mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
error.h
Go to the documentation of this file.
00001 /** 00002 * \file error.h 00003 * 00004 * \brief Error to string translation 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_ERROR_H 00024 #define MBEDTLS_ERROR_H 00025 00026 #include <stddef.h> 00027 00028 /** 00029 * Error code layout. 00030 * 00031 * Currently we try to keep all error codes within the negative space of 16 00032 * bits signed integers to support all platforms (-0x0001 - -0x7FFF). In 00033 * addition we'd like to give two layers of information on the error if 00034 * possible. 00035 * 00036 * For that purpose the error codes are segmented in the following manner: 00037 * 00038 * 16 bit error code bit-segmentation 00039 * 00040 * 1 bit - Unused (sign bit) 00041 * 3 bits - High level module ID 00042 * 5 bits - Module-dependent error code 00043 * 7 bits - Low level module errors 00044 * 00045 * For historical reasons, low-level error codes are divided in even and odd, 00046 * even codes were assigned first, and -1 is reserved for other errors. 00047 * 00048 * Low-level module errors (0x0002-0x007E, 0x0003-0x007F) 00049 * 00050 * Module Nr Codes assigned 00051 * MPI 7 0x0002-0x0010 00052 * GCM 2 0x0012-0x0014 00053 * BLOWFISH 2 0x0016-0x0018 00054 * THREADING 3 0x001A-0x001E 00055 * AES 2 0x0020-0x0022 00056 * CAMELLIA 2 0x0024-0x0026 00057 * XTEA 1 0x0028-0x0028 00058 * BASE64 2 0x002A-0x002C 00059 * OID 1 0x002E-0x002E 0x000B-0x000B 00060 * PADLOCK 1 0x0030-0x0030 00061 * DES 1 0x0032-0x0032 00062 * CTR_DBRG 4 0x0034-0x003A 00063 * ENTROPY 3 0x003C-0x0040 0x003D-0x003F 00064 * NET 11 0x0042-0x0052 0x0043-0x0045 00065 * ASN1 7 0x0060-0x006C 00066 * PBKDF2 1 0x007C-0x007C 00067 * HMAC_DRBG 4 0x0003-0x0009 00068 * CCM 2 0x000D-0x000F 00069 * 00070 * High-level module nr (3 bits - 0x0...-0x7...) 00071 * Name ID Nr of Errors 00072 * PEM 1 9 00073 * PKCS#12 1 4 (Started from top) 00074 * X509 2 19 00075 * PKCS5 2 4 (Started from top) 00076 * DHM 3 9 00077 * PK 3 14 (Started from top) 00078 * RSA 4 9 00079 * ECP 4 8 (Started from top) 00080 * MD 5 4 00081 * CIPHER 6 6 00082 * SSL 6 16 (Started from top) 00083 * SSL 7 31 00084 * 00085 * Module dependent error code (5 bits 0x.00.-0x.F8.) 00086 */ 00087 00088 #ifdef __cplusplus 00089 extern "C" { 00090 #endif 00091 00092 /** 00093 * \brief Translate a mbed TLS error code into a string representation, 00094 * Result is truncated if necessary and always includes a terminating 00095 * null byte. 00096 * 00097 * \param errnum error code 00098 * \param buffer buffer to place representation in 00099 * \param buflen length of the buffer 00100 */ 00101 void mbedtls_strerror( int errnum, char *buffer, size_t buflen ); 00102 00103 #ifdef __cplusplus 00104 } 00105 #endif 00106 00107 #endif /* error.h */
Generated on Tue Jul 12 2022 12:52:43 by
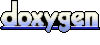