
r1.0
Dependencies: DHT libmDot mbed-rtos mbed
Fork of mDot_Connect_IoTClub_one_wire by
main.cpp
00001 #include "mbed.h" 00002 #include "mDot.h" 00003 #include "MTSLog.h" 00004 #include <string> 00005 #include <vector> 00006 #include <algorithm> 00007 00008 00009 const char TURNON[] = "ON"; 00010 const char TURNOFF[] = "OFF"; 00011 00012 bool cmpStr(char* str1, const char* str2, int len) 00013 { 00014 int k; 00015 for (k=0; k<len; k++){ 00016 if (str1[k] != str2[k]) return false; 00017 } 00018 return true; 00019 } 00020 00021 DigitalOut Alarm(PB_0); 00022 00023 //defined for mDot SVB debug, comment it if applying for whole system 00024 //#define NO_MULTITECH_GATEWAY 00025 00026 // these options must match the settings on your Conduit 00027 // uncomment the following lines and edit their values to match your configuration 00028 static std::string config_network_name = "chinaiot"; 00029 static std::string config_network_pass = "password"; 00030 static uint8_t config_frequency_sub_band = 2; 00031 00032 int main() { 00033 int32_t ret; 00034 mDot* dot; 00035 std::vector<uint8_t> sendData, recvData; 00036 std::vector<uint8_t> sendData1,sendData2, sendData3, sendData4; 00037 char recvBuf[11]; 00038 int send_failed; 00039 int i; 00040 //bool alarm_on; 00041 std::string data_str1 = "REQ1"; 00042 std::string data_str2 = "REQ2"; 00043 std::string data_str3 = "REQ3"; 00044 std::string data_str4 = "REQ4"; 00045 bool a1, a2, a3, a4; 00046 00047 00048 // get a mDot handle 00049 dot = mDot::getInstance(); 00050 00051 // print library version information 00052 logInfo("version: %s", dot->getId().c_str()); 00053 00054 //******************************************* 00055 // configuration 00056 //******************************************* 00057 // reset to default config so we know what state we're in 00058 dot->resetConfig(); 00059 00060 dot->setLogLevel(mts::MTSLog::INFO_LEVEL); 00061 00062 // set up the mDot with our network information: frequency sub band, network name, and network password 00063 // these can all be saved in NVM so they don't need to be set every time - see mDot::saveConfig() 00064 00065 // frequency sub band is only applicable in the 915 (US) frequency band 00066 // if using a MultiTech Conduit gateway, use the same sub band as your Conduit (1-8) - the mDot will use the 8 channels in that sub band 00067 // if using a gateway that supports all 64 channels, use sub band 0 - the mDot will use all 64 channels 00068 logInfo("setting frequency sub band"); 00069 if ((ret = dot->setFrequencySubBand(config_frequency_sub_band)) != mDot::MDOT_OK) { 00070 logError("failed to set frequency sub band %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00071 } 00072 00073 logInfo("setting network name"); 00074 if ((ret = dot->setNetworkName(config_network_name)) != mDot::MDOT_OK) { 00075 logError("failed to set network name %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00076 } 00077 00078 logInfo("setting network password"); 00079 if ((ret = dot->setNetworkPassphrase(config_network_pass)) != mDot::MDOT_OK) { 00080 logError("failed to set network password %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00081 } 00082 00083 // a higher spreading factor allows for longer range but lower throughput 00084 // in the 915 (US) frequency band, spreading factors 7 - 10 are available 00085 // in the 868 (EU) frequency band, spreading factors 7 - 12 are available 00086 logInfo("setting TX spreading factor"); 00087 if ((ret = dot->setTxDataRate(mDot::SF_10)) != mDot::MDOT_OK) { 00088 logError("failed to set TX datarate %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00089 } 00090 00091 // request receive confirmation of packets from the gateway 00092 logInfo("enabling ACKs"); 00093 if ((ret = dot->setAck(1)) != mDot::MDOT_OK) { 00094 logError("failed to enable ACKs %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00095 } 00096 00097 // save this configuration to the mDot's NVM 00098 logInfo("saving config"); 00099 if (! dot->saveConfig()) { 00100 logError("failed to save configuration"); 00101 } 00102 //******************************************* 00103 // end of configuration 00104 //******************************************* 00105 00106 // attempt to join the network 00107 logInfo("joining network"); 00108 while ((ret = dot->joinNetwork()) != mDot::MDOT_OK) { 00109 logError("failed to join network %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00110 // in the 868 (EU) frequency band, we need to wait until another channel is available before transmitting again 00111 osDelay(std::max((uint32_t)1000, (uint32_t)dot->getNextTxMs())); 00112 } 00113 00114 // format data for sending to the gateway 00115 for (std::string::iterator it = data_str1.begin(); it != data_str1.end(); it++) 00116 sendData1.push_back((uint8_t) *it); 00117 00118 // format data for sending to the gateway 00119 for (std::string::iterator it = data_str2.begin(); it != data_str2.end(); it++) 00120 sendData2.push_back((uint8_t) *it); 00121 00122 // format data for sending to the gateway 00123 for (std::string::iterator it = data_str3.begin(); it != data_str3.end(); it++) 00124 sendData3.push_back((uint8_t) *it); 00125 00126 // format data for sending to the gateway 00127 for (std::string::iterator it = data_str4.begin(); it != data_str4.end(); it++) 00128 sendData4.push_back((uint8_t) *it); 00129 00130 send_failed = 0; 00131 while (true) { 00132 // send the data to the gateway 00133 if ((ret = dot->send(sendData1)) != mDot::MDOT_OK) { 00134 send_failed++; 00135 logError("failed to send", ret, mDot::getReturnCodeString(ret).c_str()); 00136 osDelay(std::max((uint32_t)5000, (uint32_t)dot->getNextTxMs())); 00137 } else { 00138 send_failed=0; 00139 logInfo("successfully sent data to gateway"); 00140 for(i=0;i<11;i++)recvBuf[i]=0; //clear recv buffer 00141 recvData.clear(); //clear recv data 00142 if ((ret = dot->recv(recvData)) != mDot::MDOT_OK) { 00143 logError("failed to recv: [%d][%s]", ret, mDot::getReturnCodeString(ret).c_str()); 00144 } else { 00145 //logInfo("datasize = %d", recvData.size()); 00146 for(i=0; i< recvData.size(); i++ ) 00147 recvBuf[i] = recvData[i]; 00148 //logInfo("RECV:%s", recvBuf); 00149 if(cmpStr(recvBuf, TURNON, 2)){ 00150 a1 = true; 00151 logInfo("A1 is on!"); 00152 } 00153 if(cmpStr(recvBuf, TURNOFF, 3)){ 00154 a1 = false; 00155 logInfo("A1 is off!"); 00156 } 00157 } 00158 } 00159 00160 osDelay(std::max((uint32_t)5000, (uint32_t)dot->getNextTxMs())); 00161 00162 // send the data to the gateway 00163 if ((ret = dot->send(sendData2)) != mDot::MDOT_OK) { 00164 send_failed++; 00165 logError("failed to send", ret, mDot::getReturnCodeString(ret).c_str()); 00166 osDelay(std::max((uint32_t)5000, (uint32_t)dot->getNextTxMs())); 00167 } else { 00168 send_failed=0; 00169 logInfo("successfully sent data to gateway"); 00170 for(i=0;i<11;i++)recvBuf[i]=0; //clear recv buffer 00171 recvData.clear(); //clear recv data 00172 if ((ret = dot->recv(recvData)) != mDot::MDOT_OK) { 00173 logError("failed to recv: [%d][%s]", ret, mDot::getReturnCodeString(ret).c_str()); 00174 } else { 00175 //logInfo("datasize = %d", recvData.size()); 00176 for(i=0; i< recvData.size(); i++ ) 00177 recvBuf[i] = recvData[i]; 00178 //logInfo("RECV:%s", recvBuf); 00179 if(cmpStr(recvBuf, TURNON, 2)){ 00180 a2 = true; 00181 logInfo("A2 is on!"); 00182 } 00183 if(cmpStr(recvBuf, TURNOFF, 3)){ 00184 a2 = false; 00185 logInfo("A2 is off!"); 00186 } 00187 } 00188 } 00189 00190 osDelay(std::max((uint32_t)5000, (uint32_t)dot->getNextTxMs())); 00191 00192 // send the data to the gateway 00193 if ((ret = dot->send(sendData3)) != mDot::MDOT_OK) { 00194 send_failed++; 00195 logError("failed to send", ret, mDot::getReturnCodeString(ret).c_str()); 00196 osDelay(std::max((uint32_t)5000, (uint32_t)dot->getNextTxMs())); 00197 } else { 00198 send_failed=0; 00199 logInfo("successfully sent data to gateway"); 00200 for(i=0;i<11;i++)recvBuf[i]=0; //clear recv buffer 00201 recvData.clear(); //clear recv data 00202 if ((ret = dot->recv(recvData)) != mDot::MDOT_OK) { 00203 logError("failed to recv: [%d][%s]", ret, mDot::getReturnCodeString(ret).c_str()); 00204 } else { 00205 //logInfo("datasize = %d", recvData.size()); 00206 for(i=0; i< recvData.size(); i++ ) 00207 recvBuf[i] = recvData[i]; 00208 //logInfo("RECV:%s", recvBuf); 00209 if(cmpStr(recvBuf, TURNON, 2)){ 00210 a3 = true; 00211 logInfo("A3 is on!"); 00212 } 00213 if(cmpStr(recvBuf, TURNOFF, 3)){ 00214 a3 = false; 00215 logInfo("A3 is off!"); 00216 } 00217 } 00218 } 00219 00220 osDelay(std::max((uint32_t)5000, (uint32_t)dot->getNextTxMs())); 00221 00222 // send the data to the gateway 00223 if ((ret = dot->send(sendData4)) != mDot::MDOT_OK) { 00224 send_failed++; 00225 logError("failed to send", ret, mDot::getReturnCodeString(ret).c_str()); 00226 osDelay(std::max((uint32_t)5000, (uint32_t)dot->getNextTxMs())); 00227 } else { 00228 send_failed=0; 00229 logInfo("successfully sent data to gateway"); 00230 for(i=0;i<11;i++)recvBuf[i]=0; //clear recv buffer 00231 recvData.clear(); //clear recv data 00232 if ((ret = dot->recv(recvData)) != mDot::MDOT_OK) { 00233 logError("failed to recv: [%d][%s]", ret, mDot::getReturnCodeString(ret).c_str()); 00234 } else { 00235 //logInfo("datasize = %d", recvData.size()); 00236 for(i=0; i< recvData.size(); i++ ) 00237 recvBuf[i] = recvData[i]; 00238 //logInfo("RECV:%s", recvBuf); 00239 if(cmpStr(recvBuf, TURNON, 2)){ 00240 a4 = true; 00241 logInfo("A4 is on!"); 00242 } 00243 if(cmpStr(recvBuf, TURNOFF, 3)){ 00244 a4 = false; 00245 logInfo("A4 is off!"); 00246 } 00247 } 00248 } 00249 osDelay(std::max((uint32_t)5000, (uint32_t)dot->getNextTxMs())); 00250 00251 if(a1 || a2 || a3 || a4){ 00252 logInfo("============================"); 00253 logInfo("Alarm is ON"); 00254 Alarm = 1; 00255 }else{ 00256 logInfo("============================"); 00257 logInfo("Alarm is OFF"); 00258 Alarm = 0; 00259 } 00260 00261 osDelay(std::max((uint32_t)5000, (uint32_t)dot->getNextTxMs())); 00262 00263 00264 if(send_failed>=3){ 00265 send_failed=0; 00266 // attempt to rejoin the network 00267 logInfo("Attemp to rejoin network...."); 00268 if ((ret = dot->joinNetworkOnce()) != mDot::MDOT_OK) { 00269 logError("Failed to rejoin network!"); // %d:%s", ret, mDot::getReturnCodeString(ret).c_str()); 00270 }else{ 00271 logInfo("Rejoin network successfully!"); 00272 } 00273 osDelay(std::max((uint32_t)5000, (uint32_t)dot->getNextTxMs())); 00274 } 00275 00276 } 00277 00278 }
Generated on Wed Jul 13 2022 20:51:59 by
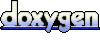