mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
camellia.h
Go to the documentation of this file.
00001 /** 00002 * \file camellia.h 00003 * 00004 * \brief Camellia block cipher 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_CAMELLIA_H 00024 #define MBEDTLS_CAMELLIA_H 00025 00026 #if !defined(MBEDTLS_CONFIG_FILE) 00027 #include "config.h" 00028 #else 00029 #include MBEDTLS_CONFIG_FILE 00030 #endif 00031 00032 #include <stddef.h> 00033 #include <stdint.h> 00034 00035 #define MBEDTLS_CAMELLIA_ENCRYPT 1 00036 #define MBEDTLS_CAMELLIA_DECRYPT 0 00037 00038 #define MBEDTLS_ERR_CAMELLIA_INVALID_KEY_LENGTH -0x0024 /**< Invalid key length. */ 00039 #define MBEDTLS_ERR_CAMELLIA_INVALID_INPUT_LENGTH -0x0026 /**< Invalid data input length. */ 00040 00041 #if !defined(MBEDTLS_CAMELLIA_ALT) 00042 // Regular implementation 00043 // 00044 00045 #ifdef __cplusplus 00046 extern "C" { 00047 #endif 00048 00049 /** 00050 * \brief CAMELLIA context structure 00051 */ 00052 typedef struct 00053 { 00054 int nr ; /*!< number of rounds */ 00055 uint32_t rk[68]; /*!< CAMELLIA round keys */ 00056 } 00057 mbedtls_camellia_context; 00058 00059 /** 00060 * \brief Initialize CAMELLIA context 00061 * 00062 * \param ctx CAMELLIA context to be initialized 00063 */ 00064 void mbedtls_camellia_init( mbedtls_camellia_context *ctx ); 00065 00066 /** 00067 * \brief Clear CAMELLIA context 00068 * 00069 * \param ctx CAMELLIA context to be cleared 00070 */ 00071 void mbedtls_camellia_free( mbedtls_camellia_context *ctx ); 00072 00073 /** 00074 * \brief CAMELLIA key schedule (encryption) 00075 * 00076 * \param ctx CAMELLIA context to be initialized 00077 * \param key encryption key 00078 * \param keybits must be 128, 192 or 256 00079 * 00080 * \return 0 if successful, or MBEDTLS_ERR_CAMELLIA_INVALID_KEY_LENGTH 00081 */ 00082 int mbedtls_camellia_setkey_enc( mbedtls_camellia_context *ctx, const unsigned char *key, 00083 unsigned int keybits ); 00084 00085 /** 00086 * \brief CAMELLIA key schedule (decryption) 00087 * 00088 * \param ctx CAMELLIA context to be initialized 00089 * \param key decryption key 00090 * \param keybits must be 128, 192 or 256 00091 * 00092 * \return 0 if successful, or MBEDTLS_ERR_CAMELLIA_INVALID_KEY_LENGTH 00093 */ 00094 int mbedtls_camellia_setkey_dec( mbedtls_camellia_context *ctx, const unsigned char *key, 00095 unsigned int keybits ); 00096 00097 /** 00098 * \brief CAMELLIA-ECB block encryption/decryption 00099 * 00100 * \param ctx CAMELLIA context 00101 * \param mode MBEDTLS_CAMELLIA_ENCRYPT or MBEDTLS_CAMELLIA_DECRYPT 00102 * \param input 16-byte input block 00103 * \param output 16-byte output block 00104 * 00105 * \return 0 if successful 00106 */ 00107 int mbedtls_camellia_crypt_ecb( mbedtls_camellia_context *ctx, 00108 int mode, 00109 const unsigned char input[16], 00110 unsigned char output[16] ); 00111 00112 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00113 /** 00114 * \brief CAMELLIA-CBC buffer encryption/decryption 00115 * Length should be a multiple of the block 00116 * size (16 bytes) 00117 * 00118 * \note Upon exit, the content of the IV is updated so that you can 00119 * call the function same function again on the following 00120 * block(s) of data and get the same result as if it was 00121 * encrypted in one call. This allows a "streaming" usage. 00122 * If on the other hand you need to retain the contents of the 00123 * IV, you should either save it manually or use the cipher 00124 * module instead. 00125 * 00126 * \param ctx CAMELLIA context 00127 * \param mode MBEDTLS_CAMELLIA_ENCRYPT or MBEDTLS_CAMELLIA_DECRYPT 00128 * \param length length of the input data 00129 * \param iv initialization vector (updated after use) 00130 * \param input buffer holding the input data 00131 * \param output buffer holding the output data 00132 * 00133 * \return 0 if successful, or 00134 * MBEDTLS_ERR_CAMELLIA_INVALID_INPUT_LENGTH 00135 */ 00136 int mbedtls_camellia_crypt_cbc( mbedtls_camellia_context *ctx, 00137 int mode, 00138 size_t length, 00139 unsigned char iv[16], 00140 const unsigned char *input, 00141 unsigned char *output ); 00142 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 00143 00144 #if defined(MBEDTLS_CIPHER_MODE_CFB) 00145 /** 00146 * \brief CAMELLIA-CFB128 buffer encryption/decryption 00147 * 00148 * Note: Due to the nature of CFB you should use the same key schedule for 00149 * both encryption and decryption. So a context initialized with 00150 * mbedtls_camellia_setkey_enc() for both MBEDTLS_CAMELLIA_ENCRYPT and CAMELLIE_DECRYPT. 00151 * 00152 * \note Upon exit, the content of the IV is updated so that you can 00153 * call the function same function again on the following 00154 * block(s) of data and get the same result as if it was 00155 * encrypted in one call. This allows a "streaming" usage. 00156 * If on the other hand you need to retain the contents of the 00157 * IV, you should either save it manually or use the cipher 00158 * module instead. 00159 * 00160 * \param ctx CAMELLIA context 00161 * \param mode MBEDTLS_CAMELLIA_ENCRYPT or MBEDTLS_CAMELLIA_DECRYPT 00162 * \param length length of the input data 00163 * \param iv_off offset in IV (updated after use) 00164 * \param iv initialization vector (updated after use) 00165 * \param input buffer holding the input data 00166 * \param output buffer holding the output data 00167 * 00168 * \return 0 if successful, or 00169 * MBEDTLS_ERR_CAMELLIA_INVALID_INPUT_LENGTH 00170 */ 00171 int mbedtls_camellia_crypt_cfb128( mbedtls_camellia_context *ctx, 00172 int mode, 00173 size_t length, 00174 size_t *iv_off, 00175 unsigned char iv[16], 00176 const unsigned char *input, 00177 unsigned char *output ); 00178 #endif /* MBEDTLS_CIPHER_MODE_CFB */ 00179 00180 #if defined(MBEDTLS_CIPHER_MODE_CTR) 00181 /** 00182 * \brief CAMELLIA-CTR buffer encryption/decryption 00183 * 00184 * Warning: You have to keep the maximum use of your counter in mind! 00185 * 00186 * Note: Due to the nature of CTR you should use the same key schedule for 00187 * both encryption and decryption. So a context initialized with 00188 * mbedtls_camellia_setkey_enc() for both MBEDTLS_CAMELLIA_ENCRYPT and MBEDTLS_CAMELLIA_DECRYPT. 00189 * 00190 * \param ctx CAMELLIA context 00191 * \param length The length of the data 00192 * \param nc_off The offset in the current stream_block (for resuming 00193 * within current cipher stream). The offset pointer to 00194 * should be 0 at the start of a stream. 00195 * \param nonce_counter The 128-bit nonce and counter. 00196 * \param stream_block The saved stream-block for resuming. Is overwritten 00197 * by the function. 00198 * \param input The input data stream 00199 * \param output The output data stream 00200 * 00201 * \return 0 if successful 00202 */ 00203 int mbedtls_camellia_crypt_ctr( mbedtls_camellia_context *ctx, 00204 size_t length, 00205 size_t *nc_off, 00206 unsigned char nonce_counter[16], 00207 unsigned char stream_block[16], 00208 const unsigned char *input, 00209 unsigned char *output ); 00210 #endif /* MBEDTLS_CIPHER_MODE_CTR */ 00211 00212 #ifdef __cplusplus 00213 } 00214 #endif 00215 00216 #else /* MBEDTLS_CAMELLIA_ALT */ 00217 #include "camellia_alt.h" 00218 #endif /* MBEDTLS_CAMELLIA_ALT */ 00219 00220 #ifdef __cplusplus 00221 extern "C" { 00222 #endif 00223 00224 /** 00225 * \brief Checkup routine 00226 * 00227 * \return 0 if successful, or 1 if the test failed 00228 */ 00229 int mbedtls_camellia_self_test( int verbose ); 00230 00231 #ifdef __cplusplus 00232 } 00233 #endif 00234 00235 #endif /* camellia.h */
Generated on Tue Jul 12 2022 12:52:41 by
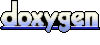