mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
md.h
00001 /** 00002 * \file mbedtls_md.h 00003 * 00004 * \brief Generic message digest wrapper 00005 * 00006 * \author Adriaan de Jong <dejong@fox-it.com> 00007 * 00008 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00009 * SPDX-License-Identifier: Apache-2.0 00010 * 00011 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00012 * not use this file except in compliance with the License. 00013 * You may obtain a copy of the License at 00014 * 00015 * http://www.apache.org/licenses/LICENSE-2.0 00016 * 00017 * Unless required by applicable law or agreed to in writing, software 00018 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00019 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00020 * See the License for the specific language governing permissions and 00021 * limitations under the License. 00022 * 00023 * This file is part of mbed TLS (https://tls.mbed.org) 00024 */ 00025 #ifndef MBEDTLS_MD_H 00026 #define MBEDTLS_MD_H 00027 00028 #include <stddef.h> 00029 00030 #define MBEDTLS_ERR_MD_FEATURE_UNAVAILABLE -0x5080 /**< The selected feature is not available. */ 00031 #define MBEDTLS_ERR_MD_BAD_INPUT_DATA -0x5100 /**< Bad input parameters to function. */ 00032 #define MBEDTLS_ERR_MD_ALLOC_FAILED -0x5180 /**< Failed to allocate memory. */ 00033 #define MBEDTLS_ERR_MD_FILE_IO_ERROR -0x5200 /**< Opening or reading of file failed. */ 00034 00035 #ifdef __cplusplus 00036 extern "C" { 00037 #endif 00038 00039 typedef enum { 00040 MBEDTLS_MD_NONE=0, 00041 MBEDTLS_MD_MD2, 00042 MBEDTLS_MD_MD4, 00043 MBEDTLS_MD_MD5, 00044 MBEDTLS_MD_SHA1, 00045 MBEDTLS_MD_SHA224, 00046 MBEDTLS_MD_SHA256, 00047 MBEDTLS_MD_SHA384, 00048 MBEDTLS_MD_SHA512, 00049 MBEDTLS_MD_RIPEMD160, 00050 } mbedtls_md_type_t; 00051 00052 #if defined(MBEDTLS_SHA512_C) 00053 #define MBEDTLS_MD_MAX_SIZE 64 /* longest known is SHA512 */ 00054 #else 00055 #define MBEDTLS_MD_MAX_SIZE 32 /* longest known is SHA256 or less */ 00056 #endif 00057 00058 /** 00059 * Opaque struct defined in md_internal.h 00060 */ 00061 typedef struct mbedtls_md_info_t mbedtls_md_info_t; 00062 00063 /** 00064 * Generic message digest context. 00065 */ 00066 typedef struct { 00067 /** Information about the associated message digest */ 00068 const mbedtls_md_info_t *md_info; 00069 00070 /** Digest-specific context */ 00071 void *md_ctx; 00072 00073 /** HMAC part of the context */ 00074 void *hmac_ctx; 00075 } mbedtls_md_context_t; 00076 00077 /** 00078 * \brief Returns the list of digests supported by the generic digest module. 00079 * 00080 * \return a statically allocated array of digests, the last entry 00081 * is 0. 00082 */ 00083 const int *mbedtls_md_list( void ); 00084 00085 /** 00086 * \brief Returns the message digest information associated with the 00087 * given digest name. 00088 * 00089 * \param md_name Name of the digest to search for. 00090 * 00091 * \return The message digest information associated with md_name or 00092 * NULL if not found. 00093 */ 00094 const mbedtls_md_info_t *mbedtls_md_info_from_string( const char *md_name ); 00095 00096 /** 00097 * \brief Returns the message digest information associated with the 00098 * given digest type. 00099 * 00100 * \param md_type type of digest to search for. 00101 * 00102 * \return The message digest information associated with md_type or 00103 * NULL if not found. 00104 */ 00105 const mbedtls_md_info_t *mbedtls_md_info_from_type( mbedtls_md_type_t md_type ); 00106 00107 /** 00108 * \brief Initialize a md_context (as NONE) 00109 * This should always be called first. 00110 * Prepares the context for mbedtls_md_setup() or mbedtls_md_free(). 00111 */ 00112 void mbedtls_md_init( mbedtls_md_context_t *ctx ); 00113 00114 /** 00115 * \brief Free and clear the internal structures of ctx. 00116 * Can be called at any time after mbedtls_md_init(). 00117 * Mandatory once mbedtls_md_setup() has been called. 00118 */ 00119 void mbedtls_md_free( mbedtls_md_context_t *ctx ); 00120 00121 #if ! defined(MBEDTLS_DEPRECATED_REMOVED) 00122 #if defined(MBEDTLS_DEPRECATED_WARNING) 00123 #define MBEDTLS_DEPRECATED __attribute__((deprecated)) 00124 #else 00125 #define MBEDTLS_DEPRECATED 00126 #endif 00127 /** 00128 * \brief Select MD to use and allocate internal structures. 00129 * Should be called after mbedtls_md_init() or mbedtls_md_free(). 00130 * Makes it necessary to call mbedtls_md_free() later. 00131 * 00132 * \deprecated Superseded by mbedtls_md_setup() in 2.0.0 00133 * 00134 * \param ctx Context to set up. 00135 * \param md_info Message digest to use. 00136 * 00137 * \returns \c 0 on success, 00138 * \c MBEDTLS_ERR_MD_BAD_INPUT_DATA on parameter failure, 00139 * \c MBEDTLS_ERR_MD_ALLOC_FAILED memory allocation failure. 00140 */ 00141 int mbedtls_md_init_ctx( mbedtls_md_context_t *ctx, const mbedtls_md_info_t *md_info ) MBEDTLS_DEPRECATED; 00142 #undef MBEDTLS_DEPRECATED 00143 #endif /* MBEDTLS_DEPRECATED_REMOVED */ 00144 00145 /** 00146 * \brief Select MD to use and allocate internal structures. 00147 * Should be called after mbedtls_md_init() or mbedtls_md_free(). 00148 * Makes it necessary to call mbedtls_md_free() later. 00149 * 00150 * \param ctx Context to set up. 00151 * \param md_info Message digest to use. 00152 * \param hmac 0 to save some memory if HMAC will not be used, 00153 * non-zero is HMAC is going to be used with this context. 00154 * 00155 * \returns \c 0 on success, 00156 * \c MBEDTLS_ERR_MD_BAD_INPUT_DATA on parameter failure, 00157 * \c MBEDTLS_ERR_MD_ALLOC_FAILED memory allocation failure. 00158 */ 00159 int mbedtls_md_setup( mbedtls_md_context_t *ctx, const mbedtls_md_info_t *md_info, int hmac ); 00160 00161 /** 00162 * \brief Clone the state of an MD context 00163 * 00164 * \note The two contexts must have been setup to the same type 00165 * (cloning from SHA-256 to SHA-512 make no sense). 00166 * 00167 * \warning Only clones the MD state, not the HMAC state! (for now) 00168 * 00169 * \param dst The destination context 00170 * \param src The context to be cloned 00171 * 00172 * \return \c 0 on success, 00173 * \c MBEDTLS_ERR_MD_BAD_INPUT_DATA on parameter failure. 00174 */ 00175 int mbedtls_md_clone( mbedtls_md_context_t *dst, 00176 const mbedtls_md_context_t *src ); 00177 00178 /** 00179 * \brief Returns the size of the message digest output. 00180 * 00181 * \param md_info message digest info 00182 * 00183 * \return size of the message digest output in bytes. 00184 */ 00185 unsigned char mbedtls_md_get_size( const mbedtls_md_info_t *md_info ); 00186 00187 /** 00188 * \brief Returns the type of the message digest output. 00189 * 00190 * \param md_info message digest info 00191 * 00192 * \return type of the message digest output. 00193 */ 00194 mbedtls_md_type_t mbedtls_md_get_type( const mbedtls_md_info_t *md_info ); 00195 00196 /** 00197 * \brief Returns the name of the message digest output. 00198 * 00199 * \param md_info message digest info 00200 * 00201 * \return name of the message digest output. 00202 */ 00203 const char *mbedtls_md_get_name( const mbedtls_md_info_t *md_info ); 00204 00205 /** 00206 * \brief Prepare the context to digest a new message. 00207 * Generally called after mbedtls_md_setup() or mbedtls_md_finish(). 00208 * Followed by mbedtls_md_update(). 00209 * 00210 * \param ctx generic message digest context. 00211 * 00212 * \returns 0 on success, MBEDTLS_ERR_MD_BAD_INPUT_DATA if parameter 00213 * verification fails. 00214 */ 00215 int mbedtls_md_starts( mbedtls_md_context_t *ctx ); 00216 00217 /** 00218 * \brief Generic message digest process buffer 00219 * Called between mbedtls_md_starts() and mbedtls_md_finish(). 00220 * May be called repeatedly. 00221 * 00222 * \param ctx Generic message digest context 00223 * \param input buffer holding the datal 00224 * \param ilen length of the input data 00225 * 00226 * \returns 0 on success, MBEDTLS_ERR_MD_BAD_INPUT_DATA if parameter 00227 * verification fails. 00228 */ 00229 int mbedtls_md_update( mbedtls_md_context_t *ctx, const unsigned char *input, size_t ilen ); 00230 00231 /** 00232 * \brief Generic message digest final digest 00233 * Called after mbedtls_md_update(). 00234 * Usually followed by mbedtls_md_free() or mbedtls_md_starts(). 00235 * 00236 * \param ctx Generic message digest context 00237 * \param output Generic message digest checksum result 00238 * 00239 * \returns 0 on success, MBEDTLS_ERR_MD_BAD_INPUT_DATA if parameter 00240 * verification fails. 00241 */ 00242 int mbedtls_md_finish( mbedtls_md_context_t *ctx, unsigned char *output ); 00243 00244 /** 00245 * \brief Output = message_digest( input buffer ) 00246 * 00247 * \param md_info message digest info 00248 * \param input buffer holding the data 00249 * \param ilen length of the input data 00250 * \param output Generic message digest checksum result 00251 * 00252 * \returns 0 on success, MBEDTLS_ERR_MD_BAD_INPUT_DATA if parameter 00253 * verification fails. 00254 */ 00255 int mbedtls_md( const mbedtls_md_info_t *md_info, const unsigned char *input, size_t ilen, 00256 unsigned char *output ); 00257 00258 #if defined(MBEDTLS_FS_IO) 00259 /** 00260 * \brief Output = message_digest( file contents ) 00261 * 00262 * \param md_info message digest info 00263 * \param path input file name 00264 * \param output generic message digest checksum result 00265 * 00266 * \return 0 if successful, 00267 * MBEDTLS_ERR_MD_FILE_IO_ERROR if file input failed, 00268 * MBEDTLS_ERR_MD_BAD_INPUT_DATA if md_info was NULL. 00269 */ 00270 int mbedtls_md_file( const mbedtls_md_info_t *md_info, const char *path, 00271 unsigned char *output ); 00272 #endif /* MBEDTLS_FS_IO */ 00273 00274 /** 00275 * \brief Set HMAC key and prepare to authenticate a new message. 00276 * Usually called after mbedtls_md_setup() or mbedtls_md_hmac_finish(). 00277 * 00278 * \param ctx HMAC context 00279 * \param key HMAC secret key 00280 * \param keylen length of the HMAC key in bytes 00281 * 00282 * \returns 0 on success, MBEDTLS_ERR_MD_BAD_INPUT_DATA if parameter 00283 * verification fails. 00284 */ 00285 int mbedtls_md_hmac_starts( mbedtls_md_context_t *ctx, const unsigned char *key, 00286 size_t keylen ); 00287 00288 /** 00289 * \brief Generic HMAC process buffer. 00290 * Called between mbedtls_md_hmac_starts() or mbedtls_md_hmac_reset() 00291 * and mbedtls_md_hmac_finish(). 00292 * May be called repeatedly. 00293 * 00294 * \param ctx HMAC context 00295 * \param input buffer holding the data 00296 * \param ilen length of the input data 00297 * 00298 * \returns 0 on success, MBEDTLS_ERR_MD_BAD_INPUT_DATA if parameter 00299 * verification fails. 00300 */ 00301 int mbedtls_md_hmac_update( mbedtls_md_context_t *ctx, const unsigned char *input, 00302 size_t ilen ); 00303 00304 /** 00305 * \brief Output HMAC. 00306 * Called after mbedtls_md_hmac_update(). 00307 * Usually followed my mbedtls_md_hmac_reset(), mbedtls_md_hmac_starts(), 00308 * or mbedtls_md_free(). 00309 * 00310 * \param ctx HMAC context 00311 * \param output Generic HMAC checksum result 00312 * 00313 * \returns 0 on success, MBEDTLS_ERR_MD_BAD_INPUT_DATA if parameter 00314 * verification fails. 00315 */ 00316 int mbedtls_md_hmac_finish( mbedtls_md_context_t *ctx, unsigned char *output); 00317 00318 /** 00319 * \brief Prepare to authenticate a new message with the same key. 00320 * Called after mbedtls_md_hmac_finish() and before mbedtls_md_hmac_update(). 00321 * 00322 * \param ctx HMAC context to be reset 00323 * 00324 * \returns 0 on success, MBEDTLS_ERR_MD_BAD_INPUT_DATA if parameter 00325 * verification fails. 00326 */ 00327 int mbedtls_md_hmac_reset( mbedtls_md_context_t *ctx ); 00328 00329 /** 00330 * \brief Output = Generic_HMAC( hmac key, input buffer ) 00331 * 00332 * \param md_info message digest info 00333 * \param key HMAC secret key 00334 * \param keylen length of the HMAC key in bytes 00335 * \param input buffer holding the data 00336 * \param ilen length of the input data 00337 * \param output Generic HMAC-result 00338 * 00339 * \returns 0 on success, MBEDTLS_ERR_MD_BAD_INPUT_DATA if parameter 00340 * verification fails. 00341 */ 00342 int mbedtls_md_hmac( const mbedtls_md_info_t *md_info, const unsigned char *key, size_t keylen, 00343 const unsigned char *input, size_t ilen, 00344 unsigned char *output ); 00345 00346 /* Internal use */ 00347 int mbedtls_md_process( mbedtls_md_context_t *ctx, const unsigned char *data ); 00348 00349 #ifdef __cplusplus 00350 } 00351 #endif 00352 00353 #endif /* MBEDTLS_MD_H */
Generated on Tue Jul 12 2022 12:52:43 by
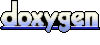