mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
ssl_cookie.h
Go to the documentation of this file.
00001 /** 00002 * \file ssl_cookie.h 00003 * 00004 * \brief DTLS cookie callbacks implementation 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_SSL_COOKIE_H 00024 #define MBEDTLS_SSL_COOKIE_H 00025 00026 #include "ssl.h" 00027 00028 #if defined(MBEDTLS_THREADING_C) 00029 #include "threading.h" 00030 #endif 00031 00032 /** 00033 * \name SECTION: Module settings 00034 * 00035 * The configuration options you can set for this module are in this section. 00036 * Either change them in config.h or define them on the compiler command line. 00037 * \{ 00038 */ 00039 #ifndef MBEDTLS_SSL_COOKIE_TIMEOUT 00040 #define MBEDTLS_SSL_COOKIE_TIMEOUT 60 /**< Default expiration delay of DTLS cookies, in seconds if HAVE_TIME, or in number of cookies issued */ 00041 #endif 00042 00043 /* \} name SECTION: Module settings */ 00044 00045 #ifdef __cplusplus 00046 extern "C" { 00047 #endif 00048 00049 /** 00050 * \brief Context for the default cookie functions. 00051 */ 00052 typedef struct 00053 { 00054 mbedtls_md_context_t hmac_ctx ; /*!< context for the HMAC portion */ 00055 #if !defined(MBEDTLS_HAVE_TIME) 00056 unsigned long serial ; /*!< serial number for expiration */ 00057 #endif 00058 unsigned long timeout; /*!< timeout delay, in seconds if HAVE_TIME, 00059 or in number of tickets issued */ 00060 00061 #if defined(MBEDTLS_THREADING_C) 00062 mbedtls_threading_mutex_t mutex; 00063 #endif 00064 } mbedtls_ssl_cookie_ctx; 00065 00066 /** 00067 * \brief Initialize cookie context 00068 */ 00069 void mbedtls_ssl_cookie_init( mbedtls_ssl_cookie_ctx *ctx ); 00070 00071 /** 00072 * \brief Setup cookie context (generate keys) 00073 */ 00074 int mbedtls_ssl_cookie_setup( mbedtls_ssl_cookie_ctx *ctx, 00075 int (*f_rng)(void *, unsigned char *, size_t), 00076 void *p_rng ); 00077 00078 /** 00079 * \brief Set expiration delay for cookies 00080 * (Default MBEDTLS_SSL_COOKIE_TIMEOUT) 00081 * 00082 * \param ctx Cookie contex 00083 * \param delay Delay, in seconds if HAVE_TIME, or in number of cookies 00084 * issued in the meantime. 00085 * 0 to disable expiration (NOT recommended) 00086 */ 00087 void mbedtls_ssl_cookie_set_timeout( mbedtls_ssl_cookie_ctx *ctx, unsigned long delay ); 00088 00089 /** 00090 * \brief Free cookie context 00091 */ 00092 void mbedtls_ssl_cookie_free( mbedtls_ssl_cookie_ctx *ctx ); 00093 00094 /** 00095 * \brief Generate cookie, see \c mbedtls_ssl_cookie_write_t 00096 */ 00097 mbedtls_ssl_cookie_write_t mbedtls_ssl_cookie_write; 00098 00099 /** 00100 * \brief Verify cookie, see \c mbedtls_ssl_cookie_write_t 00101 */ 00102 mbedtls_ssl_cookie_check_t mbedtls_ssl_cookie_check; 00103 00104 #ifdef __cplusplus 00105 } 00106 #endif 00107 00108 #endif /* ssl_cookie.h */
Generated on Tue Jul 12 2022 12:52:47 by
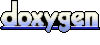