
Test, please delete
Embed:
(wiki syntax)
Show/hide line numbers
adi_sense_1000_sensor_types.h
Go to the documentation of this file.
00001 /* 00002 Copyright 2017 (c) Analog Devices, Inc. 00003 00004 All rights reserved. 00005 00006 Redistribution and use in source and binary forms, with or without 00007 modification, are permitted provided that the following conditions are met: 00008 - Redistributions of source code must retain the above copyright 00009 notice, this list of conditions and the following disclaimer. 00010 - Redistributions in binary form must reproduce the above copyright 00011 notice, this list of conditions and the following disclaimer in 00012 the documentation and/or other materials provided with the 00013 distribution. 00014 - Neither the name of Analog Devices, Inc. nor the names of its 00015 contributors may be used to endorse or promote products derived 00016 from this software without specific prior written permission. 00017 - The use of this software may or may not infringe the patent rights 00018 of one or more patent holders. This license does not release you 00019 from the requirement that you obtain separate licenses from these 00020 patent holders to use this software. 00021 - Use of the software either in source or binary form, must be run 00022 on or directly connected to an Analog Devices Inc. component. 00023 00024 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00025 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00026 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00027 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00028 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 00036 /*! 00037 ****************************************************************************** 00038 * @file: 00039 * @brief: Sensor type definitions for ADSNS1000. 00040 *----------------------------------------------------------------------------- 00041 */ 00042 00043 #ifndef __ADI_SENSE_1000_SENSOR_TYPES_H__ 00044 #define __ADI_SENSE_1000_SENSOR_TYPES_H__ 00045 00046 /*! @addtogroup ADSNS1000_Api 00047 * @{ 00048 */ 00049 00050 #ifdef __cplusplus 00051 extern "C" { 00052 #endif 00053 00054 /*! ADSNS1000 measurement channel identifiers */ 00055 typedef enum { 00056 ADI_SENSE_1000_CHANNEL_ID_NONE = -1, 00057 /*!< Used to indicate when no channel is selected (e.g. compensation channel) */ 00058 00059 ADI_SENSE_1000_CHANNEL_ID_CJC_0 = 0, 00060 /*!< Cold-Juction Compensation channel #0 */ 00061 ADI_SENSE_1000_CHANNEL_ID_CJC_1 , 00062 /*!< Cold-Juction Compensation channel #1 */ 00063 ADI_SENSE_1000_CHANNEL_ID_SENSOR_0 , 00064 /*!< Analog Sensor channel #0 */ 00065 ADI_SENSE_1000_CHANNEL_ID_SENSOR_1 , 00066 /*!< Analog Sensor channel #1 */ 00067 ADI_SENSE_1000_CHANNEL_ID_SENSOR_2 , 00068 /*!< Analog Sensor channel #2 */ 00069 ADI_SENSE_1000_CHANNEL_ID_SENSOR_3 , 00070 /*!< Analog Sensor channel #3 */ 00071 ADI_SENSE_1000_CHANNEL_ID_VOLTAGE_0 , 00072 /*!< Analog 0-10V Voltage Sensor channel #0 */ 00073 ADI_SENSE_1000_CHANNEL_ID_CURRENT_0 , 00074 /*!< Analog 4-20mA Current Sensor channel #0 */ 00075 ADI_SENSE_1000_CHANNEL_ID_I2C_0 , 00076 /*!< Digital I2C Sensor channel #0 */ 00077 ADI_SENSE_1000_CHANNEL_ID_I2C_1 , 00078 /*!< Digital I2C Sensor channel #1 */ 00079 ADI_SENSE_1000_CHANNEL_ID_SPI_0 , 00080 /*!< Digital SPI Sensor channel #0 */ 00081 ADI_SENSE_1000_CHANNEL_ID_SPI_1 , 00082 /*!< Digital SPI Sensor channel #1 */ 00083 ADI_SENSE_1000_CHANNEL_ID_SPI_2 , 00084 /*!< Digital SPI Sensor channel #2 */ 00085 ADI_SENSE_1000_CHANNEL_ID_UART , 00086 /*!< Digital UART Sensor channel #0 */ 00087 00088 ADI_SENSE_1000_MAX_CHANNELS , 00089 /*!< Maximum number of measurement channels on ADSNS1000 */ 00090 } ADI_SENSE_1000_CHANNEL_ID ; 00091 00092 /*! ADSNS1000 analog sensor type options 00093 * 00094 * Select the sensor type that is connected to an ADC analog measurement 00095 * channel. 00096 * 00097 * @note Some channels may only support a subset of the available sensor types 00098 * below. 00099 * 00100 * @note The sensor type name may include a classification suffix: 00101 * - _DEF_L1: pre-defined sensor using built-in linearisation data 00102 * - _DEF_L2: pre-defined sensor using user-supplied linearisation data 00103 * Where the suffix is absent, assume the _DEF_L1 classification above. 00104 */ 00105 typedef enum { 00106 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_T_DEF_L1 = 0, 00107 /*!< Standard T-type Thermocouple temperature sensor with default 00108 * linearisation and default configuration options 00109 * 00110 * @note For use with Analog Sensor channels only 00111 */ 00112 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_J_DEF_L1 = 1, 00113 /*!< Standard J-type Thermocouple temperature sensor with default 00114 * linearisation and default configuration options 00115 * 00116 * @note For use with Analog Sensor channels only 00117 */ 00118 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_K_DEF_L1 = 2, 00119 /*!< Standard K-type Thermocouple temperature sensor with default 00120 * linearisation and default configuration options 00121 * 00122 * @note For use with Analog Sensor channels only 00123 */ 00124 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_1_DEF_L2 = 8, 00125 /*!< Standard thermocouple temperature sensor with user-defined 00126 * linearisation and default configuration options 00127 * 00128 * @note For use with Analog Sensor channels only 00129 */ 00130 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_2_DEF_L2 = 9, 00131 /*!< Standard thermocouple temperature sensor with user-defined 00132 * linearisation and default configuration options 00133 * 00134 * @note For use with Analog Sensor channels only 00135 */ 00136 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_3_DEF_L2 = 10, 00137 /*!< Standard thermocouple temperature sensor with user-defined 00138 * linearisation and default configuration options 00139 * 00140 * @note For use with Analog Sensor channels only 00141 */ 00142 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_4_DEF_L2 = 11, 00143 /*!< Standard thermocouple temperature sensor with user-defined 00144 * linearisation and default configuration options 00145 * 00146 * @note For use with Analog Sensor channels only 00147 */ 00148 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_T_ADV_L1 = 16, 00149 /*!< T-type thermocouple temperature sensor with default linearisation and 00150 * advanced configuration options 00151 * 00152 * @note For use with Analog Sensor channels only 00153 */ 00154 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_J_ADV_L1 = 17, 00155 /*!< T-type thermocouple temperature sensor with default linearisation and 00156 * advanced configuration options 00157 * 00158 * @note For use with Analog Sensor channels only 00159 */ 00160 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_K_ADV_L1 = 18, 00161 /*!< T-type thermocouple temperature sensor with default linearisation and 00162 * advanced configuration options 00163 * 00164 * @note For use with Analog Sensor channels only 00165 */ 00166 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_1_ADV_L2 = 24, 00167 /*!< Thermocouple temperature sensor with user-defined 00168 * linearisation and advanced configuration options 00169 * 00170 * @note For use with Analog Sensor channels only 00171 */ 00172 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_2_ADV_L2 = 25, 00173 /*!< Thermocouple temperature sensor with user-defined 00174 * linearisation and advanced configuration options 00175 * 00176 * @note For use with Analog Sensor channels only 00177 */ 00178 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_3_ADV_L2 = 26, 00179 /*!< Thermocouple temperature sensor with user-defined 00180 * linearisation and advanced configuration options 00181 * 00182 * @note For use with Analog Sensor channels only 00183 */ 00184 ADI_SENSE_1000_ADC_SENSOR_THERMOCOUPLE_4_ADV_L2 = 27, 00185 /*!< Thermocouple temperature sensor with user-defined 00186 * linearisation and advanced configuration options 00187 * 00188 * @note For use with Analog Sensor channels only 00189 */ 00190 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_PT100_DEF_L1 = 32, 00191 /*!< Standard 2-wire PT100 RTD temperature sensor with default 00192 * linearisation and default configuration options 00193 * 00194 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00195 * only 00196 */ 00197 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_PT1000_DEF_L1 = 33, 00198 /*!< Standard 2-wire PT1000 RTD temperature sensor with default 00199 * linearisation and default configuration options 00200 * 00201 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00202 * only 00203 */ 00204 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_1_DEF_L2 = 40, 00205 /*!< 2-wire RTD temperature sensor with user-defined linearisation and 00206 * default configuration options 00207 * 00208 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00209 * only 00210 */ 00211 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_2_DEF_L2 = 41, 00212 /*!< 2-wire RTD temperature sensor with user-defined linearisation and 00213 * default configuration options 00214 * 00215 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00216 * only 00217 */ 00218 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_3_DEF_L2 = 42, 00219 /*!< 2-wire RTD temperature sensor with user-defined linearisation and 00220 * default configuration options 00221 * 00222 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00223 * only 00224 */ 00225 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_4_DEF_L2 = 43, 00226 /*!< 2-wire RTD temperature sensor with user-defined linearisation and 00227 * default configuration options 00228 * 00229 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00230 * only 00231 */ 00232 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_PT100_ADV_L1 = 48, 00233 /*!< Standard 2-wire PT100 RTD temperature sensor with default 00234 * linearisation and advanced configuration options 00235 * 00236 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00237 * only 00238 */ 00239 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_PT1000_ADV_L1 = 49, 00240 /*!< Standard 2-wire PT1000 RTD temperature sensor with default 00241 * linearisation and advanced configuration options 00242 * 00243 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00244 * only 00245 */ 00246 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_1_ADV_L2 = 56, 00247 /*!< 2-wire RTD temperature sensor with user-defined linearisation and 00248 * advanced configuration options 00249 * 00250 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00251 * only 00252 */ 00253 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_2_ADV_L2 = 57, 00254 /*!< 2-wire RTD temperature sensor with user-defined linearisation and 00255 * advanced configuration options 00256 * 00257 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00258 * only 00259 */ 00260 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_3_ADV_L2 = 58, 00261 /*!< 2-wire RTD temperature sensor with user-defined linearisation and 00262 * advanced configuration options 00263 * 00264 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00265 * only 00266 */ 00267 ADI_SENSE_1000_ADC_SENSOR_RTD_2WIRE_4_ADV_L2 = 59, 00268 /*!< 2-wire RTD temperature sensor with user-defined linearisation and 00269 * advanced configuration options 00270 * 00271 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00272 * only 00273 */ 00274 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_PT100_DEF_L1 = 64, 00275 /*!< Standard 3-wire PT100 RTD temperature sensor with default 00276 * linearisation and default configuration options 00277 * 00278 * @note For use with Analog Sensor channels only 00279 */ 00280 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_PT1000_DEF_L1 = 65, 00281 /*!< Standard 3-wire PT1000 RTD temperature sensor with default 00282 * linearisation and default configuration options 00283 * 00284 * @note For use with Analog Sensor channels only 00285 */ 00286 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_1_DEF_L2 = 72, 00287 /*!< 3-wire RTD temperature sensor with user-defined linearisation and 00288 * default configuration options 00289 * 00290 * @note For use with Analog Sensor channels only 00291 */ 00292 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_2_DEF_L2 = 73, 00293 /*!< 3-wire RTD temperature sensor with user-defined linearisation and 00294 * default configuration options 00295 * 00296 * @note For use with Analog Sensor channels only 00297 */ 00298 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_3_DEF_L2 = 74, 00299 /*!< 3-wire RTD temperature sensor with user-defined linearisation and 00300 * default configuration options 00301 * 00302 * @note For use with Analog Sensor channels only 00303 */ 00304 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_4_DEF_L2 = 75, 00305 /*!< 3-wire RTD temperature sensor with user-defined linearisation and 00306 * default configuration options 00307 * 00308 * @note For use with Analog Sensor channels only 00309 */ 00310 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_PT100_ADV_L1 = 80, 00311 /*!< Standard 3-wire PT100 RTD temperature sensor with default 00312 * linearisation and advanced configuration options 00313 * 00314 * @note For use with Analog Sensor channels only 00315 */ 00316 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_PT1000_ADV_L1 = 81, 00317 /*!< Standard 3-wire PT1000 RTD temperature sensor with default 00318 * linearisation and advanced configuration options 00319 * 00320 * @note For use with Analog Sensor channels only 00321 */ 00322 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_1_ADV_L2 = 88, 00323 /*!< 3-wire RTD temperature sensor with user-defined linearisation and 00324 * advanced configuration options 00325 * 00326 * @note For use with Analog Sensor channels only 00327 */ 00328 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_2_ADV_L2 = 89, 00329 /*!< 3-wire RTD temperature sensor with user-defined linearisation and 00330 * advanced configuration options 00331 * 00332 * @note For use with Analog Sensor channels only 00333 */ 00334 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_3_ADV_L2 = 90, 00335 /*!< 3-wire RTD temperature sensor with user-defined linearisation and 00336 * advanced configuration options 00337 * 00338 * @note For use with Analog Sensor channels only 00339 */ 00340 ADI_SENSE_1000_ADC_SENSOR_RTD_3WIRE_4_ADV_L2 = 91, 00341 /*!< 3-wire RTD temperature sensor with user-defined linearisation and 00342 * advanced configuration options 00343 * 00344 * @note For use with Analog Sensor channels only 00345 */ 00346 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_PT100_DEF_L1 = 96, 00347 /*!< Standard 4-wire PT100 RTD temperature sensor with default 00348 * linearisation and default configuration options 00349 * 00350 * @note For use with Analog Sensor channels only 00351 */ 00352 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_PT1000_DEF_L1 = 97, 00353 /*!< Standard 4-wire PT1000 RTD temperature sensor with default 00354 * linearisation and default configuration options 00355 * 00356 * @note For use with Analog Sensor channels only 00357 */ 00358 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_1_DEF_L2 = 104, 00359 /*!< 4-wire RTD temperature sensor with user-defined linearisation and 00360 * default configuration options 00361 * 00362 * @note For use with Analog Sensor channels only 00363 */ 00364 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_2_DEF_L2 = 105, 00365 /*!< 4-wire RTD temperature sensor with user-defined linearisation and 00366 * default configuration options 00367 * 00368 * @note For use with Analog Sensor channels only 00369 */ 00370 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_3_DEF_L2 = 106, 00371 /*!< 4-wire RTD temperature sensor with user-defined linearisation and 00372 * default configuration options 00373 * 00374 * @note For use with Analog Sensor channels only 00375 */ 00376 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_4_DEF_L2 = 107, 00377 /*!< 4-wire RTD temperature sensor with user-defined linearisation and 00378 * default configuration options 00379 * 00380 * @note For use with Analog Sensor channels only 00381 */ 00382 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_PT100_ADV_L1 = 112, 00383 /*!< Standard 4-wire PT100 RTD temperature sensor with default 00384 * linearisation and advanced configuration options 00385 * 00386 * @note For use with Analog Sensor channels only 00387 */ 00388 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_PT1000_ADV_L1 = 113, 00389 /*!< Standard 4-wire PT1000 RTD temperature sensor with default 00390 * linearisation and advanced configuration options 00391 * 00392 * @note For use with Analog Sensor channels only 00393 */ 00394 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_1_ADV_L2 = 120, 00395 /*!< 4-wire RTD temperature sensor with user-defined linearisation and 00396 * advanced configuration options 00397 * 00398 * @note For use with Analog Sensor channels only 00399 */ 00400 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_2_ADV_L2 = 121, 00401 /*!< 4-wire RTD temperature sensor with user-defined linearisation and 00402 * advanced configuration options 00403 * 00404 * @note For use with Analog Sensor channels only 00405 */ 00406 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_3_ADV_L2 = 122, 00407 /*!< 4-wire RTD temperature sensor with user-defined linearisation and 00408 * advanced configuration options 00409 * 00410 * @note For use with Analog Sensor channels only 00411 */ 00412 ADI_SENSE_1000_ADC_SENSOR_RTD_4WIRE_4_ADV_L2 = 123, 00413 /*!< 4-wire RTD temperature sensor with user-defined linearisation and 00414 * advanced configuration options 00415 * 00416 * @note For use with Analog Sensor channels only 00417 */ 00418 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_A_10K_DEF_L1 = 128, 00419 /*!< Standard 10kOhm NTC Thermistor temperature sensor with Steinhart–Hart 00420 * linearisation equation and default configuration options 00421 * 00422 * @note For use with Analog Sensor channels only 00423 */ 00424 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_B_10K_DEF_L1 = 129, 00425 /*!< Standard 10kOhm NTC Thermistor temperature sensor with Beta 00426 * linearisation equation and default configuration options 00427 * 00428 * @note For use with Analog Sensor channels only 00429 */ 00430 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_1_DEF_L2 = 136, 00431 /*!< Thermistor sensor with user-defined linearisation and 00432 * default configuration options 00433 * 00434 * @note For use with Analog Sensor channels only 00435 */ 00436 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_2_DEF_L2 = 137, 00437 /*!< Thermistor sensor with user-defined linearisation and 00438 * default configuration options 00439 * 00440 * @note For use with Analog Sensor channels only 00441 */ 00442 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_3_DEF_L2 = 138, 00443 /*!< Thermistor sensor with user-defined linearisation and 00444 * default configuration options 00445 * 00446 * @note For use with Analog Sensor channels only 00447 */ 00448 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_4_DEF_L2 = 139, 00449 /*!< Thermistor sensor with user-defined linearisation and 00450 * default configuration options 00451 * 00452 * @note For use with Analog Sensor channels only 00453 */ 00454 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_A_10K_ADV_L1 = 144, 00455 /*!< 10kOhm NTC Thermistor temperature sensor with Steinhart–Hart 00456 * linearisation equation and advanced configuration options 00457 * 00458 * @note For use with Analog Sensor channels only 00459 */ 00460 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_B_10K_ADV_L1 = 145, 00461 /*!< 10kOhm NTC Thermistor temperature sensor with Beta 00462 * linearisation equation and advanced configuration options 00463 * 00464 * @note For use with Analog Sensor channels only 00465 */ 00466 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_1_ADV_L2 = 152, 00467 /*!< Thermistor sensor with user-defined linearisation and 00468 * advanced configuration options 00469 * 00470 * @note For use with Analog Sensor channels only 00471 */ 00472 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_2_ADV_L2 = 153, 00473 /*!< Thermistor sensor with user-defined linearisation and 00474 * advanced configuration options 00475 * 00476 * @note For use with Analog Sensor channels only 00477 */ 00478 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_3_ADV_L2 = 154, 00479 /*!< Thermistor sensor with user-defined linearisation and 00480 * advanced configuration options 00481 * 00482 * @note For use with Analog Sensor channels only 00483 */ 00484 ADI_SENSE_1000_ADC_SENSOR_THERMISTOR_4_ADV_L2 = 155, 00485 /*!< Thermistor sensor with user-defined linearisation and 00486 * advanced configuration options 00487 * 00488 * @note For use with Analog Sensor channels only 00489 */ 00490 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_4WIRE_1_DEF_L2 = 168, 00491 /*!< Standard 4-wire Bridge Transducer sensor with user-defined 00492 * linearisation and default configuration options 00493 * 00494 * @note For use with Analog Sensor channels only 00495 * @note Bridge Excition Voltage must be selected as reference 00496 */ 00497 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_4WIRE_2_DEF_L2 = 169, 00498 /*!< Standard 4-wire Bridge Transducer sensor with user-defined 00499 * linearisation and default configuration options 00500 * 00501 * @note For use with Analog Sensor channels only 00502 * @note Bridge Excition Voltage must be selected as reference 00503 */ 00504 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_4WIRE_3_DEF_L2 = 170, 00505 /*!< Standard 4-wire Bridge Transducer sensor with user-defined 00506 * linearisation and default configuration options 00507 * 00508 * @note For use with Analog Sensor channels only 00509 * @note Bridge Excition Voltage must be selected as reference 00510 */ 00511 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_4WIRE_4_DEF_L2 = 171, 00512 /*!< Standard 4-wire Bridge Transducer sensor with user-defined 00513 * linearisation and default configuration options 00514 * 00515 * @note For use with Analog Sensor channels only 00516 * @note Bridge Excition Voltage must be selected as reference 00517 */ 00518 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_4WIRE_1_ADV_L2 = 184, 00519 /*!< Standard 4-wire Bridge Transducer sensor with user-defined 00520 * linearisation and advanced configuration options 00521 * 00522 * @note For use with Analog Sensor channels only 00523 * @note Bridge Excition Voltage must be selected as reference 00524 */ 00525 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_4WIRE_2_ADV_L2 = 185, 00526 /*!< Standard 4-wire Bridge Transducer sensor with user-defined 00527 * linearisation and advanced configuration options 00528 * 00529 * @note For use with Analog Sensor channels only 00530 * @note Bridge Excition Voltage must be selected as reference 00531 */ 00532 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_4WIRE_3_ADV_L2 = 186, 00533 /*!< Standard 4-wire Bridge Transducer sensor with user-defined 00534 * linearisation and advanced configuration options 00535 * 00536 * @note For use with Analog Sensor channels only 00537 * @note Bridge Excition Voltage must be selected as reference 00538 */ 00539 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_4WIRE_4_ADV_L2 = 187, 00540 /*!< Standard 4-wire Bridge Transducer sensor with user-defined 00541 * linearisation and advanced configuration options 00542 * 00543 * @note For use with Analog Sensor channels only 00544 * @note Bridge Excition Voltage must be selected as reference 00545 */ 00546 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_6WIRE_1_DEF_L2 = 200, 00547 /*!< Standard 6-wire Bridge Transducer sensor with user-defined 00548 * linearisation and default configuration options 00549 * 00550 * @note For use with Analog Sensor channels only 00551 * @note Bridge Excition Voltage must be selected as reference 00552 */ 00553 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_6WIRE_2_DEF_L2 = 201, 00554 /*!< Standard 6-wire Bridge Transducer sensor with user-defined 00555 * linearisation and default configuration options 00556 * 00557 * @note For use with Analog Sensor channels only 00558 * @note Bridge Excition Voltage must be selected as reference 00559 */ 00560 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_6WIRE_3_DEF_L2 = 202, 00561 /*!< Standard 6-wire Bridge Transducer sensor with user-defined 00562 * linearisation and default configuration options 00563 * 00564 * @note For use with Analog Sensor channels only 00565 * @note Bridge Excition Voltage must be selected as reference 00566 */ 00567 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_6WIRE_4_DEF_L2 = 203, 00568 /*!< Standard 6-wire Bridge Transducer sensor with user-defined 00569 * linearisation and default configuration options 00570 * 00571 * @note For use with Analog Sensor channels only 00572 * @note Bridge Excition Voltage must be selected as reference 00573 */ 00574 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_6WIRE_1_ADV_L2 = 216, 00575 /*!< Standard 6-wire Bridge Transducer sensor with user-defined 00576 * linearisation and advanced configuration options 00577 * 00578 * @note For use with Analog Sensor channels only 00579 * @note Bridge Excition Voltage must be selected as reference 00580 */ 00581 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_6WIRE_2_ADV_L2 = 217, 00582 /*!< Standard 6-wire Bridge Transducer sensor with user-defined 00583 * linearisation and advanced configuration options 00584 * 00585 * @note For use with Analog Sensor channels only 00586 * @note Bridge Excition Voltage must be selected as reference 00587 */ 00588 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_6WIRE_3_ADV_L2 = 218, 00589 /*!< Standard 6-wire Bridge Transducer sensor with user-defined 00590 * linearisation and advanced configuration options 00591 * 00592 * @note For use with Analog Sensor channels only 00593 * @note Bridge Excition Voltage must be selected as reference 00594 */ 00595 ADI_SENSE_1000_ADC_SENSOR_BRIDGE_6WIRE_4_ADV_L2 = 219, 00596 /*!< Standard 6-wire Bridge Transducer sensor with user-defined 00597 * linearisation and advanced configuration options 00598 * 00599 * @note For use with Analog Sensor channels only 00600 * @note Bridge Excition Voltage must be selected as reference 00601 */ 00602 ADI_SENSE_1000_ADC_SENSOR_DIODE_2C_TYPEA_DEF_L1 = 224, 00603 /*!< Standard Diode two current temperature sensor with default 00604 * linearisation equation and default configuration options 00605 * 00606 * @note For use with Analog Sensor channels only 00607 */ 00608 ADI_SENSE_1000_ADC_SENSOR_DIODE_3C_TYPEA_DEF_L1 = 225, 00609 /*!< Standard Diode three current temperature sensor with default 00610 * linearisation equation and default configuration options 00611 * 00612 * @note For use with Analog Sensor channels only 00613 */ 00614 ADI_SENSE_1000_ADC_SENSOR_DIODE_2C_1_DEF_L2 = 232, 00615 /*!< Standard Diode two current sensor with user-defined linearisation and 00616 * default configuration options 00617 * 00618 * @note For use with Analog Sensor channels only 00619 */ 00620 ADI_SENSE_1000_ADC_SENSOR_DIODE_3C_1_DEF_L2 = 233, 00621 /*!< Standard Diode three current sensor with user-defined linearisation and 00622 * default configuration options 00623 * 00624 * @note For use with Analog Sensor channels only 00625 */ 00626 ADI_SENSE_1000_ADC_SENSOR_DIODE_2C_TYPEA_ADV_L1 = 240, 00627 /*!< Standard Diode two current temperature sensor with default 00628 * linearisation equation and advanced configuration options 00629 * 00630 * @note For use with Analog Sensor channels only 00631 */ 00632 ADI_SENSE_1000_ADC_SENSOR_DIODE_3C_TYPEA_ADV_L1 = 241, 00633 /*!< Standard Diode three current sensor with default linearisation and 00634 * advanced configuration options 00635 * 00636 * @note For use with Analog Sensor channels only 00637 */ 00638 ADI_SENSE_1000_ADC_SENSOR_DIODE_2C_1_ADV_L2 = 248, 00639 /*!< Standard Diode two current sensor with user-defined linearisation and 00640 * advanced configuration options 00641 * 00642 * @note For use with Analog Sensor channels only 00643 */ 00644 ADI_SENSE_1000_ADC_SENSOR_DIODE_3C_1_ADV_L2 = 249, 00645 /*!< Standard Diode three current sensor with user-defined linearisation and 00646 * advanced configuration options 00647 * 00648 * @note For use with Analog Sensor channels only 00649 */ 00650 ADI_SENSE_1000_ADC_SENSOR_MICROPHONE_A_DEF_L1 = 256, 00651 /*!< Generic microphone sensor without external amplifier, and with 00652 * default linearisation and default configuration options 00653 * 00654 * @note For use with Analog Sensor channels only 00655 */ 00656 ADI_SENSE_1000_ADC_SENSOR_MICROPHONE_B_DEF_L1 = 257, 00657 /*!< Generic microphone sensor with external amplifier and bias, and with 00658 * default linearisation and default configuration options 00659 * 00660 * @note For use with Analog Sensor channels only 00661 */ 00662 ADI_SENSE_1000_ADC_SENSOR_MICROPHONE_1_DEF_L2 = 264, 00663 /*!< Generic microphone sensor without external amplifier, and with 00664 * user-defined linearisation and default configuration options 00665 * 00666 * @note For use with Analog Sensor channels only 00667 */ 00668 ADI_SENSE_1000_ADC_SENSOR_MICROPHONE_2_DEF_L2 = 265, 00669 /*!< Generic microphone sensor with external amplifier and bias, and with 00670 * user-defined linearisation and default configuration options 00671 * 00672 * @note For use with Analog Sensor channels only 00673 */ 00674 ADI_SENSE_1000_ADC_SENSOR_MICROPHONE_A_ADV_L1 = 272, 00675 /*!< Generic microphone sensor without external amplifier, and with 00676 * default linearisation and advanced configuration options 00677 * 00678 * @note For use with Analog Sensor channels only 00679 */ 00680 ADI_SENSE_1000_ADC_SENSOR_MICROPHONE_B_ADV_L1 = 273, 00681 /*!< Generic microphone sensor with external amplifier and bias, and with 00682 * default linearisation and advanced configuration options 00683 * 00684 * @note For use with Analog Sensor channels only 00685 */ 00686 ADI_SENSE_1000_ADC_SENSOR_MICROPHONE_1_ADV_L2 = 278, 00687 /*!< Generic microphone sensor without external amplifier, and with 00688 * user-defined linearisation and advanced configuration options 00689 * 00690 * @note For use with Analog Sensor channels only 00691 */ 00692 ADI_SENSE_1000_ADC_SENSOR_MICROPHONE_2_ADV_L2 = 279, 00693 /*!< Generic microphone sensor with external amplifier and bias, and with 00694 * user-defined linearisation and advanced configuration options 00695 * 00696 * @note For use with Analog Sensor channels only 00697 */ 00698 ADI_SENSE_1000_ADC_SENSOR_VOLTAGE = 512, 00699 /*!< Generic voltage sensor with no linearisation applied 00700 * 00701 * @note For use with Analog 0-10V Voltage Sensor channels only 00702 */ 00703 ADI_SENSE_1000_ADC_SENSOR_VOLTAGE_PRESSURE_A_DEF_L1 = 544, 00704 /*!< Honeywell Pressure voltage sensor (HSCMRNN1.6BAAA3) with default 00705 * linearisation and default configuration options 00706 * 00707 * @note For use with Analog 0-10V Voltage Sensor channels only 00708 */ 00709 ADI_SENSE_1000_ADC_SENSOR_VOLTAGE_PRESSURE_B_DEF_L1 = 545, 00710 /*!< Amphenol Pressure voltage sensor (NPA-300B-015A) with default 00711 * linearisation and default configuration options 00712 * 00713 * @note For use with Analog 0-10V Voltage Sensor channels only 00714 */ 00715 ADI_SENSE_1000_ADC_SENSOR_VOLTAGE_PRESSURE_1_DEF_L2 = 552, 00716 /*!< Generic pressure voltage sensor with user-defined 00717 * linearisation and default configuration options 00718 * 00719 * @note For use with Analog 0-10V Voltage Sensor channels only 00720 */ 00721 ADI_SENSE_1000_ADC_SENSOR_VOLTAGE_PRESSURE_2_DEF_L2 = 553, 00722 /*!< Generic pressure voltage sensor with user-defined 00723 * linearisation and advanced configuration options 00724 * 00725 * @note For use with Analog 0-10V Voltage Sensor channels only 00726 */ 00727 ADI_SENSE_1000_ADC_SENSOR_VOLTAGE_PRESSURE_A_ADV_L1 = 560, 00728 /*!< Honeywell Pressure voltage sensor (HSCMRNN1.6BAAA3) with default 00729 * linearisation and advanced configuration options 00730 * 00731 * @note For use with Analog 0-10V Voltage Sensor channels only 00732 */ 00733 ADI_SENSE_1000_ADC_SENSOR_VOLTAGE_PRESSURE_B_ADV_L1 = 561, 00734 /*!< Amphenol Pressure voltage sensor (NPA-300B-015A) with default 00735 * linearisation and advanced configuration options 00736 * 00737 * @note For use with Analog 0-10V Voltage Sensor channels only 00738 */ 00739 ADI_SENSE_1000_ADC_SENSOR_VOLTAGE_PRESSURE_1_ADV_L2 = 568, 00740 /*!< Generic pressure voltage sensor with user-defined 00741 * linearisation and advanced configuration options 00742 * 00743 * @note For use with Analog 0-10V Voltage Sensor channels only 00744 */ 00745 ADI_SENSE_1000_ADC_SENSOR_VOLTAGE_PRESSURE_2_ADV_L2 = 569, 00746 /*!< Generic pressure voltage sensor with user-defined 00747 * linearisation and default configuration options 00748 * 00749 * @note For use with Analog 0-10V Voltage Sensor channels only 00750 */ 00751 ADI_SENSE_1000_ADC_SENSOR_CURRENT = 768, 00752 /*!< Generic current sensor with no linearisation applied 00753 * 00754 * @note For use with Analog 4-20mA Current Sensor channels only 00755 */ 00756 ADI_SENSE_1000_ADC_SENSOR_CURRENT_PRESSURE_A_DEF_L1 = 800, 00757 /*!< Honeywell Pressure current sensor (PX2CN2XX100PACH) with default 00758 * linearisation and default configuration options 00759 * 00760 * @note For use with Analog 4-20mA Current Sensor channels only 00761 */ 00762 ADI_SENSE_1000_ADC_SENSOR_CURRENT_PRESSURE_1_DEF_L2 = 808, 00763 /*!< Generic pressure current sensor with user-defined 00764 * linearisation and default configuration options 00765 * 00766 * @note For use with Analog 4-20mA Current Sensor channels only 00767 */ 00768 ADI_SENSE_1000_ADC_SENSOR_CURRENT_PRESSURE_2_DEF_L2 = 809, 00769 /*!< Generic pressure current sensor with user-defined 00770 * linearisation and default configuration options 00771 * 00772 * @note For use with Analog 4-20mA Current Sensor channels only 00773 */ 00774 ADI_SENSE_1000_ADC_SENSOR_CURRENT_PRESSURE_A_ADV_L1 = 816, 00775 /*!< Honeywell Pressure current sensor (PX2CN2XX100PACH) with default 00776 * linearisation and advanced configuration options 00777 * 00778 * @note For use with Analog 4-20mA Current Sensor channels only 00779 */ 00780 ADI_SENSE_1000_ADC_SENSOR_CURRENT_PRESSURE_1_ADV_L2 = 824, 00781 /*!< Generic pressure current sensor with user-defined 00782 * linearisation and advanced configuration options 00783 * 00784 * @note For use with Analog 4-20mA Current Sensor channels only 00785 */ 00786 ADI_SENSE_1000_ADC_SENSOR_CURRENT_PRESSURE_2_ADV_L2 = 825, 00787 /*!< Generic pressure current sensor with user-defined 00788 * linearisation and advanced configuration options 00789 * 00790 * @note For use with Analog 4-20mA Current Sensor channels only 00791 */ 00792 } ADI_SENSE_1000_ADC_SENSOR_TYPE ; 00793 00794 /*! ADSNS1000 I2C digital sensor type options 00795 * 00796 * Select the sensor type that is connected to an I2C digital measurement 00797 * channel. 00798 * 00799 * @note These are pre-defined sensors using built-in linearisation data 00800 */ 00801 typedef enum { 00802 ADI_SENSE_1000_I2C_SENSOR_HUMIDITY_A_DEF_L1 = 2112, 00803 /*!< Honeywell HiH9000-series humidity sensor with default linearisation 00804 * and default configuration options 00805 * 00806 * @note For use with I2C Digital Sensor channels only 00807 */ 00808 ADI_SENSE_1000_I2C_SENSOR_HUMIDITY_B_DEF_L1 = 2113, 00809 /*!< Sensirion SHT35-DIS-B humidity sensor with default linearisation 00810 * and default configuration options 00811 * 00812 * @note For use with I2C Digital Sensor channels only 00813 */ 00814 ADI_SENSE_1000_I2C_SENSOR_HUMIDITY_A_DEF_L2 = 2120, 00815 /*!< Honeywell HiH9000-series humidity sensor with user-defined linearisation 00816 * and default configuration options 00817 * 00818 * @note For use with I2C Digital Sensor channels only 00819 */ 00820 ADI_SENSE_1000_I2C_SENSOR_HUMIDITY_B_DEF_L2 = 2121, 00821 /*!< Sensirion SHT35-DIS-B humidity sensor with user-defined linearisation 00822 * and default configuration options 00823 * 00824 * @note For use with I2C Digital Sensor channels only 00825 */ 00826 ADI_SENSE_1000_I2C_SENSOR_HUMIDITY_A_ADV_L1 = 2128, 00827 /*!< Honeywell HiH9000-series humidity sensor with default linearisation 00828 * and advanced configuration options 00829 * 00830 * @note For use with I2C Digital Sensor channels only 00831 */ 00832 ADI_SENSE_1000_I2C_SENSOR_HUMIDITY_B_ADV_L1 = 2129, 00833 /*!< Sensirion SHT35-DIS-B humidity sensor with default linearisation 00834 * and advanced configuration options 00835 * 00836 * @note For use with I2C Digital Sensor channels only 00837 */ 00838 ADI_SENSE_1000_I2C_SENSOR_HUMIDITY_A_ADV_L2 = 2136, 00839 /*!< Honeywell HiH9000-series humidity sensor with user-defined 00840 * linearisation and advanced configuration options 00841 * 00842 * @note For use with I2C Digital Sensor channels only 00843 */ 00844 ADI_SENSE_1000_I2C_SENSOR_HUMIDITY_B_ADV_L2 = 2137, 00845 /*!< Sensirion SHT35-DIS-B humidity sensor with user-defined linearisation 00846 * and advanced configuration options 00847 * 00848 * @note For use with I2C Digital Sensor channels only 00849 */ 00850 ADI_SENSE_1000_I2C_SENSOR_AMBIENTLIGHT_A_DEF_L1 = 2176, 00851 /*!< ON-Semiconductor NOA1305 ambient light sensor with default 00852 * linearisation and default configuration options 00853 * 00854 * @note For use with I2C Digital Sensor channels only 00855 */ 00856 ADI_SENSE_1000_I2C_SENSOR_AMBIENTLIGHT_A_DEF_L2 = 2184, 00857 /*!< ON-Semiconductor NOA1305 ambient light sensor with user-defined 00858 * linearisation and default configuration options 00859 * 00860 * @note For use with I2C Digital Sensor channels only 00861 */ 00862 ADI_SENSE_1000_I2C_SENSOR_AMBIENTLIGHT_A_ADV_L1 = 2192, 00863 /*!< ON-Semiconductor NOA1305 ambient light sensor with default 00864 * linearisation and advanced configuration options 00865 * 00866 * @note For use with I2C Digital Sensor channels only 00867 */ 00868 ADI_SENSE_1000_I2C_SENSOR_AMBIENTLIGHT_A_ADV_L2 = 2200, 00869 /*!< ON-Semiconductor NOA1305 ambient light sensor with user-defined 00870 * linearisation and advanced configuration options 00871 * 00872 * @note For use with I2C Digital Sensor channels only 00873 */ 00874 } ADI_SENSE_1000_I2C_SENSOR_TYPE ; 00875 00876 /*! ADSNS1000 SPI digital sensor type options 00877 * 00878 * Select the sensor type that is connected to an SPI digital measurement 00879 * channel. 00880 * 00881 * @note These are pre-defined sensors using built-in linearisation data 00882 */ 00883 typedef enum { 00884 ADI_SENSE_1000_SPI_SENSOR_PRESSURE_A_DEF_L1 = 3072, 00885 /*!< Honeywell HSCDRNN1.6BASA3 pressure sensor with default linearisation 00886 * and default configuration options 00887 * 00888 * @note For use with SPI Digital Sensor channels only 00889 */ 00890 ADI_SENSE_1000_SPI_SENSOR_PRESSURE_A_DEF_L2 = 3080, 00891 /*!< Honeywell HSCDRNN1.6BASA3 pressure sensor with user-defined 00892 * linearisation and default configuration options 00893 * 00894 * @note For use with SPI Digital Sensor channels only 00895 */ 00896 ADI_SENSE_1000_SPI_SENSOR_PRESSURE_A_ADV_L1 = 3088, 00897 /*!< Honeywell HSCDRNN1.6BASA3 pressure sensor with default linearisation 00898 * and advanced configuration options 00899 * 00900 * @note For use with SPI Digital Sensor channels only 00901 */ 00902 ADI_SENSE_1000_SPI_SENSOR_PRESSURE_A_ADV_L2 = 3096, 00903 /*!< Honeywell HSCDRNN1.6BASA3 pressure sensor with user-defined 00904 * linearisation and advanced configuration options 00905 * 00906 * @note For use with SPI Digital Sensor channels only 00907 */ 00908 ADI_SENSE_1000_SPI_SENSOR_ACCELEROMETER_A_DEF_L1 = 3200, 00909 /*!< Analog Devices ADxL362 3-axis accelerometer sensor with default 00910 * linearisation and default configuration options(*) 00911 * 00912 * @note For use with SPI Digital Sensor channels only 00913 * 00914 * @note This sensor requires the use of 3 SPI Digital Sensor channels, with 00915 * the sensor measurements from the X/Y/Z axes each output on a 00916 * seperate dedicated channel (SPI#0/SPI#1/SPI#2, respectively) 00917 */ 00918 ADI_SENSE_1000_SPI_SENSOR_ACCELEROMETER_B_DEF_L1 = 3201, 00919 /*!< Analog Devices ADxL355 3-axis accelerometer sensor with default 00920 * linearisation and default configuration options(*) 00921 * 00922 * @note For use with SPI Digital Sensor channels only 00923 * 00924 * @note This sensor requires the use of 3 SPI Digital Sensor channels, with 00925 * the sensor measurements from the X/Y/Z axes each output on a 00926 * seperate dedicated channel (SPI#0/SPI#1/SPI#2, respectively) 00927 */ 00928 ADI_SENSE_1000_SPI_SENSOR_ACCELEROMETER_A_DEF_L2 = 3208, 00929 /*!< Analog Devices ADxL362 3-axis accelerometer sensor with user-defined 00930 * linearisation and default configuration options(*) 00931 * 00932 * @note For use with SPI Digital Sensor channels only 00933 * 00934 * @note This sensor requires the use of 3 SPI Digital Sensor channels, with 00935 * the sensor measurements from the X/Y/Z axes each output on a 00936 * seperate dedicated channel (SPI#0/SPI#1/SPI#2, respectively) 00937 */ 00938 ADI_SENSE_1000_SPI_SENSOR_ACCELEROMETER_B_DEF_L2 = 3209, 00939 /*!< Analog Devices ADxL355 3-axis accelerometer sensor with user-defined 00940 * linearisation and default configuration options(*) 00941 * 00942 * @note For use with SPI Digital Sensor channels only 00943 * 00944 * @note This sensor requires the use of 3 SPI Digital Sensor channels, with 00945 * the sensor measurements from the X/Y/Z axes each output on a 00946 * seperate dedicated channel (SPI#0/SPI#1/SPI#2, respectively) 00947 */ 00948 ADI_SENSE_1000_SPI_SENSOR_ACCELEROMETER_A_ADV_L1 = 3216, 00949 /*!< Analog Devices ADxL362 3-axis accelerometer sensor with default 00950 * linearisation and advanced configuration options(*) 00951 * 00952 * @note For use with SPI Digital Sensor channels only 00953 * 00954 * @note This sensor requires the use of 3 SPI Digital Sensor channels, with 00955 * the sensor measurements from the X/Y/Z axes each output on a 00956 * seperate dedicated channel (SPI#0/SPI#1/SPI#2, respectively) 00957 */ 00958 ADI_SENSE_1000_SPI_SENSOR_ACCELEROMETER_B_ADV_L1 = 3217, 00959 /*!< Analog Devices ADxL355 3-axis accelerometer sensor with default 00960 * linearisation and advanced configuration options(*) 00961 * 00962 * @note For use with SPI Digital Sensor channels only 00963 * 00964 * @note This sensor requires the use of 3 SPI Digital Sensor channels, with 00965 * the sensor measurements from the X/Y/Z axes each output on a 00966 * seperate dedicated channel (SPI#0/SPI#1/SPI#2, respectively) 00967 */ 00968 ADI_SENSE_1000_SPI_SENSOR_ACCELEROMETER_A_ADV_L2 = 3224, 00969 /*!< Analog Devices ADxL362 3-axis accelerometer sensor with user-defined 00970 * linearisation and advanced configuration options(*) 00971 * 00972 * @note For use with SPI Digital Sensor channels only 00973 * 00974 * @note This sensor requires the use of 3 SPI Digital Sensor channels, with 00975 * the sensor measurements from the X/Y/Z axes each output on a 00976 * seperate dedicated channel (SPI#0/SPI#1/SPI#2, respectively) 00977 */ 00978 ADI_SENSE_1000_SPI_SENSOR_ACCELEROMETER_B_ADV_L2 = 3225, 00979 /*!< Analog Devices ADxL355 3-axis accelerometer sensor with user-defined 00980 * linearisation and advanced configuration options(*) 00981 * 00982 * @note For use with SPI Digital Sensor channels only 00983 * 00984 * @note This sensor requires the use of 3 SPI Digital Sensor channels, with 00985 * the sensor measurements from the X/Y/Z axes each output on a 00986 * seperate dedicated channel (SPI#0/SPI#1/SPI#2, respectively) 00987 */ 00988 } ADI_SENSE_1000_SPI_SENSOR_TYPE ; 00989 00990 /*! ADSNS1000 UART digital sensor type options 00991 * 00992 * Select the sensor type that is connected to an UART digital measurement 00993 * channel. 00994 * 00995 * @note These are pre-defined sensors using built-in linearisation data 00996 */ 00997 typedef enum { 00998 ADI_SENSE_1000_UART_SENSOR_UART_CO2_A_DEF_L1 = 3584, 00999 /*!< COZIR AMB 2,000 ppm CO2 Sensor with default linearisation 01000 * and default configuration options 01001 * 01002 * @note For use with UART Digital Sensor channels only 01003 */ 01004 ADI_SENSE_1000_UART_SENSOR_UART_CO2_B_DEF_L1 = 3585, 01005 /*!< COZIR LP Miniature 5,000ppm CO2 Sensor with default linearisation 01006 * and default configuration options 01007 * 01008 * @note For use with UART Digital Sensor channels only 01009 */ 01010 ADI_SENSE_1000_UART_SENSOR_UART_CO2_A_DEF_L2 = 3592, 01011 /*!< COZIR AMB 2,000 ppm CO2 Sensor with user-defined linearisation 01012 * and default configuration options 01013 * 01014 * @note For use with UART Digital Sensor channels only 01015 */ 01016 ADI_SENSE_1000_UART_SENSOR_UART_CO2_B_DEF_L2 = 3593, 01017 /*!< COZIR LP Miniature 5,000ppm CO2 Sensor with user-defined linearisation 01018 * and default configuration options 01019 * 01020 * @note For use with UART Digital Sensor channels only 01021 */ 01022 ADI_SENSE_1000_UART_SENSOR_UART_CO2_A_ADV_L1 = 3600, 01023 /*!< COZIR AMB 2,000 ppm CO2 Sensor with default linearisation 01024 * and advanced configuration options 01025 * 01026 * @note For use with UART Digital Sensor channels only 01027 */ 01028 ADI_SENSE_1000_UART_SENSOR_UART_CO2_B_ADV_L1 = 3601, 01029 /*!< COZIR LP Miniature 5,000ppm CO2 Sensor with default linearisation 01030 * and advanced configuration options 01031 * 01032 * @note For use with UART Digital Sensor channels only 01033 */ 01034 ADI_SENSE_1000_UART_SENSOR_UART_CO2_A_ADV_L2 = 3608, 01035 /*!< COZIR AMB 2,000 ppm CO2 Sensor with user-defined linearisation 01036 * and advanced configuration options 01037 * 01038 * @note For use with UART Digital Sensor channels only 01039 */ 01040 ADI_SENSE_1000_UART_SENSOR_UART_CO2_B_ADV_L2 = 3609, 01041 /*!< COZIR LP Miniature 5,000ppm CO2 Sensor with user-defined linearisation 01042 * and advanced configuration options 01043 * 01044 * @note For use with UART Digital Sensor channels only 01045 */ 01046 } ADI_SENSE_1000_UART_SENSOR_TYPE ; 01047 01048 #ifdef __cplusplus 01049 } 01050 #endif 01051 01052 /*! 01053 * @} 01054 */ 01055 01056 #endif /* __ADI_SENSE_1000_SENSOR_TYPES_H__ */
Generated on Tue Jul 12 2022 18:59:24 by
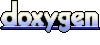