mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
padlock.c
00001 /* 00002 * VIA PadLock support functions 00003 * 00004 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00005 * SPDX-License-Identifier: Apache-2.0 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00008 * not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00015 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 * 00019 * This file is part of mbed TLS (https://tls.mbed.org) 00020 */ 00021 /* 00022 * This implementation is based on the VIA PadLock Programming Guide: 00023 * 00024 * http://www.via.com.tw/en/downloads/whitepapers/initiatives/padlock/ 00025 * programming_guide.pdf 00026 */ 00027 00028 #if !defined(MBEDTLS_CONFIG_FILE) 00029 #include "mbedtls/config.h" 00030 #else 00031 #include MBEDTLS_CONFIG_FILE 00032 #endif 00033 00034 #if defined(MBEDTLS_PADLOCK_C) 00035 00036 #include "mbedtls/padlock.h" 00037 00038 #include <string.h> 00039 00040 #ifndef asm 00041 #define asm __asm 00042 #endif 00043 00044 #if defined(MBEDTLS_HAVE_X86) 00045 00046 /* 00047 * PadLock detection routine 00048 */ 00049 int mbedtls_padlock_has_support( int feature ) 00050 { 00051 static int flags = -1; 00052 int ebx = 0, edx = 0; 00053 00054 if( flags == -1 ) 00055 { 00056 asm( "movl %%ebx, %0 \n\t" 00057 "movl $0xC0000000, %%eax \n\t" 00058 "cpuid \n\t" 00059 "cmpl $0xC0000001, %%eax \n\t" 00060 "movl $0, %%edx \n\t" 00061 "jb unsupported \n\t" 00062 "movl $0xC0000001, %%eax \n\t" 00063 "cpuid \n\t" 00064 "unsupported: \n\t" 00065 "movl %%edx, %1 \n\t" 00066 "movl %2, %%ebx \n\t" 00067 : "=m" (ebx), "=m" (edx) 00068 : "m" (ebx) 00069 : "eax", "ecx", "edx" ); 00070 00071 flags = edx; 00072 } 00073 00074 return( flags & feature ); 00075 } 00076 00077 /* 00078 * PadLock AES-ECB block en(de)cryption 00079 */ 00080 int mbedtls_padlock_xcryptecb( mbedtls_aes_context *ctx, 00081 int mode, 00082 const unsigned char input[16], 00083 unsigned char output[16] ) 00084 { 00085 int ebx = 0; 00086 uint32_t *rk; 00087 uint32_t *blk; 00088 uint32_t *ctrl; 00089 unsigned char buf[256]; 00090 00091 rk = ctx->rk ; 00092 blk = MBEDTLS_PADLOCK_ALIGN16( buf ); 00093 memcpy( blk, input, 16 ); 00094 00095 ctrl = blk + 4; 00096 *ctrl = 0x80 | ctx->nr | ( ( ctx->nr + ( mode^1 ) - 10 ) << 9 ); 00097 00098 asm( "pushfl \n\t" 00099 "popfl \n\t" 00100 "movl %%ebx, %0 \n\t" 00101 "movl $1, %%ecx \n\t" 00102 "movl %2, %%edx \n\t" 00103 "movl %3, %%ebx \n\t" 00104 "movl %4, %%esi \n\t" 00105 "movl %4, %%edi \n\t" 00106 ".byte 0xf3,0x0f,0xa7,0xc8 \n\t" 00107 "movl %1, %%ebx \n\t" 00108 : "=m" (ebx) 00109 : "m" (ebx), "m" (ctrl), "m" (rk), "m" (blk) 00110 : "memory", "ecx", "edx", "esi", "edi" ); 00111 00112 memcpy( output, blk, 16 ); 00113 00114 return( 0 ); 00115 } 00116 00117 /* 00118 * PadLock AES-CBC buffer en(de)cryption 00119 */ 00120 int mbedtls_padlock_xcryptcbc( mbedtls_aes_context *ctx, 00121 int mode, 00122 size_t length, 00123 unsigned char iv[16], 00124 const unsigned char *input, 00125 unsigned char *output ) 00126 { 00127 int ebx = 0; 00128 size_t count; 00129 uint32_t *rk; 00130 uint32_t *iw; 00131 uint32_t *ctrl; 00132 unsigned char buf[256]; 00133 00134 if( ( (long) input & 15 ) != 0 || 00135 ( (long) output & 15 ) != 0 ) 00136 return( MBEDTLS_ERR_PADLOCK_DATA_MISALIGNED ); 00137 00138 rk = ctx->rk ; 00139 iw = MBEDTLS_PADLOCK_ALIGN16( buf ); 00140 memcpy( iw, iv, 16 ); 00141 00142 ctrl = iw + 4; 00143 *ctrl = 0x80 | ctx->nr | ( ( ctx->nr + ( mode ^ 1 ) - 10 ) << 9 ); 00144 00145 count = ( length + 15 ) >> 4; 00146 00147 asm( "pushfl \n\t" 00148 "popfl \n\t" 00149 "movl %%ebx, %0 \n\t" 00150 "movl %2, %%ecx \n\t" 00151 "movl %3, %%edx \n\t" 00152 "movl %4, %%ebx \n\t" 00153 "movl %5, %%esi \n\t" 00154 "movl %6, %%edi \n\t" 00155 "movl %7, %%eax \n\t" 00156 ".byte 0xf3,0x0f,0xa7,0xd0 \n\t" 00157 "movl %1, %%ebx \n\t" 00158 : "=m" (ebx) 00159 : "m" (ebx), "m" (count), "m" (ctrl), 00160 "m" (rk), "m" (input), "m" (output), "m" (iw) 00161 : "memory", "eax", "ecx", "edx", "esi", "edi" ); 00162 00163 memcpy( iv, iw, 16 ); 00164 00165 return( 0 ); 00166 } 00167 00168 #endif /* MBEDTLS_HAVE_X86 */ 00169 00170 #endif /* MBEDTLS_PADLOCK_C */
Generated on Tue Jul 12 2022 12:52:45 by
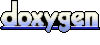