
Esta versión v6 pasa a ser el nuevo master. Funciona correctamente
Dependencies: ADXL345 Display1602 MSCFileSystem SDFileSystem mbed FATFileSystem
RingBuffer.cpp
00001 #include "RingBuffer.h" 00002 00003 Buffer::Buffer(int size) : bufSize(size), full(false), empty(true), windex(0), rindex(0) 00004 { 00005 data = std::vector<float>(bufSize); 00006 itBeg = data.begin(); 00007 itEnd = data.end(); 00008 head = itBeg; 00009 tail = itBeg; 00010 } 00011 00012 void Buffer::put(float val) 00013 { 00014 if(!full) 00015 { 00016 //std::cout << "Escribiendo " << val << " en " << windex << std::endl; 00017 data[windex] = val; 00018 windex++; 00019 empty = false; 00020 if(windex >= bufSize) 00021 { 00022 windex = 0; 00023 } 00024 if(windex == rindex) 00025 { 00026 full = true; 00027 //std::cout << "Buffer lleno..." << std::endl; 00028 } 00029 } 00030 } 00031 00032 const float Buffer::get() 00033 { 00034 float temp; 00035 if(!empty) 00036 { 00037 temp = data[rindex]; 00038 //std::cout << "Leyendo " << temp << " de " << rindex << std::endl; 00039 data[rindex] = 0; 00040 full = false; 00041 rindex++; 00042 if(rindex >= bufSize) 00043 { 00044 rindex = 0; 00045 } 00046 if(rindex == windex) 00047 { 00048 empty = true; 00049 //std::cout << "Buffer vacío. R-Index: " << rindex << std::endl; 00050 } 00051 } 00052 return temp; 00053 } 00054 00055 const bool Buffer::isFull() 00056 { 00057 return full; 00058 } 00059 00060 const bool Buffer::isEmpty() 00061 { 00062 return empty; 00063 } 00064 00065 const int Buffer::getSize() 00066 { 00067 return bufSize; 00068 } 00069 00070 const int Buffer::getWritingIndex() 00071 { 00072 return windex; 00073 } 00074 00075 const int Buffer::getReadingIndex() 00076 { 00077 return rindex; 00078 } 00079 00080 /* 00081 void Buffer::printBuffer() 00082 { 00083 std::cout << "Imprimiendo buffer..." << std::endl; 00084 int k = 0; 00085 for(std::vector<float>::iterator it = data.begin(); it != data.end(); ++it) 00086 { 00087 std::cout << "Elemento " << k++ << " es: " << *it << std::endl; 00088 } 00089 } 00090 */ 00091 00092
Generated on Thu Jul 14 2022 18:41:53 by
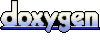