
a
Dependencies: mbed mbed-STM32F103C8T6
main.cpp
00001 #include "stm32f103c8t6.h" 00002 #include "mbed.h" 00003 00004 #define pow1 0.5 00005 #define pow2 0.7 00006 #define time 0.7 00007 00008 DigitalOut led( LED1); 00009 CANMessage msg; 00010 CAN Reseive ( PA_11, PA_12); 00011 00012 PwmOut dcY( PA_8);//Y軸のpwmピン 00013 DigitalOut motordcY1( PB_15); 00014 DigitalOut motordcY2( PB_14); 00015 00016 PwmOut dcZ( PB_3);//Z軸のpwmピン 00017 DigitalOut motordcZ1( PA_15); 00018 DigitalOut motordcZ2( PA_12); 00019 00020 DigitalIn switch1( PA_1); 00021 DigitalIn switch2( PA_2); 00022 DigitalIn switch3( PA_3); 00023 DigitalIn switch4( PA_4);//micro switch x 4 00024 00025 int mode = 0; 00026 00027 void motorForward() 00028 { 00029 dcY.period_us( 50); 00030 dcY = pow1; 00031 motordcY1 = 1; 00032 motordcY2 = 0; 00033 } 00034 void motorBack() 00035 { 00036 dcY.period_us( 50); 00037 dcY = pow1; 00038 motordcY1 = 0; 00039 motordcY2 = 1; 00040 } 00041 void motorDown() 00042 { 00043 dcZ.period_us( 50); 00044 dcZ = pow2; 00045 motordcZ1 = 0; 00046 motordcZ2 = 1; 00047 } 00048 void motorUp() 00049 { 00050 dcZ.period_us( 50); 00051 dcZ = pow2; 00052 motordcZ1 = 1; 00053 motordcZ2 = 0; 00054 } 00055 void motorStopY()//Y軸が止まる 00056 { 00057 motordcY1 = 0; 00058 motordcY2 = 0; 00059 } 00060 void case0(){ 00061 if( switch1.read() == 0){ 00062 motorBack(); 00063 if( switch1.read() == 1) 00064 motorStopY(); 00065 } 00066 else if( switch1.read() == 1) 00067 motorStopY(); 00068 mode = 1; 00069 } 00070 void case1(){ 00071 motorForward(); 00072 motorUp(); 00073 //wait( time); 00074 mode = 2; 00075 } 00076 void case2(){ 00077 motorForward(); 00078 motorDown(); 00079 //wait( time); 00080 mode = 3; 00081 } 00082 void case3(){ 00083 motorBack(); 00084 motorDown(); 00085 //wait( time); 00086 mode = 4; 00087 } 00088 void case4(){ 00089 motorBack(); 00090 motorUp(); 00091 //wait( time); 00092 mode = 1; 00093 } 00094 00095 int main(){ 00096 /*led.write(0); 00097 00098 while( true){ 00099 if( Reseive.id == 1 ){ 00100 data 00101 } 00102 wait( 0.1); 00103 }*/ 00104 00105 while(1){ 00106 switch( mode){ 00107 case 0: 00108 case0(); 00109 break; 00110 case 1: 00111 case1(); 00112 break; 00113 case 2: 00114 case2(); 00115 break; 00116 case 3: 00117 case3(); 00118 break; 00119 case 4: 00120 case4(); 00121 break; 00122 } 00123 00124 if( switch1.read() == 0) 00125 led.write(0); 00126 else if( switch1.read() == 1) 00127 led.write(1); 00128 00129 wait( time); 00130 } 00131 }
Generated on Fri Jul 15 2022 10:38:46 by
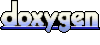