
SmartMesh QSL for STM32F4 version
Fork of COG-AD4050_QSL by
Embed:
(wiki syntax)
Show/hide line numbers
dn_endianness.c
00001 /* 00002 Copyright (c) 2016, Dust Networks. All rights reserved. 00003 00004 Port of the endianness module to the NUCLEO-L053R8. 00005 00006 \license See attached DN_LICENSE.txt. 00007 */ 00008 00009 #include "dn_endianness.h" 00010 00011 //=========================== variables ======================================= 00012 00013 //=========================== prototypes ====================================== 00014 00015 //=========================== public ========================================== 00016 00017 void dn_write_uint16_t(uint8_t* ptr, uint16_t val){ 00018 // STM32L0 is a little-endian platform 00019 ptr[0] = (val>>8) & 0xff; 00020 ptr[1] = (val>>0) & 0xff; 00021 } 00022 00023 void dn_write_uint32_t(uint8_t* ptr, uint32_t val){ 00024 // STM32L0 is a little-endian platform 00025 ptr[0] = (val>>24) & 0xff; 00026 ptr[1] = (val>>16) & 0xff; 00027 ptr[2] = (val>>8) & 0xff; 00028 ptr[3] = (val>>0) & 0xff; 00029 } 00030 00031 void dn_read_uint16_t(uint16_t* to, uint8_t* from){ 00032 // STM32L0 is a little-endian platform 00033 *to = 0; 00034 *to |= (from[1]<<0); 00035 *to |= (from[0]<<8); 00036 } 00037 00038 void dn_read_uint32_t(uint32_t* to, uint8_t* from){ 00039 // STM32L0 is a little-endian platform 00040 *to = 0; 00041 *to |= ( ((uint32_t)from[3])<<0 ); 00042 *to |= ( ((uint32_t)from[2])<<8 ); 00043 *to |= ( ((uint32_t)from[1])<<16); 00044 *to |= ( ((uint32_t)from[0])<<24); 00045 } 00046 00047 //=========================== private ========================================= 00048 00049 //=========================== helpers ========================================= 00050
Generated on Tue Jul 12 2022 21:33:36 by
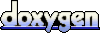