mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
hmac_drbg.h
Go to the documentation of this file.
00001 /** 00002 * \file hmac_drbg.h 00003 * 00004 * \brief HMAC_DRBG (NIST SP 800-90A) 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_HMAC_DRBG_H 00024 #define MBEDTLS_HMAC_DRBG_H 00025 00026 #include "md.h" 00027 00028 #if defined(MBEDTLS_THREADING_C) 00029 #include "mbedtls/threading.h" 00030 #endif 00031 00032 /* 00033 * Error codes 00034 */ 00035 #define MBEDTLS_ERR_HMAC_DRBG_REQUEST_TOO_BIG -0x0003 /**< Too many random requested in single call. */ 00036 #define MBEDTLS_ERR_HMAC_DRBG_INPUT_TOO_BIG -0x0005 /**< Input too large (Entropy + additional). */ 00037 #define MBEDTLS_ERR_HMAC_DRBG_FILE_IO_ERROR -0x0007 /**< Read/write error in file. */ 00038 #define MBEDTLS_ERR_HMAC_DRBG_ENTROPY_SOURCE_FAILED -0x0009 /**< The entropy source failed. */ 00039 00040 /** 00041 * \name SECTION: Module settings 00042 * 00043 * The configuration options you can set for this module are in this section. 00044 * Either change them in config.h or define them on the compiler command line. 00045 * \{ 00046 */ 00047 00048 #if !defined(MBEDTLS_HMAC_DRBG_RESEED_INTERVAL) 00049 #define MBEDTLS_HMAC_DRBG_RESEED_INTERVAL 10000 /**< Interval before reseed is performed by default */ 00050 #endif 00051 00052 #if !defined(MBEDTLS_HMAC_DRBG_MAX_INPUT) 00053 #define MBEDTLS_HMAC_DRBG_MAX_INPUT 256 /**< Maximum number of additional input bytes */ 00054 #endif 00055 00056 #if !defined(MBEDTLS_HMAC_DRBG_MAX_REQUEST) 00057 #define MBEDTLS_HMAC_DRBG_MAX_REQUEST 1024 /**< Maximum number of requested bytes per call */ 00058 #endif 00059 00060 #if !defined(MBEDTLS_HMAC_DRBG_MAX_SEED_INPUT) 00061 #define MBEDTLS_HMAC_DRBG_MAX_SEED_INPUT 384 /**< Maximum size of (re)seed buffer */ 00062 #endif 00063 00064 /* \} name SECTION: Module settings */ 00065 00066 #define MBEDTLS_HMAC_DRBG_PR_OFF 0 /**< No prediction resistance */ 00067 #define MBEDTLS_HMAC_DRBG_PR_ON 1 /**< Prediction resistance enabled */ 00068 00069 #ifdef __cplusplus 00070 extern "C" { 00071 #endif 00072 00073 /** 00074 * HMAC_DRBG context. 00075 */ 00076 typedef struct 00077 { 00078 /* Working state: the key K is not stored explicitely, 00079 * but is implied by the HMAC context */ 00080 mbedtls_md_context_t md_ctx ; /*!< HMAC context (inc. K) */ 00081 unsigned char V[MBEDTLS_MD_MAX_SIZE]; /*!< V in the spec */ 00082 int reseed_counter ; /*!< reseed counter */ 00083 00084 /* Administrative state */ 00085 size_t entropy_len ; /*!< entropy bytes grabbed on each (re)seed */ 00086 int prediction_resistance; /*!< enable prediction resistance (Automatic 00087 reseed before every random generation) */ 00088 int reseed_interval ; /*!< reseed interval */ 00089 00090 /* Callbacks */ 00091 int (*f_entropy)(void *, unsigned char *, size_t); /*!< entropy function */ 00092 void *p_entropy ; /*!< context for the entropy function */ 00093 00094 #if defined(MBEDTLS_THREADING_C) 00095 mbedtls_threading_mutex_t mutex; 00096 #endif 00097 } mbedtls_hmac_drbg_context; 00098 00099 /** 00100 * \brief HMAC_DRBG context initialization 00101 * Makes the context ready for mbedtls_hmac_drbg_seed(), 00102 * mbedtls_hmac_drbg_seed_buf() or 00103 * mbedtls_hmac_drbg_free(). 00104 * 00105 * \param ctx HMAC_DRBG context to be initialized 00106 */ 00107 void mbedtls_hmac_drbg_init( mbedtls_hmac_drbg_context *ctx ); 00108 00109 /** 00110 * \brief HMAC_DRBG initial seeding 00111 * Seed and setup entropy source for future reseeds. 00112 * 00113 * \param ctx HMAC_DRBG context to be seeded 00114 * \param md_info MD algorithm to use for HMAC_DRBG 00115 * \param f_entropy Entropy callback (p_entropy, buffer to fill, buffer 00116 * length) 00117 * \param p_entropy Entropy context 00118 * \param custom Personalization data (Device specific identifiers) 00119 * (Can be NULL) 00120 * \param len Length of personalization data 00121 * 00122 * \note The "security strength" as defined by NIST is set to: 00123 * 128 bits if md_alg is SHA-1, 00124 * 192 bits if md_alg is SHA-224, 00125 * 256 bits if md_alg is SHA-256 or higher. 00126 * Note that SHA-256 is just as efficient as SHA-224. 00127 * 00128 * \return 0 if successful, or 00129 * MBEDTLS_ERR_MD_BAD_INPUT_DATA, or 00130 * MBEDTLS_ERR_MD_ALLOC_FAILED, or 00131 * MBEDTLS_ERR_HMAC_DRBG_ENTROPY_SOURCE_FAILED. 00132 */ 00133 int mbedtls_hmac_drbg_seed( mbedtls_hmac_drbg_context *ctx, 00134 const mbedtls_md_info_t * md_info, 00135 int (*f_entropy)(void *, unsigned char *, size_t), 00136 void *p_entropy, 00137 const unsigned char *custom, 00138 size_t len ); 00139 00140 /** 00141 * \brief Initilisation of simpified HMAC_DRBG (never reseeds). 00142 * (For use with deterministic ECDSA.) 00143 * 00144 * \param ctx HMAC_DRBG context to be initialised 00145 * \param md_info MD algorithm to use for HMAC_DRBG 00146 * \param data Concatenation of entropy string and additional data 00147 * \param data_len Length of data in bytes 00148 * 00149 * \return 0 if successful, or 00150 * MBEDTLS_ERR_MD_BAD_INPUT_DATA, or 00151 * MBEDTLS_ERR_MD_ALLOC_FAILED. 00152 */ 00153 int mbedtls_hmac_drbg_seed_buf( mbedtls_hmac_drbg_context *ctx, 00154 const mbedtls_md_info_t * md_info, 00155 const unsigned char *data, size_t data_len ); 00156 00157 /** 00158 * \brief Enable / disable prediction resistance (Default: Off) 00159 * 00160 * Note: If enabled, entropy is used for ctx->entropy_len before each call! 00161 * Only use this if you have ample supply of good entropy! 00162 * 00163 * \param ctx HMAC_DRBG context 00164 * \param resistance MBEDTLS_HMAC_DRBG_PR_ON or MBEDTLS_HMAC_DRBG_PR_OFF 00165 */ 00166 void mbedtls_hmac_drbg_set_prediction_resistance( mbedtls_hmac_drbg_context *ctx, 00167 int resistance ); 00168 00169 /** 00170 * \brief Set the amount of entropy grabbed on each reseed 00171 * (Default: given by the security strength, which 00172 * depends on the hash used, see \c mbedtls_hmac_drbg_init() ) 00173 * 00174 * \param ctx HMAC_DRBG context 00175 * \param len Amount of entropy to grab, in bytes 00176 */ 00177 void mbedtls_hmac_drbg_set_entropy_len( mbedtls_hmac_drbg_context *ctx, 00178 size_t len ); 00179 00180 /** 00181 * \brief Set the reseed interval 00182 * (Default: MBEDTLS_HMAC_DRBG_RESEED_INTERVAL) 00183 * 00184 * \param ctx HMAC_DRBG context 00185 * \param interval Reseed interval 00186 */ 00187 void mbedtls_hmac_drbg_set_reseed_interval( mbedtls_hmac_drbg_context *ctx, 00188 int interval ); 00189 00190 /** 00191 * \brief HMAC_DRBG update state 00192 * 00193 * \param ctx HMAC_DRBG context 00194 * \param additional Additional data to update state with, or NULL 00195 * \param add_len Length of additional data, or 0 00196 * 00197 * \note Additional data is optional, pass NULL and 0 as second 00198 * third argument if no additional data is being used. 00199 */ 00200 void mbedtls_hmac_drbg_update( mbedtls_hmac_drbg_context *ctx, 00201 const unsigned char *additional, size_t add_len ); 00202 00203 /** 00204 * \brief HMAC_DRBG reseeding (extracts data from entropy source) 00205 * 00206 * \param ctx HMAC_DRBG context 00207 * \param additional Additional data to add to state (Can be NULL) 00208 * \param len Length of additional data 00209 * 00210 * \return 0 if successful, or 00211 * MBEDTLS_ERR_HMAC_DRBG_ENTROPY_SOURCE_FAILED 00212 */ 00213 int mbedtls_hmac_drbg_reseed( mbedtls_hmac_drbg_context *ctx, 00214 const unsigned char *additional, size_t len ); 00215 00216 /** 00217 * \brief HMAC_DRBG generate random with additional update input 00218 * 00219 * Note: Automatically reseeds if reseed_counter is reached or PR is enabled. 00220 * 00221 * \param p_rng HMAC_DRBG context 00222 * \param output Buffer to fill 00223 * \param output_len Length of the buffer 00224 * \param additional Additional data to update with (can be NULL) 00225 * \param add_len Length of additional data (can be 0) 00226 * 00227 * \return 0 if successful, or 00228 * MBEDTLS_ERR_HMAC_DRBG_ENTROPY_SOURCE_FAILED, or 00229 * MBEDTLS_ERR_HMAC_DRBG_REQUEST_TOO_BIG, or 00230 * MBEDTLS_ERR_HMAC_DRBG_INPUT_TOO_BIG. 00231 */ 00232 int mbedtls_hmac_drbg_random_with_add( void *p_rng, 00233 unsigned char *output, size_t output_len, 00234 const unsigned char *additional, 00235 size_t add_len ); 00236 00237 /** 00238 * \brief HMAC_DRBG generate random 00239 * 00240 * Note: Automatically reseeds if reseed_counter is reached or PR is enabled. 00241 * 00242 * \param p_rng HMAC_DRBG context 00243 * \param output Buffer to fill 00244 * \param out_len Length of the buffer 00245 * 00246 * \return 0 if successful, or 00247 * MBEDTLS_ERR_HMAC_DRBG_ENTROPY_SOURCE_FAILED, or 00248 * MBEDTLS_ERR_HMAC_DRBG_REQUEST_TOO_BIG 00249 */ 00250 int mbedtls_hmac_drbg_random( void *p_rng, unsigned char *output, size_t out_len ); 00251 00252 /** 00253 * \brief Free an HMAC_DRBG context 00254 * 00255 * \param ctx HMAC_DRBG context to free. 00256 */ 00257 void mbedtls_hmac_drbg_free( mbedtls_hmac_drbg_context *ctx ); 00258 00259 #if defined(MBEDTLS_FS_IO) 00260 /** 00261 * \brief Write a seed file 00262 * 00263 * \param ctx HMAC_DRBG context 00264 * \param path Name of the file 00265 * 00266 * \return 0 if successful, 1 on file error, or 00267 * MBEDTLS_ERR_HMAC_DRBG_ENTROPY_SOURCE_FAILED 00268 */ 00269 int mbedtls_hmac_drbg_write_seed_file( mbedtls_hmac_drbg_context *ctx, const char *path ); 00270 00271 /** 00272 * \brief Read and update a seed file. Seed is added to this 00273 * instance 00274 * 00275 * \param ctx HMAC_DRBG context 00276 * \param path Name of the file 00277 * 00278 * \return 0 if successful, 1 on file error, 00279 * MBEDTLS_ERR_HMAC_DRBG_ENTROPY_SOURCE_FAILED or 00280 * MBEDTLS_ERR_HMAC_DRBG_INPUT_TOO_BIG 00281 */ 00282 int mbedtls_hmac_drbg_update_seed_file( mbedtls_hmac_drbg_context *ctx, const char *path ); 00283 #endif /* MBEDTLS_FS_IO */ 00284 00285 00286 #if defined(MBEDTLS_SELF_TEST) 00287 /** 00288 * \brief Checkup routine 00289 * 00290 * \return 0 if successful, or 1 if the test failed 00291 */ 00292 int mbedtls_hmac_drbg_self_test( int verbose ); 00293 #endif 00294 00295 #ifdef __cplusplus 00296 } 00297 #endif 00298 00299 #endif /* hmac_drbg.h */
Generated on Tue Jul 12 2022 12:52:43 by
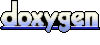