mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
x509_csr.h
00001 /** 00002 * \file mbedtls_x509_csr.h 00003 * 00004 * \brief X.509 certificate signing request parsing and writing 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_X509_CSR_H 00024 #define MBEDTLS_X509_CSR_H 00025 00026 #if !defined(MBEDTLS_CONFIG_FILE) 00027 #include "config.h" 00028 #else 00029 #include MBEDTLS_CONFIG_FILE 00030 #endif 00031 00032 #include "x509.h" 00033 00034 #ifdef __cplusplus 00035 extern "C" { 00036 #endif 00037 00038 /** 00039 * \addtogroup x509_module 00040 * \{ */ 00041 00042 /** 00043 * \name Structures and functions for X.509 Certificate Signing Requests (CSR) 00044 * \{ 00045 */ 00046 00047 /** 00048 * Certificate Signing Request (CSR) structure. 00049 */ 00050 typedef struct mbedtls_x509_csr 00051 { 00052 mbedtls_x509_buf raw; /**< The raw CSR data (DER). */ 00053 mbedtls_x509_buf cri; /**< The raw CertificateRequestInfo body (DER). */ 00054 00055 int version; /**< CSR version (1=v1). */ 00056 00057 mbedtls_x509_buf subject_raw; /**< The raw subject data (DER). */ 00058 mbedtls_x509_name subject; /**< The parsed subject data (named information object). */ 00059 00060 mbedtls_pk_context pk; /**< Container for the public key context. */ 00061 00062 mbedtls_x509_buf sig_oid; 00063 mbedtls_x509_buf sig; 00064 mbedtls_md_type_t sig_md; /**< Internal representation of the MD algorithm of the signature algorithm, e.g. MBEDTLS_MD_SHA256 */ 00065 mbedtls_pk_type_t sig_pk; /**< Internal representation of the Public Key algorithm of the signature algorithm, e.g. MBEDTLS_PK_RSA */ 00066 void *sig_opts; /**< Signature options to be passed to mbedtls_pk_verify_ext(), e.g. for RSASSA-PSS */ 00067 } 00068 mbedtls_x509_csr; 00069 00070 /** 00071 * Container for writing a CSR 00072 */ 00073 typedef struct mbedtls_x509write_csr 00074 { 00075 mbedtls_pk_context *key; 00076 mbedtls_asn1_named_data *subject; 00077 mbedtls_md_type_t md_alg; 00078 mbedtls_asn1_named_data *extensions; 00079 } 00080 mbedtls_x509write_csr; 00081 00082 #if defined(MBEDTLS_X509_CSR_PARSE_C) 00083 /** 00084 * \brief Load a Certificate Signing Request (CSR) in DER format 00085 * 00086 * \param csr CSR context to fill 00087 * \param buf buffer holding the CRL data 00088 * \param buflen size of the buffer 00089 * 00090 * \return 0 if successful, or a specific X509 error code 00091 */ 00092 int mbedtls_x509_csr_parse_der( mbedtls_x509_csr *csr, 00093 const unsigned char *buf, size_t buflen ); 00094 00095 /** 00096 * \brief Load a Certificate Signing Request (CSR), DER or PEM format 00097 * 00098 * \param csr CSR context to fill 00099 * \param buf buffer holding the CRL data 00100 * \param buflen size of the buffer 00101 * (including the terminating null byte for PEM data) 00102 * 00103 * \return 0 if successful, or a specific X509 or PEM error code 00104 */ 00105 int mbedtls_x509_csr_parse( mbedtls_x509_csr *csr, const unsigned char *buf, size_t buflen ); 00106 00107 #if defined(MBEDTLS_FS_IO) 00108 /** 00109 * \brief Load a Certificate Signing Request (CSR) 00110 * 00111 * \param csr CSR context to fill 00112 * \param path filename to read the CSR from 00113 * 00114 * \return 0 if successful, or a specific X509 or PEM error code 00115 */ 00116 int mbedtls_x509_csr_parse_file( mbedtls_x509_csr *csr, const char *path ); 00117 #endif /* MBEDTLS_FS_IO */ 00118 00119 /** 00120 * \brief Returns an informational string about the 00121 * CSR. 00122 * 00123 * \param buf Buffer to write to 00124 * \param size Maximum size of buffer 00125 * \param prefix A line prefix 00126 * \param csr The X509 CSR to represent 00127 * 00128 * \return The length of the string written (not including the 00129 * terminated nul byte), or a negative error code. 00130 */ 00131 int mbedtls_x509_csr_info( char *buf, size_t size, const char *prefix, 00132 const mbedtls_x509_csr *csr ); 00133 00134 /** 00135 * \brief Initialize a CSR 00136 * 00137 * \param csr CSR to initialize 00138 */ 00139 void mbedtls_x509_csr_init( mbedtls_x509_csr *csr ); 00140 00141 /** 00142 * \brief Unallocate all CSR data 00143 * 00144 * \param csr CSR to free 00145 */ 00146 void mbedtls_x509_csr_free( mbedtls_x509_csr *csr ); 00147 #endif /* MBEDTLS_X509_CSR_PARSE_C */ 00148 00149 /* \} name */ 00150 /* \} addtogroup x509_module */ 00151 00152 #if defined(MBEDTLS_X509_CSR_WRITE_C) 00153 /** 00154 * \brief Initialize a CSR context 00155 * 00156 * \param ctx CSR context to initialize 00157 */ 00158 void mbedtls_x509write_csr_init( mbedtls_x509write_csr *ctx ); 00159 00160 /** 00161 * \brief Set the subject name for a CSR 00162 * Subject names should contain a comma-separated list 00163 * of OID types and values: 00164 * e.g. "C=UK,O=ARM,CN=mbed TLS Server 1" 00165 * 00166 * \param ctx CSR context to use 00167 * \param subject_name subject name to set 00168 * 00169 * \return 0 if subject name was parsed successfully, or 00170 * a specific error code 00171 */ 00172 int mbedtls_x509write_csr_set_subject_name( mbedtls_x509write_csr *ctx, 00173 const char *subject_name ); 00174 00175 /** 00176 * \brief Set the key for a CSR (public key will be included, 00177 * private key used to sign the CSR when writing it) 00178 * 00179 * \param ctx CSR context to use 00180 * \param key Asymetric key to include 00181 */ 00182 void mbedtls_x509write_csr_set_key( mbedtls_x509write_csr *ctx, mbedtls_pk_context *key ); 00183 00184 /** 00185 * \brief Set the MD algorithm to use for the signature 00186 * (e.g. MBEDTLS_MD_SHA1) 00187 * 00188 * \param ctx CSR context to use 00189 * \param md_alg MD algorithm to use 00190 */ 00191 void mbedtls_x509write_csr_set_md_alg( mbedtls_x509write_csr *ctx, mbedtls_md_type_t md_alg ); 00192 00193 /** 00194 * \brief Set the Key Usage Extension flags 00195 * (e.g. MBEDTLS_X509_KU_DIGITAL_SIGNATURE | MBEDTLS_X509_KU_KEY_CERT_SIGN) 00196 * 00197 * \param ctx CSR context to use 00198 * \param key_usage key usage flags to set 00199 * 00200 * \return 0 if successful, or MBEDTLS_ERR_X509_ALLOC_FAILED 00201 */ 00202 int mbedtls_x509write_csr_set_key_usage( mbedtls_x509write_csr *ctx, unsigned char key_usage ); 00203 00204 /** 00205 * \brief Set the Netscape Cert Type flags 00206 * (e.g. MBEDTLS_X509_NS_CERT_TYPE_SSL_CLIENT | MBEDTLS_X509_NS_CERT_TYPE_EMAIL) 00207 * 00208 * \param ctx CSR context to use 00209 * \param ns_cert_type Netscape Cert Type flags to set 00210 * 00211 * \return 0 if successful, or MBEDTLS_ERR_X509_ALLOC_FAILED 00212 */ 00213 int mbedtls_x509write_csr_set_ns_cert_type( mbedtls_x509write_csr *ctx, 00214 unsigned char ns_cert_type ); 00215 00216 /** 00217 * \brief Generic function to add to or replace an extension in the 00218 * CSR 00219 * 00220 * \param ctx CSR context to use 00221 * \param oid OID of the extension 00222 * \param oid_len length of the OID 00223 * \param val value of the extension OCTET STRING 00224 * \param val_len length of the value data 00225 * 00226 * \return 0 if successful, or a MBEDTLS_ERR_X509_ALLOC_FAILED 00227 */ 00228 int mbedtls_x509write_csr_set_extension( mbedtls_x509write_csr *ctx, 00229 const char *oid, size_t oid_len, 00230 const unsigned char *val, size_t val_len ); 00231 00232 /** 00233 * \brief Free the contents of a CSR context 00234 * 00235 * \param ctx CSR context to free 00236 */ 00237 void mbedtls_x509write_csr_free( mbedtls_x509write_csr *ctx ); 00238 00239 /** 00240 * \brief Write a CSR (Certificate Signing Request) to a 00241 * DER structure 00242 * Note: data is written at the end of the buffer! Use the 00243 * return value to determine where you should start 00244 * using the buffer 00245 * 00246 * \param ctx CSR to write away 00247 * \param buf buffer to write to 00248 * \param size size of the buffer 00249 * \param f_rng RNG function (for signature, see note) 00250 * \param p_rng RNG parameter 00251 * 00252 * \return length of data written if successful, or a specific 00253 * error code 00254 * 00255 * \note f_rng may be NULL if RSA is used for signature and the 00256 * signature is made offline (otherwise f_rng is desirable 00257 * for countermeasures against timing attacks). 00258 * ECDSA signatures always require a non-NULL f_rng. 00259 */ 00260 int mbedtls_x509write_csr_der( mbedtls_x509write_csr *ctx, unsigned char *buf, size_t size, 00261 int (*f_rng)(void *, unsigned char *, size_t), 00262 void *p_rng ); 00263 00264 #if defined(MBEDTLS_PEM_WRITE_C) 00265 /** 00266 * \brief Write a CSR (Certificate Signing Request) to a 00267 * PEM string 00268 * 00269 * \param ctx CSR to write away 00270 * \param buf buffer to write to 00271 * \param size size of the buffer 00272 * \param f_rng RNG function (for signature, see note) 00273 * \param p_rng RNG parameter 00274 * 00275 * \return 0 if successful, or a specific error code 00276 * 00277 * \note f_rng may be NULL if RSA is used for signature and the 00278 * signature is made offline (otherwise f_rng is desirable 00279 * for couermeasures against timing attacks). 00280 * ECDSA signatures always require a non-NULL f_rng. 00281 */ 00282 int mbedtls_x509write_csr_pem( mbedtls_x509write_csr *ctx, unsigned char *buf, size_t size, 00283 int (*f_rng)(void *, unsigned char *, size_t), 00284 void *p_rng ); 00285 #endif /* MBEDTLS_PEM_WRITE_C */ 00286 #endif /* MBEDTLS_X509_CSR_WRITE_C */ 00287 00288 #ifdef __cplusplus 00289 } 00290 #endif 00291 00292 #endif /* mbedtls_x509_csr.h */
Generated on Tue Jul 12 2022 12:52:50 by
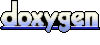