
supprimer les warnings
Dependencies: mbed projet_embarque WakeUp SigfoxToiture DHT DS1820
main.cpp
00001 #include"mbed.h" 00002 #include"DS1820.h" 00003 #include"DHT.h" 00004 #include "TCS34725.h" 00005 #include "Sigfox.h" 00006 #include "WakeUp.h" 00007 #include <math.h> 00008 00009 #define GROVE_MOIST_MIN 0 00010 #define GROVE_MOIST_MAX 0.55 00011 #define LOG_RANGE 5.0f 00012 00013 float Temp_Sol; 00014 float Temp_Air; 00015 float Humi_Air; 00016 float Humi_Sol; 00017 float Lumiere; 00018 uint16_t r; 00019 uint16_t g; 00020 uint16_t b; 00021 uint16_t c; 00022 00023 DHT dht22(D6,DHT22);//pin,type 00024 DS1820 ds1820(A0);//pin 00025 TCS34725 tcs34725(D4,D5); //I2C sda and scl 00026 AnalogIn humigrove(A1); 00027 AnalogIn lumiere(A5); 00028 AnalogIn bat(A4); 00029 DigitalOut ledTest(LED1); 00030 DigitalOut mos(D9); 00031 00032 void ReadTempHumiAir(){ 00033 int err=1; 00034 while(err!=0){ 00035 err=dht22.readData(); 00036 //ledTest = 1; 00037 wait(1); 00038 } 00039 if(err==0){ 00040 Temp_Air= dht22.ReadTemperature(CELCIUS); 00041 Humi_Air=dht22.ReadHumidity(); 00042 //ledTest = 0; 00043 } 00044 00045 } 00046 00047 void ReadTempSol(){ 00048 if (ds1820.begin()) { 00049 ds1820.startConversion(); // start temperature conversion from analog to digital 00050 wait(1); // let DS1820 complete the temperature conversion 00051 Temp_Sol = ds1820.read(); 00052 } 00053 } 00054 00055 void ReadRGBC(){ 00056 if(tcs34725.init(TCS34725_INTEGRATIONTIME_101MS, TCS34725_GAIN_60X)){ 00057 tcs34725.getColor(r,g,b,c); 00058 r/=256; 00059 g/=256; 00060 b/=256; 00061 c/=256; 00062 } 00063 } 00064 00065 void ReadHumiSol(){ 00066 float grove_moist=humigrove.read(); 00067 Humi_Sol= ((grove_moist-GROVE_MOIST_MIN) / (GROVE_MOIST_MAX - GROVE_MOIST_MIN)) * 100; 00068 } 00069 00070 void ReadLumi(){ 00071 float raw=lumiere.read(); 00072 float loglux=raw*LOG_RANGE; 00073 Lumiere=(float)(pow(10,loglux)*255.0f)/10000; 00074 //Lumiere=loglux; 00075 } 00076 00077 int main(){ 00078 //Serial at(D1,D0); 00079 Sigfox sigfox(D1,D0); 00080 /*ledTest = 1; 00081 wait(20); 00082 ledTest = 0; 00083 wait(5);*/ 00084 WakeUp::calibrate(); 00085 //wait(30); 00086 00087 while(1){ 00088 WakeUp::set(120); 00089 mos = 0; 00090 sigfox.wake(); 00091 wait(2); 00092 ReadTempHumiAir(); 00093 ReadTempSol(); 00094 ReadRGBC(); 00095 ReadHumiSol(); 00096 wait(1); 00097 ReadLumi(); 00098 wait(1); 00099 printf("R:%d, G:%d, B:%d\n\r", r, g, b); 00100 sigfox.send((s16)(Temp_Air*10),(u16)(Humi_Air*10),(s16)(Temp_Sol*10),(u16)(Humi_Sol*10), 00101 (u8)Lumiere,(u8)r,(u8)g,(u8)b, (u8)((float)bat*255.0f)); 00102 wait(10); 00103 mos = 1; 00104 //wait(10); 00105 sigfox.sleep(); 00106 deepsleep(); 00107 } 00108 }
Generated on Sun Jul 17 2022 16:18:37 by
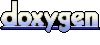