
SmartMesh QSL for STM32F4 version
Fork of COG-AD4050_QSL by
Embed:
(wiki syntax)
Show/hide line numbers
dn_qsl_api.h
00001 /* 00002 Copyright (c) 2016, Dust Networks. All rights reserved. 00003 00004 QuickStart Library API. 00005 00006 \license See attached DN_LICENSE.txt. 00007 */ 00008 00009 #ifndef DN_QSL_API_H 00010 #define DN_QSL_API_H 00011 00012 #include "dn_common.h" 00013 #include "dn_defaults.h" 00014 00015 //=========================== defines ========================================= 00016 00017 #define DN_DEST_IP DN_DEFAULT_DEST_IP 00018 00019 //=========================== typedef ========================================= 00020 00021 //=========================== variables ======================================= 00022 00023 //=========================== prototypes ====================================== 00024 00025 #ifdef __cplusplus 00026 extern "C" { 00027 #endif 00028 00029 //===== init 00030 00031 /** 00032 \brief Initialize the necessary structures and underlying C Library. 00033 00034 Has to be run once before any of the other functions below is run. 00035 00036 \return A boolean indicating the success of the initialization. 00037 */ 00038 bool dn_qsl_init(void); 00039 00040 00041 //===== isConnected 00042 00043 /** 00044 \brief Return TRUE if the mote is connected to a network. 00045 00046 Simply checks if the FSM is in the CONNECTED state. 00047 00048 \return A boolean indicating if the mote is connected to a network. 00049 */ 00050 bool dn_qsl_isConnected(void); 00051 00052 00053 //===== connect 00054 00055 /** 00056 \brief Attempt to make the mote connect to a network with the given parameters. 00057 00058 If the passed network ID, join key or source port is zero/NULL, default values 00059 are used. If non-zero, the passed service will be requested after joining a 00060 network. A new service can be requested later by calling connect again with the 00061 same network ID, join key and source port. Further, if the requested service is 00062 also indifferent, the function simply returns TRUE. 00063 00064 \param netID The ID of the network to attempt to connect with. 00065 \param joinKey The join key to use in the connection attempt. 00066 \param srcPort The port that you expect to get downstream data on. 00067 \param service_ms The service to request after establishing a connection, given in milliseconds. 00068 \return A boolean indicating if the connection attempt and/or service request was successful. 00069 */ 00070 bool dn_qsl_connect(uint16_t netID, const uint8_t* joinKey, uint16_t srcPort, uint32_t service_ms); 00071 00072 00073 //===== send 00074 00075 /** 00076 \brief Send a packet into the network. 00077 00078 Tells the mote send a packet with the given payload into the network. 00079 Said packet is sent to the provided destination port (default if 0) and the 00080 IPv6 address defined by DEST_IP (default is the well-known manager address). 00081 The FSM waits for a confirmation from the mote that the packet was accepted 00082 and queued up for transmission. Note that end-to-end delivery is not 00083 guaranteed with the utilized UDP, but reliability is typically > 99.9 %. 00084 00085 \param payload Pointer to a byte array containing the payload. 00086 \param payloadSize_B Byte size of the payload. 00087 \param destPort The destination port for the packet. 00088 \return A boolean indicating if the packet was queued up for transmission. 00089 */ 00090 bool dn_qsl_send(const uint8_t* payload, uint8_t payloadSize_B, uint16_t destPort); 00091 00092 00093 //===== read 00094 00095 /** 00096 \brief Read the FIFO buffered inbox of downstream messages. 00097 00098 As the payload of downstream messages are pushed into a buffered FIFO inbox, 00099 calling this function will pop the first one (oldest) stored into the provided 00100 buffer and return the byte size. An empty inbox will simply return 0. 00101 00102 \param readBuffer Pointer to a byte array to store the read message payload. 00103 \return The number of bytes read into the provided buffer. 00104 */ 00105 uint8_t dn_qsl_read(uint8_t* readBuffer); 00106 00107 #ifdef __cplusplus 00108 } 00109 #endif 00110 00111 #endif
Generated on Tue Jul 12 2022 21:33:36 by
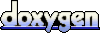