mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
cipher.h
Go to the documentation of this file.
00001 /** 00002 * \file cipher.h 00003 * 00004 * \brief Generic cipher wrapper. 00005 * 00006 * \author Adriaan de Jong <dejong@fox-it.com> 00007 * 00008 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00009 * SPDX-License-Identifier: Apache-2.0 00010 * 00011 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00012 * not use this file except in compliance with the License. 00013 * You may obtain a copy of the License at 00014 * 00015 * http://www.apache.org/licenses/LICENSE-2.0 00016 * 00017 * Unless required by applicable law or agreed to in writing, software 00018 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00019 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00020 * See the License for the specific language governing permissions and 00021 * limitations under the License. 00022 * 00023 * This file is part of mbed TLS (https://tls.mbed.org) 00024 */ 00025 00026 #ifndef MBEDTLS_CIPHER_H 00027 #define MBEDTLS_CIPHER_H 00028 00029 #if !defined(MBEDTLS_CONFIG_FILE) 00030 #include "config.h" 00031 #else 00032 #include MBEDTLS_CONFIG_FILE 00033 #endif 00034 00035 #include <stddef.h> 00036 00037 #if defined(MBEDTLS_GCM_C) || defined(MBEDTLS_CCM_C) 00038 #define MBEDTLS_CIPHER_MODE_AEAD 00039 #endif 00040 00041 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00042 #define MBEDTLS_CIPHER_MODE_WITH_PADDING 00043 #endif 00044 00045 #if defined(MBEDTLS_ARC4_C) 00046 #define MBEDTLS_CIPHER_MODE_STREAM 00047 #endif 00048 00049 #if ( defined(__ARMCC_VERSION) || defined(_MSC_VER) ) && \ 00050 !defined(inline) && !defined(__cplusplus) 00051 #define inline __inline 00052 #endif 00053 00054 #define MBEDTLS_ERR_CIPHER_FEATURE_UNAVAILABLE -0x6080 /**< The selected feature is not available. */ 00055 #define MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA -0x6100 /**< Bad input parameters to function. */ 00056 #define MBEDTLS_ERR_CIPHER_ALLOC_FAILED -0x6180 /**< Failed to allocate memory. */ 00057 #define MBEDTLS_ERR_CIPHER_INVALID_PADDING -0x6200 /**< Input data contains invalid padding and is rejected. */ 00058 #define MBEDTLS_ERR_CIPHER_FULL_BLOCK_EXPECTED -0x6280 /**< Decryption of block requires a full block. */ 00059 #define MBEDTLS_ERR_CIPHER_AUTH_FAILED -0x6300 /**< Authentication failed (for AEAD modes). */ 00060 00061 #define MBEDTLS_CIPHER_VARIABLE_IV_LEN 0x01 /**< Cipher accepts IVs of variable length */ 00062 #define MBEDTLS_CIPHER_VARIABLE_KEY_LEN 0x02 /**< Cipher accepts keys of variable length */ 00063 00064 #ifdef __cplusplus 00065 extern "C" { 00066 #endif 00067 00068 typedef enum { 00069 MBEDTLS_CIPHER_ID_NONE = 0, 00070 MBEDTLS_CIPHER_ID_NULL, 00071 MBEDTLS_CIPHER_ID_AES, 00072 MBEDTLS_CIPHER_ID_DES, 00073 MBEDTLS_CIPHER_ID_3DES, 00074 MBEDTLS_CIPHER_ID_CAMELLIA, 00075 MBEDTLS_CIPHER_ID_BLOWFISH, 00076 MBEDTLS_CIPHER_ID_ARC4, 00077 } mbedtls_cipher_id_t; 00078 00079 typedef enum { 00080 MBEDTLS_CIPHER_NONE = 0, 00081 MBEDTLS_CIPHER_NULL, 00082 MBEDTLS_CIPHER_AES_128_ECB, 00083 MBEDTLS_CIPHER_AES_192_ECB, 00084 MBEDTLS_CIPHER_AES_256_ECB, 00085 MBEDTLS_CIPHER_AES_128_CBC, 00086 MBEDTLS_CIPHER_AES_192_CBC, 00087 MBEDTLS_CIPHER_AES_256_CBC, 00088 MBEDTLS_CIPHER_AES_128_CFB128, 00089 MBEDTLS_CIPHER_AES_192_CFB128, 00090 MBEDTLS_CIPHER_AES_256_CFB128, 00091 MBEDTLS_CIPHER_AES_128_CTR, 00092 MBEDTLS_CIPHER_AES_192_CTR, 00093 MBEDTLS_CIPHER_AES_256_CTR, 00094 MBEDTLS_CIPHER_AES_128_GCM, 00095 MBEDTLS_CIPHER_AES_192_GCM, 00096 MBEDTLS_CIPHER_AES_256_GCM, 00097 MBEDTLS_CIPHER_CAMELLIA_128_ECB, 00098 MBEDTLS_CIPHER_CAMELLIA_192_ECB, 00099 MBEDTLS_CIPHER_CAMELLIA_256_ECB, 00100 MBEDTLS_CIPHER_CAMELLIA_128_CBC, 00101 MBEDTLS_CIPHER_CAMELLIA_192_CBC, 00102 MBEDTLS_CIPHER_CAMELLIA_256_CBC, 00103 MBEDTLS_CIPHER_CAMELLIA_128_CFB128, 00104 MBEDTLS_CIPHER_CAMELLIA_192_CFB128, 00105 MBEDTLS_CIPHER_CAMELLIA_256_CFB128, 00106 MBEDTLS_CIPHER_CAMELLIA_128_CTR, 00107 MBEDTLS_CIPHER_CAMELLIA_192_CTR, 00108 MBEDTLS_CIPHER_CAMELLIA_256_CTR, 00109 MBEDTLS_CIPHER_CAMELLIA_128_GCM, 00110 MBEDTLS_CIPHER_CAMELLIA_192_GCM, 00111 MBEDTLS_CIPHER_CAMELLIA_256_GCM, 00112 MBEDTLS_CIPHER_DES_ECB, 00113 MBEDTLS_CIPHER_DES_CBC, 00114 MBEDTLS_CIPHER_DES_EDE_ECB, 00115 MBEDTLS_CIPHER_DES_EDE_CBC, 00116 MBEDTLS_CIPHER_DES_EDE3_ECB, 00117 MBEDTLS_CIPHER_DES_EDE3_CBC, 00118 MBEDTLS_CIPHER_BLOWFISH_ECB, 00119 MBEDTLS_CIPHER_BLOWFISH_CBC, 00120 MBEDTLS_CIPHER_BLOWFISH_CFB64, 00121 MBEDTLS_CIPHER_BLOWFISH_CTR, 00122 MBEDTLS_CIPHER_ARC4_128, 00123 MBEDTLS_CIPHER_AES_128_CCM, 00124 MBEDTLS_CIPHER_AES_192_CCM, 00125 MBEDTLS_CIPHER_AES_256_CCM, 00126 MBEDTLS_CIPHER_CAMELLIA_128_CCM, 00127 MBEDTLS_CIPHER_CAMELLIA_192_CCM, 00128 MBEDTLS_CIPHER_CAMELLIA_256_CCM, 00129 } mbedtls_cipher_type_t; 00130 00131 typedef enum { 00132 MBEDTLS_MODE_NONE = 0, 00133 MBEDTLS_MODE_ECB, 00134 MBEDTLS_MODE_CBC, 00135 MBEDTLS_MODE_CFB, 00136 MBEDTLS_MODE_OFB, /* Unused! */ 00137 MBEDTLS_MODE_CTR, 00138 MBEDTLS_MODE_GCM, 00139 MBEDTLS_MODE_STREAM, 00140 MBEDTLS_MODE_CCM, 00141 } mbedtls_cipher_mode_t; 00142 00143 typedef enum { 00144 MBEDTLS_PADDING_PKCS7 = 0, /**< PKCS7 padding (default) */ 00145 MBEDTLS_PADDING_ONE_AND_ZEROS, /**< ISO/IEC 7816-4 padding */ 00146 MBEDTLS_PADDING_ZEROS_AND_LEN, /**< ANSI X.923 padding */ 00147 MBEDTLS_PADDING_ZEROS, /**< zero padding (not reversible!) */ 00148 MBEDTLS_PADDING_NONE, /**< never pad (full blocks only) */ 00149 } mbedtls_cipher_padding_t ; 00150 00151 typedef enum { 00152 MBEDTLS_OPERATION_NONE = -1, 00153 MBEDTLS_DECRYPT = 0, 00154 MBEDTLS_ENCRYPT, 00155 } mbedtls_operation_t; 00156 00157 enum { 00158 /** Undefined key length */ 00159 MBEDTLS_KEY_LENGTH_NONE = 0, 00160 /** Key length, in bits (including parity), for DES keys */ 00161 MBEDTLS_KEY_LENGTH_DES = 64, 00162 /** Key length, in bits (including parity), for DES in two key EDE */ 00163 MBEDTLS_KEY_LENGTH_DES_EDE = 128, 00164 /** Key length, in bits (including parity), for DES in three-key EDE */ 00165 MBEDTLS_KEY_LENGTH_DES_EDE3 = 192, 00166 }; 00167 00168 /** Maximum length of any IV, in bytes */ 00169 #define MBEDTLS_MAX_IV_LENGTH 16 00170 /** Maximum block size of any cipher, in bytes */ 00171 #define MBEDTLS_MAX_BLOCK_LENGTH 16 00172 00173 /** 00174 * Base cipher information (opaque struct). 00175 */ 00176 typedef struct mbedtls_cipher_base_t mbedtls_cipher_base_t; 00177 00178 /** 00179 * Cipher information. Allows cipher functions to be called in a generic way. 00180 */ 00181 typedef struct { 00182 /** Full cipher identifier (e.g. MBEDTLS_CIPHER_AES_256_CBC) */ 00183 mbedtls_cipher_type_t type; 00184 00185 /** Cipher mode (e.g. MBEDTLS_MODE_CBC) */ 00186 mbedtls_cipher_mode_t mode; 00187 00188 /** Cipher key length, in bits (default length for variable sized ciphers) 00189 * (Includes parity bits for ciphers like DES) */ 00190 unsigned int key_bitlen; 00191 00192 /** Name of the cipher */ 00193 const char * name; 00194 00195 /** IV/NONCE size, in bytes. 00196 * For cipher that accept many sizes: recommended size */ 00197 unsigned int iv_size; 00198 00199 /** Flags for variable IV size, variable key size, etc. */ 00200 int flags; 00201 00202 /** block size, in bytes */ 00203 unsigned int block_size; 00204 00205 /** Base cipher information and functions */ 00206 const mbedtls_cipher_base_t *base; 00207 00208 } mbedtls_cipher_info_t; 00209 00210 /** 00211 * Generic cipher context. 00212 */ 00213 typedef struct { 00214 /** Information about the associated cipher */ 00215 const mbedtls_cipher_info_t *cipher_info; 00216 00217 /** Key length to use */ 00218 int key_bitlen; 00219 00220 /** Operation that the context's key has been initialised for */ 00221 mbedtls_operation_t operation; 00222 00223 #if defined(MBEDTLS_CIPHER_MODE_WITH_PADDING) 00224 /** Padding functions to use, if relevant for cipher mode */ 00225 void (*add_padding)( unsigned char *output, size_t olen, size_t data_len ); 00226 int (*get_padding)( unsigned char *input, size_t ilen, size_t *data_len ); 00227 #endif 00228 00229 /** Buffer for data that hasn't been encrypted yet */ 00230 unsigned char unprocessed_data[MBEDTLS_MAX_BLOCK_LENGTH]; 00231 00232 /** Number of bytes that still need processing */ 00233 size_t unprocessed_len; 00234 00235 /** Current IV or NONCE_COUNTER for CTR-mode */ 00236 unsigned char iv[MBEDTLS_MAX_IV_LENGTH]; 00237 00238 /** IV size in bytes (for ciphers with variable-length IVs) */ 00239 size_t iv_size; 00240 00241 /** Cipher-specific context */ 00242 void *cipher_ctx; 00243 } mbedtls_cipher_context_t; 00244 00245 /** 00246 * \brief Returns the list of ciphers supported by the generic cipher module. 00247 * 00248 * \return a statically allocated array of ciphers, the last entry 00249 * is 0. 00250 */ 00251 const int *mbedtls_cipher_list( void ); 00252 00253 /** 00254 * \brief Returns the cipher information structure associated 00255 * with the given cipher name. 00256 * 00257 * \param cipher_name Name of the cipher to search for. 00258 * 00259 * \return the cipher information structure associated with the 00260 * given cipher_name, or NULL if not found. 00261 */ 00262 const mbedtls_cipher_info_t *mbedtls_cipher_info_from_string( const char *cipher_name ); 00263 00264 /** 00265 * \brief Returns the cipher information structure associated 00266 * with the given cipher type. 00267 * 00268 * \param cipher_type Type of the cipher to search for. 00269 * 00270 * \return the cipher information structure associated with the 00271 * given cipher_type, or NULL if not found. 00272 */ 00273 const mbedtls_cipher_info_t *mbedtls_cipher_info_from_type( const mbedtls_cipher_type_t cipher_type ); 00274 00275 /** 00276 * \brief Returns the cipher information structure associated 00277 * with the given cipher id, key size and mode. 00278 * 00279 * \param cipher_id Id of the cipher to search for 00280 * (e.g. MBEDTLS_CIPHER_ID_AES) 00281 * \param key_bitlen Length of the key in bits 00282 * \param mode Cipher mode (e.g. MBEDTLS_MODE_CBC) 00283 * 00284 * \return the cipher information structure associated with the 00285 * given cipher_type, or NULL if not found. 00286 */ 00287 const mbedtls_cipher_info_t *mbedtls_cipher_info_from_values( const mbedtls_cipher_id_t cipher_id, 00288 int key_bitlen, 00289 const mbedtls_cipher_mode_t mode ); 00290 00291 /** 00292 * \brief Initialize a cipher_context (as NONE) 00293 */ 00294 void mbedtls_cipher_init( mbedtls_cipher_context_t *ctx ); 00295 00296 /** 00297 * \brief Free and clear the cipher-specific context of ctx. 00298 * Freeing ctx itself remains the responsibility of the 00299 * caller. 00300 */ 00301 void mbedtls_cipher_free( mbedtls_cipher_context_t *ctx ); 00302 00303 /** 00304 * \brief Initialises and fills the cipher context structure with 00305 * the appropriate values. 00306 * 00307 * \note Currently also clears structure. In future versions you 00308 * will be required to call mbedtls_cipher_init() on the structure 00309 * first. 00310 * 00311 * \param ctx context to initialise. May not be NULL. 00312 * \param cipher_info cipher to use. 00313 * 00314 * \return 0 on success, 00315 * MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA on parameter failure, 00316 * MBEDTLS_ERR_CIPHER_ALLOC_FAILED if allocation of the 00317 * cipher-specific context failed. 00318 */ 00319 int mbedtls_cipher_setup( mbedtls_cipher_context_t *ctx, const mbedtls_cipher_info_t *cipher_info ); 00320 00321 /** 00322 * \brief Returns the block size of the given cipher. 00323 * 00324 * \param ctx cipher's context. Must have been initialised. 00325 * 00326 * \return size of the cipher's blocks, or 0 if ctx has not been 00327 * initialised. 00328 */ 00329 static inline unsigned int mbedtls_cipher_get_block_size( const mbedtls_cipher_context_t *ctx ) 00330 { 00331 if( NULL == ctx || NULL == ctx->cipher_info ) 00332 return 0; 00333 00334 return ctx->cipher_info->block_size; 00335 } 00336 00337 /** 00338 * \brief Returns the mode of operation for the cipher. 00339 * (e.g. MBEDTLS_MODE_CBC) 00340 * 00341 * \param ctx cipher's context. Must have been initialised. 00342 * 00343 * \return mode of operation, or MBEDTLS_MODE_NONE if ctx 00344 * has not been initialised. 00345 */ 00346 static inline mbedtls_cipher_mode_t mbedtls_cipher_get_cipher_mode( const mbedtls_cipher_context_t *ctx ) 00347 { 00348 if( NULL == ctx || NULL == ctx->cipher_info ) 00349 return MBEDTLS_MODE_NONE; 00350 00351 return ctx->cipher_info->mode; 00352 } 00353 00354 /** 00355 * \brief Returns the size of the cipher's IV/NONCE in bytes. 00356 * 00357 * \param ctx cipher's context. Must have been initialised. 00358 * 00359 * \return If IV has not been set yet: (recommended) IV size 00360 * (0 for ciphers not using IV/NONCE). 00361 * If IV has already been set: actual size. 00362 */ 00363 static inline int mbedtls_cipher_get_iv_size( const mbedtls_cipher_context_t *ctx ) 00364 { 00365 if( NULL == ctx || NULL == ctx->cipher_info ) 00366 return 0; 00367 00368 if( ctx->iv_size != 0 ) 00369 return (int) ctx->iv_size; 00370 00371 return (int) ctx->cipher_info->iv_size; 00372 } 00373 00374 /** 00375 * \brief Returns the type of the given cipher. 00376 * 00377 * \param ctx cipher's context. Must have been initialised. 00378 * 00379 * \return type of the cipher, or MBEDTLS_CIPHER_NONE if ctx has 00380 * not been initialised. 00381 */ 00382 static inline mbedtls_cipher_type_t mbedtls_cipher_get_type( const mbedtls_cipher_context_t *ctx ) 00383 { 00384 if( NULL == ctx || NULL == ctx->cipher_info ) 00385 return MBEDTLS_CIPHER_NONE; 00386 00387 return ctx->cipher_info->type; 00388 } 00389 00390 /** 00391 * \brief Returns the name of the given cipher, as a string. 00392 * 00393 * \param ctx cipher's context. Must have been initialised. 00394 * 00395 * \return name of the cipher, or NULL if ctx was not initialised. 00396 */ 00397 static inline const char *mbedtls_cipher_get_name( const mbedtls_cipher_context_t *ctx ) 00398 { 00399 if( NULL == ctx || NULL == ctx->cipher_info ) 00400 return 0; 00401 00402 return ctx->cipher_info->name; 00403 } 00404 00405 /** 00406 * \brief Returns the key length of the cipher. 00407 * 00408 * \param ctx cipher's context. Must have been initialised. 00409 * 00410 * \return cipher's key length, in bits, or 00411 * MBEDTLS_KEY_LENGTH_NONE if ctx has not been 00412 * initialised. 00413 */ 00414 static inline int mbedtls_cipher_get_key_bitlen( const mbedtls_cipher_context_t *ctx ) 00415 { 00416 if( NULL == ctx || NULL == ctx->cipher_info ) 00417 return MBEDTLS_KEY_LENGTH_NONE; 00418 00419 return (int) ctx->cipher_info->key_bitlen; 00420 } 00421 00422 /** 00423 * \brief Returns the operation of the given cipher. 00424 * 00425 * \param ctx cipher's context. Must have been initialised. 00426 * 00427 * \return operation (MBEDTLS_ENCRYPT or MBEDTLS_DECRYPT), 00428 * or MBEDTLS_OPERATION_NONE if ctx has not been 00429 * initialised. 00430 */ 00431 static inline mbedtls_operation_t mbedtls_cipher_get_operation( const mbedtls_cipher_context_t *ctx ) 00432 { 00433 if( NULL == ctx || NULL == ctx->cipher_info ) 00434 return MBEDTLS_OPERATION_NONE; 00435 00436 return ctx->operation; 00437 } 00438 00439 /** 00440 * \brief Set the key to use with the given context. 00441 * 00442 * \param ctx generic cipher context. May not be NULL. Must have been 00443 * initialised using cipher_context_from_type or 00444 * cipher_context_from_string. 00445 * \param key The key to use. 00446 * \param key_bitlen key length to use, in bits. 00447 * \param operation Operation that the key will be used for, either 00448 * MBEDTLS_ENCRYPT or MBEDTLS_DECRYPT. 00449 * 00450 * \returns 0 on success, MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA if 00451 * parameter verification fails or a cipher specific 00452 * error code. 00453 */ 00454 int mbedtls_cipher_setkey( mbedtls_cipher_context_t *ctx, const unsigned char *key, 00455 int key_bitlen, const mbedtls_operation_t operation ); 00456 00457 #if defined(MBEDTLS_CIPHER_MODE_WITH_PADDING) 00458 /** 00459 * \brief Set padding mode, for cipher modes that use padding. 00460 * (Default: PKCS7 padding.) 00461 * 00462 * \param ctx generic cipher context 00463 * \param mode padding mode 00464 * 00465 * \returns 0 on success, MBEDTLS_ERR_CIPHER_FEATURE_UNAVAILABLE 00466 * if selected padding mode is not supported, or 00467 * MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA if the cipher mode 00468 * does not support padding. 00469 */ 00470 int mbedtls_cipher_set_padding_mode( mbedtls_cipher_context_t *ctx, mbedtls_cipher_padding_t mode ); 00471 #endif /* MBEDTLS_CIPHER_MODE_WITH_PADDING */ 00472 00473 /** 00474 * \brief Set the initialization vector (IV) or nonce 00475 * 00476 * \param ctx generic cipher context 00477 * \param iv IV to use (or NONCE_COUNTER for CTR-mode ciphers) 00478 * \param iv_len IV length for ciphers with variable-size IV; 00479 * discarded by ciphers with fixed-size IV. 00480 * 00481 * \returns 0 on success, or MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA 00482 * 00483 * \note Some ciphers don't use IVs nor NONCE. For these 00484 * ciphers, this function has no effect. 00485 */ 00486 int mbedtls_cipher_set_iv( mbedtls_cipher_context_t *ctx, 00487 const unsigned char *iv, size_t iv_len ); 00488 00489 /** 00490 * \brief Finish preparation of the given context 00491 * 00492 * \param ctx generic cipher context 00493 * 00494 * \returns 0 on success, MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA 00495 * if parameter verification fails. 00496 */ 00497 int mbedtls_cipher_reset( mbedtls_cipher_context_t *ctx ); 00498 00499 #if defined(MBEDTLS_GCM_C) 00500 /** 00501 * \brief Add additional data (for AEAD ciphers). 00502 * Currently only supported with GCM. 00503 * Must be called exactly once, after mbedtls_cipher_reset(). 00504 * 00505 * \param ctx generic cipher context 00506 * \param ad Additional data to use. 00507 * \param ad_len Length of ad. 00508 * 00509 * \return 0 on success, or a specific error code. 00510 */ 00511 int mbedtls_cipher_update_ad( mbedtls_cipher_context_t *ctx, 00512 const unsigned char *ad, size_t ad_len ); 00513 #endif /* MBEDTLS_GCM_C */ 00514 00515 /** 00516 * \brief Generic cipher update function. Encrypts/decrypts 00517 * using the given cipher context. Writes as many block 00518 * size'd blocks of data as possible to output. Any data 00519 * that cannot be written immediately will either be added 00520 * to the next block, or flushed when cipher_final is 00521 * called. 00522 * Exception: for MBEDTLS_MODE_ECB, expects single block 00523 * in size (e.g. 16 bytes for AES) 00524 * 00525 * \param ctx generic cipher context 00526 * \param input buffer holding the input data 00527 * \param ilen length of the input data 00528 * \param output buffer for the output data. Should be able to hold at 00529 * least ilen + block_size. Cannot be the same buffer as 00530 * input! 00531 * \param olen length of the output data, will be filled with the 00532 * actual number of bytes written. 00533 * 00534 * \returns 0 on success, MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA if 00535 * parameter verification fails, 00536 * MBEDTLS_ERR_CIPHER_FEATURE_UNAVAILABLE on an 00537 * unsupported mode for a cipher or a cipher specific 00538 * error code. 00539 * 00540 * \note If the underlying cipher is GCM, all calls to this 00541 * function, except the last one before mbedtls_cipher_finish(), 00542 * must have ilen a multiple of the block size. 00543 */ 00544 int mbedtls_cipher_update( mbedtls_cipher_context_t *ctx, const unsigned char *input, 00545 size_t ilen, unsigned char *output, size_t *olen ); 00546 00547 /** 00548 * \brief Generic cipher finalisation function. If data still 00549 * needs to be flushed from an incomplete block, data 00550 * contained within it will be padded with the size of 00551 * the last block, and written to the output buffer. 00552 * 00553 * \param ctx Generic cipher context 00554 * \param output buffer to write data to. Needs block_size available. 00555 * \param olen length of the data written to the output buffer. 00556 * 00557 * \returns 0 on success, MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA if 00558 * parameter verification fails, 00559 * MBEDTLS_ERR_CIPHER_FULL_BLOCK_EXPECTED if decryption 00560 * expected a full block but was not provided one, 00561 * MBEDTLS_ERR_CIPHER_INVALID_PADDING on invalid padding 00562 * while decrypting or a cipher specific error code. 00563 */ 00564 int mbedtls_cipher_finish( mbedtls_cipher_context_t *ctx, 00565 unsigned char *output, size_t *olen ); 00566 00567 #if defined(MBEDTLS_GCM_C) 00568 /** 00569 * \brief Write tag for AEAD ciphers. 00570 * Currently only supported with GCM. 00571 * Must be called after mbedtls_cipher_finish(). 00572 * 00573 * \param ctx Generic cipher context 00574 * \param tag buffer to write the tag 00575 * \param tag_len Length of the tag to write 00576 * 00577 * \return 0 on success, or a specific error code. 00578 */ 00579 int mbedtls_cipher_write_tag( mbedtls_cipher_context_t *ctx, 00580 unsigned char *tag, size_t tag_len ); 00581 00582 /** 00583 * \brief Check tag for AEAD ciphers. 00584 * Currently only supported with GCM. 00585 * Must be called after mbedtls_cipher_finish(). 00586 * 00587 * \param ctx Generic cipher context 00588 * \param tag Buffer holding the tag 00589 * \param tag_len Length of the tag to check 00590 * 00591 * \return 0 on success, or a specific error code. 00592 */ 00593 int mbedtls_cipher_check_tag( mbedtls_cipher_context_t *ctx, 00594 const unsigned char *tag, size_t tag_len ); 00595 #endif /* MBEDTLS_GCM_C */ 00596 00597 /** 00598 * \brief Generic all-in-one encryption/decryption 00599 * (for all ciphers except AEAD constructs). 00600 * 00601 * \param ctx generic cipher context 00602 * \param iv IV to use (or NONCE_COUNTER for CTR-mode ciphers) 00603 * \param iv_len IV length for ciphers with variable-size IV; 00604 * discarded by ciphers with fixed-size IV. 00605 * \param input buffer holding the input data 00606 * \param ilen length of the input data 00607 * \param output buffer for the output data. Should be able to hold at 00608 * least ilen + block_size. Cannot be the same buffer as 00609 * input! 00610 * \param olen length of the output data, will be filled with the 00611 * actual number of bytes written. 00612 * 00613 * \note Some ciphers don't use IVs nor NONCE. For these 00614 * ciphers, use iv = NULL and iv_len = 0. 00615 * 00616 * \returns 0 on success, or 00617 * MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA, or 00618 * MBEDTLS_ERR_CIPHER_FULL_BLOCK_EXPECTED if decryption 00619 * expected a full block but was not provided one, or 00620 * MBEDTLS_ERR_CIPHER_INVALID_PADDING on invalid padding 00621 * while decrypting, or 00622 * a cipher specific error code. 00623 */ 00624 int mbedtls_cipher_crypt( mbedtls_cipher_context_t *ctx, 00625 const unsigned char *iv, size_t iv_len, 00626 const unsigned char *input, size_t ilen, 00627 unsigned char *output, size_t *olen ); 00628 00629 #if defined(MBEDTLS_CIPHER_MODE_AEAD) 00630 /** 00631 * \brief Generic autenticated encryption (AEAD ciphers). 00632 * 00633 * \param ctx generic cipher context 00634 * \param iv IV to use (or NONCE_COUNTER for CTR-mode ciphers) 00635 * \param iv_len IV length for ciphers with variable-size IV; 00636 * discarded by ciphers with fixed-size IV. 00637 * \param ad Additional data to authenticate. 00638 * \param ad_len Length of ad. 00639 * \param input buffer holding the input data 00640 * \param ilen length of the input data 00641 * \param output buffer for the output data. 00642 * Should be able to hold at least ilen. 00643 * \param olen length of the output data, will be filled with the 00644 * actual number of bytes written. 00645 * \param tag buffer for the authentication tag 00646 * \param tag_len desired tag length 00647 * 00648 * \returns 0 on success, or 00649 * MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA, or 00650 * a cipher specific error code. 00651 */ 00652 int mbedtls_cipher_auth_encrypt( mbedtls_cipher_context_t *ctx, 00653 const unsigned char *iv, size_t iv_len, 00654 const unsigned char *ad, size_t ad_len, 00655 const unsigned char *input, size_t ilen, 00656 unsigned char *output, size_t *olen, 00657 unsigned char *tag, size_t tag_len ); 00658 00659 /** 00660 * \brief Generic autenticated decryption (AEAD ciphers). 00661 * 00662 * \param ctx generic cipher context 00663 * \param iv IV to use (or NONCE_COUNTER for CTR-mode ciphers) 00664 * \param iv_len IV length for ciphers with variable-size IV; 00665 * discarded by ciphers with fixed-size IV. 00666 * \param ad Additional data to be authenticated. 00667 * \param ad_len Length of ad. 00668 * \param input buffer holding the input data 00669 * \param ilen length of the input data 00670 * \param output buffer for the output data. 00671 * Should be able to hold at least ilen. 00672 * \param olen length of the output data, will be filled with the 00673 * actual number of bytes written. 00674 * \param tag buffer holding the authentication tag 00675 * \param tag_len length of the authentication tag 00676 * 00677 * \returns 0 on success, or 00678 * MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA, or 00679 * MBEDTLS_ERR_CIPHER_AUTH_FAILED if data isn't authentic, 00680 * or a cipher specific error code. 00681 * 00682 * \note If the data is not authentic, then the output buffer 00683 * is zeroed out to prevent the unauthentic plaintext to 00684 * be used by mistake, making this interface safer. 00685 */ 00686 int mbedtls_cipher_auth_decrypt( mbedtls_cipher_context_t *ctx, 00687 const unsigned char *iv, size_t iv_len, 00688 const unsigned char *ad, size_t ad_len, 00689 const unsigned char *input, size_t ilen, 00690 unsigned char *output, size_t *olen, 00691 const unsigned char *tag, size_t tag_len ); 00692 #endif /* MBEDTLS_CIPHER_MODE_AEAD */ 00693 00694 #ifdef __cplusplus 00695 } 00696 #endif 00697 00698 #endif /* MBEDTLS_CIPHER_H */
Generated on Tue Jul 12 2022 12:52:42 by
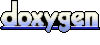