mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
threading.h
Go to the documentation of this file.
00001 /** 00002 * \file threading.h 00003 * 00004 * \brief Threading abstraction layer 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_THREADING_H 00024 #define MBEDTLS_THREADING_H 00025 00026 #if !defined(MBEDTLS_CONFIG_FILE) 00027 #include "config.h" 00028 #else 00029 #include MBEDTLS_CONFIG_FILE 00030 #endif 00031 00032 #include <stdlib.h> 00033 00034 #ifdef __cplusplus 00035 extern "C" { 00036 #endif 00037 00038 #define MBEDTLS_ERR_THREADING_FEATURE_UNAVAILABLE -0x001A /**< The selected feature is not available. */ 00039 #define MBEDTLS_ERR_THREADING_BAD_INPUT_DATA -0x001C /**< Bad input parameters to function. */ 00040 #define MBEDTLS_ERR_THREADING_MUTEX_ERROR -0x001E /**< Locking / unlocking / free failed with error code. */ 00041 00042 #if defined(MBEDTLS_THREADING_PTHREAD) 00043 #include <pthread.h> 00044 typedef struct 00045 { 00046 pthread_mutex_t mutex; 00047 char is_valid; 00048 } mbedtls_threading_mutex_t; 00049 #endif 00050 00051 #if defined(MBEDTLS_THREADING_ALT) 00052 /* You should define the mbedtls_threading_mutex_t type in your header */ 00053 #include "threading_alt.h" 00054 00055 /** 00056 * \brief Set your alternate threading implementation function 00057 * pointers and initialize global mutexes. If used, this 00058 * function must be called once in the main thread before any 00059 * other mbed TLS function is called, and 00060 * mbedtls_threading_free_alt() must be called once in the main 00061 * thread after all other mbed TLS functions. 00062 * 00063 * \note mutex_init() and mutex_free() don't return a status code. 00064 * If mutex_init() fails, it should leave its argument (the 00065 * mutex) in a state such that mutex_lock() will fail when 00066 * called with this argument. 00067 * 00068 * \param mutex_init the init function implementation 00069 * \param mutex_free the free function implementation 00070 * \param mutex_lock the lock function implementation 00071 * \param mutex_unlock the unlock function implementation 00072 */ 00073 void mbedtls_threading_set_alt( void (*mutex_init)( mbedtls_threading_mutex_t * ), 00074 void (*mutex_free)( mbedtls_threading_mutex_t * ), 00075 int (*mutex_lock)( mbedtls_threading_mutex_t * ), 00076 int (*mutex_unlock)( mbedtls_threading_mutex_t * ) ); 00077 00078 /** 00079 * \brief Free global mutexes. 00080 */ 00081 void mbedtls_threading_free_alt( void ); 00082 #endif /* MBEDTLS_THREADING_ALT */ 00083 00084 /* 00085 * The function pointers for mutex_init, mutex_free, mutex_ and mutex_unlock 00086 * 00087 * All these functions are expected to work or the result will be undefined. 00088 */ 00089 extern void (*mbedtls_mutex_init)( mbedtls_threading_mutex_t *mutex ); 00090 extern void (*mbedtls_mutex_free)( mbedtls_threading_mutex_t *mutex ); 00091 extern int (*mbedtls_mutex_lock)( mbedtls_threading_mutex_t *mutex ); 00092 extern int (*mbedtls_mutex_unlock)( mbedtls_threading_mutex_t *mutex ); 00093 00094 /* 00095 * Global mutexes 00096 */ 00097 extern mbedtls_threading_mutex_t mbedtls_threading_readdir_mutex; 00098 extern mbedtls_threading_mutex_t mbedtls_threading_gmtime_mutex; 00099 00100 #ifdef __cplusplus 00101 } 00102 #endif 00103 00104 #endif /* threading.h */
Generated on Tue Jul 12 2022 12:52:49 by
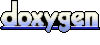