mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
pk_internal.h
00001 /** 00002 * \file pk.h 00003 * 00004 * \brief Public Key abstraction layer: wrapper functions 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 00024 #ifndef MBEDTLS_PK_WRAP_H 00025 #define MBEDTLS_PK_WRAP_H 00026 00027 #if !defined(MBEDTLS_CONFIG_FILE) 00028 #include "config.h" 00029 #else 00030 #include MBEDTLS_CONFIG_FILE 00031 #endif 00032 00033 #include "pk.h" 00034 00035 struct mbedtls_pk_info_t 00036 { 00037 /** Public key type */ 00038 mbedtls_pk_type_t type; 00039 00040 /** Type name */ 00041 const char *name; 00042 00043 /** Get key size in bits */ 00044 size_t (*get_bitlen)( const void * ); 00045 00046 /** Tell if the context implements this type (e.g. ECKEY can do ECDSA) */ 00047 int (*can_do)( mbedtls_pk_type_t type ); 00048 00049 /** Verify signature */ 00050 int (*verify_func)( void *ctx, mbedtls_md_type_t md_alg, 00051 const unsigned char *hash, size_t hash_len, 00052 const unsigned char *sig, size_t sig_len ); 00053 00054 /** Make signature */ 00055 int (*sign_func)( void *ctx, mbedtls_md_type_t md_alg, 00056 const unsigned char *hash, size_t hash_len, 00057 unsigned char *sig, size_t *sig_len, 00058 int (*f_rng)(void *, unsigned char *, size_t), 00059 void *p_rng ); 00060 00061 /** Decrypt message */ 00062 int (*decrypt_func)( void *ctx, const unsigned char *input, size_t ilen, 00063 unsigned char *output, size_t *olen, size_t osize, 00064 int (*f_rng)(void *, unsigned char *, size_t), 00065 void *p_rng ); 00066 00067 /** Encrypt message */ 00068 int (*encrypt_func)( void *ctx, const unsigned char *input, size_t ilen, 00069 unsigned char *output, size_t *olen, size_t osize, 00070 int (*f_rng)(void *, unsigned char *, size_t), 00071 void *p_rng ); 00072 00073 /** Check public-private key pair */ 00074 int (*check_pair_func)( const void *pub, const void *prv ); 00075 00076 /** Allocate a new context */ 00077 void * (*ctx_alloc_func)( void ); 00078 00079 /** Free the given context */ 00080 void (*ctx_free_func)( void *ctx ); 00081 00082 /** Interface with the debug module */ 00083 void (*debug_func)( const void *ctx, mbedtls_pk_debug_item *items ); 00084 00085 }; 00086 #if defined(MBEDTLS_PK_RSA_ALT_SUPPORT) 00087 /* Container for RSA-alt */ 00088 typedef struct 00089 { 00090 void *key; 00091 mbedtls_pk_rsa_alt_decrypt_func decrypt_func; 00092 mbedtls_pk_rsa_alt_sign_func sign_func; 00093 mbedtls_pk_rsa_alt_key_len_func key_len_func; 00094 } mbedtls_rsa_alt_context; 00095 #endif 00096 00097 #if defined(MBEDTLS_RSA_C) 00098 extern const mbedtls_pk_info_t mbedtls_rsa_info; 00099 #endif 00100 00101 #if defined(MBEDTLS_ECP_C) 00102 extern const mbedtls_pk_info_t mbedtls_eckey_info; 00103 extern const mbedtls_pk_info_t mbedtls_eckeydh_info; 00104 #endif 00105 00106 #if defined(MBEDTLS_ECDSA_C) 00107 extern const mbedtls_pk_info_t mbedtls_ecdsa_info; 00108 #endif 00109 00110 #if defined(MBEDTLS_PK_RSA_ALT_SUPPORT) 00111 extern const mbedtls_pk_info_t mbedtls_rsa_alt_info; 00112 #endif 00113 00114 #endif /* MBEDTLS_PK_WRAP_H */
Generated on Tue Jul 12 2022 12:52:45 by
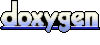