108 do 00109 { 00110 bytesRead = dn_qsl_read(inboxBuf); 00111 parsePayload(inboxBuf, bytesRead); 00112 } while (bytesRead > 0); 00113 00114 myled = 1; // Turn on LED 00115 dn_sleep_ms(DATA_PERIOD_MS); 00116 } else 00117 { 00118 log_info("Connecting..."); 00119 myled = 1; // Not connected; turn off LED 00120 if (dn_qsl_connect(NETID, JOINKEY, SRC_PORT, BANDWIDTH_MS)) 00121 { 00122 myled = 0; // Connected; turn on LED 00123 status=1;//log_info("Connected to network"); 00124 } else 00125 { 00126 log_info("Failed to connect"); 00127 } 00128 } 00129 status=0; 00130 wait(0.5); 00131 status=1; 00132 } 00133 } 00134 00135 static uint16_t randomWalk(void) 00136 { 00137 static bool first = TRUE; 00138 static uint16_t lastValue = 0x7fff; // Start in middle of uint16 range 00139 const int powerLevel = 9001; 00140 00141 // Seed random number generator on first call 00142 if (first) 00143 { 00144 first = FALSE; 00145 srand(dn_time_ms()); 00146 } 00147 00148 // Random walk within +/- powerLevel 00149 lastValue += rand() / (RAND_MAX / (2*powerLevel) + 1) - powerLevel; 00150 return lastValue; 00151 } 00152 00153 static void parsePayload(const uint8_t *payload, uint8_t size) 00154 { 00155 uint8_t i; 00156 char msg[size + 1]; 00157 00158 if (size == 0) 00159 { 00160 // Nothing to parse 00161 return; 00162 } 00163 00164 // Parse bytes individually as well as together as a string 00165 //log_info("Received downstream payload of %u bytes:", size); 00166 for (i = 0; i < size; i++) 00167 { 00168 msg[i] = payload[i]; 00169 log_info("\tByte# %03u: %#.2x (%u)", i, payload[i], payload[i]); 00170 } 00171 msg[size] = '\0'; 00172 log_info("\tMessage: %s", msg); 00173 }
Generated on Tue Jul 12 2022 21:33:36 by
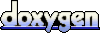