mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
platform.c
00001 /* 00002 * Platform abstraction layer 00003 * 00004 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00005 * SPDX-License-Identifier: Apache-2.0 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00008 * not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00015 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 * 00019 * This file is part of mbed TLS (https://tls.mbed.org) 00020 */ 00021 00022 #if !defined(MBEDTLS_CONFIG_FILE) 00023 #include "mbedtls/config.h" 00024 #else 00025 #include MBEDTLS_CONFIG_FILE 00026 #endif 00027 00028 #if defined(MBEDTLS_PLATFORM_C) 00029 00030 #include "mbedtls/platform.h" 00031 00032 #if defined(MBEDTLS_PLATFORM_MEMORY) 00033 #if !defined(MBEDTLS_PLATFORM_STD_CALLOC) 00034 static void *platform_calloc_uninit( size_t n, size_t size ) 00035 { 00036 ((void) n); 00037 ((void) size); 00038 return( NULL ); 00039 } 00040 00041 #define MBEDTLS_PLATFORM_STD_CALLOC platform_calloc_uninit 00042 #endif /* !MBEDTLS_PLATFORM_STD_CALLOC */ 00043 00044 #if !defined(MBEDTLS_PLATFORM_STD_FREE) 00045 static void platform_free_uninit( void *ptr ) 00046 { 00047 ((void) ptr); 00048 } 00049 00050 #define MBEDTLS_PLATFORM_STD_FREE platform_free_uninit 00051 #endif /* !MBEDTLS_PLATFORM_STD_FREE */ 00052 00053 void * (*mbedtls_calloc)( size_t, size_t ) = MBEDTLS_PLATFORM_STD_CALLOC; 00054 void (*mbedtls_free)( void * ) = MBEDTLS_PLATFORM_STD_FREE; 00055 00056 int mbedtls_platform_set_calloc_free( void * (*calloc_func)( size_t, size_t ), 00057 void (*free_func)( void * ) ) 00058 { 00059 mbedtls_calloc = calloc_func; 00060 mbedtls_free = free_func; 00061 return( 0 ); 00062 } 00063 #endif /* MBEDTLS_PLATFORM_MEMORY */ 00064 00065 #if defined(_WIN32) 00066 #include <stdarg.h> 00067 int mbedtls_platform_win32_snprintf( char *s, size_t n, const char *fmt, ... ) 00068 { 00069 int ret; 00070 va_list argp; 00071 00072 /* Avoid calling the invalid parameter handler by checking ourselves */ 00073 if( s == NULL || n == 0 || fmt == NULL ) 00074 return( -1 ); 00075 00076 va_start( argp, fmt ); 00077 #if defined(_TRUNCATE) 00078 ret = _vsnprintf_s( s, n, _TRUNCATE, fmt, argp ); 00079 #else 00080 ret = _vsnprintf( s, n, fmt, argp ); 00081 if( ret < 0 || (size_t) ret == n ) 00082 { 00083 s[n-1] = '\0'; 00084 ret = -1; 00085 } 00086 #endif 00087 va_end( argp ); 00088 00089 return( ret ); 00090 } 00091 #endif 00092 00093 #if defined(MBEDTLS_PLATFORM_SNPRINTF_ALT) 00094 #if !defined(MBEDTLS_PLATFORM_STD_SNPRINTF) 00095 /* 00096 * Make dummy function to prevent NULL pointer dereferences 00097 */ 00098 static int platform_snprintf_uninit( char * s, size_t n, 00099 const char * format, ... ) 00100 { 00101 ((void) s); 00102 ((void) n); 00103 ((void) format); 00104 return( 0 ); 00105 } 00106 00107 #define MBEDTLS_PLATFORM_STD_SNPRINTF platform_snprintf_uninit 00108 #endif /* !MBEDTLS_PLATFORM_STD_SNPRINTF */ 00109 00110 int (*mbedtls_snprintf)( char * s, size_t n, 00111 const char * format, 00112 ... ) = MBEDTLS_PLATFORM_STD_SNPRINTF; 00113 00114 int mbedtls_platform_set_snprintf( int (*snprintf_func)( char * s, size_t n, 00115 const char * format, 00116 ... ) ) 00117 { 00118 mbedtls_snprintf = snprintf_func; 00119 return( 0 ); 00120 } 00121 #endif /* MBEDTLS_PLATFORM_SNPRINTF_ALT */ 00122 00123 #if defined(MBEDTLS_PLATFORM_PRINTF_ALT) 00124 #if !defined(MBEDTLS_PLATFORM_STD_PRINTF) 00125 /* 00126 * Make dummy function to prevent NULL pointer dereferences 00127 */ 00128 static int platform_printf_uninit( const char *format, ... ) 00129 { 00130 ((void) format); 00131 return( 0 ); 00132 } 00133 00134 #define MBEDTLS_PLATFORM_STD_PRINTF platform_printf_uninit 00135 #endif /* !MBEDTLS_PLATFORM_STD_PRINTF */ 00136 00137 int (*mbedtls_printf)( const char *, ... ) = MBEDTLS_PLATFORM_STD_PRINTF; 00138 00139 int mbedtls_platform_set_printf( int (*printf_func)( const char *, ... ) ) 00140 { 00141 mbedtls_printf = printf_func; 00142 return( 0 ); 00143 } 00144 #endif /* MBEDTLS_PLATFORM_PRINTF_ALT */ 00145 00146 #if defined(MBEDTLS_PLATFORM_FPRINTF_ALT) 00147 #if !defined(MBEDTLS_PLATFORM_STD_FPRINTF) 00148 /* 00149 * Make dummy function to prevent NULL pointer dereferences 00150 */ 00151 static int platform_fprintf_uninit( FILE *stream, const char *format, ... ) 00152 { 00153 ((void) stream); 00154 ((void) format); 00155 return( 0 ); 00156 } 00157 00158 #define MBEDTLS_PLATFORM_STD_FPRINTF platform_fprintf_uninit 00159 #endif /* !MBEDTLS_PLATFORM_STD_FPRINTF */ 00160 00161 int (*mbedtls_fprintf)( FILE *, const char *, ... ) = 00162 MBEDTLS_PLATFORM_STD_FPRINTF; 00163 00164 int mbedtls_platform_set_fprintf( int (*fprintf_func)( FILE *, const char *, ... ) ) 00165 { 00166 mbedtls_fprintf = fprintf_func; 00167 return( 0 ); 00168 } 00169 #endif /* MBEDTLS_PLATFORM_FPRINTF_ALT */ 00170 00171 #if defined(MBEDTLS_PLATFORM_EXIT_ALT) 00172 #if !defined(MBEDTLS_PLATFORM_STD_EXIT) 00173 /* 00174 * Make dummy function to prevent NULL pointer dereferences 00175 */ 00176 static void platform_exit_uninit( int status ) 00177 { 00178 ((void) status); 00179 } 00180 00181 #define MBEDTLS_PLATFORM_STD_EXIT platform_exit_uninit 00182 #endif /* !MBEDTLS_PLATFORM_STD_EXIT */ 00183 00184 void (*mbedtls_exit)( int status ) = MBEDTLS_PLATFORM_STD_EXIT; 00185 00186 int mbedtls_platform_set_exit( void (*exit_func)( int status ) ) 00187 { 00188 mbedtls_exit = exit_func; 00189 return( 0 ); 00190 } 00191 #endif /* MBEDTLS_PLATFORM_EXIT_ALT */ 00192 00193 #endif /* MBEDTLS_PLATFORM_C */
Generated on Tue Jul 12 2022 12:52:46 by
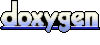