mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
camellia.c
00001 /* 00002 * Camellia implementation 00003 * 00004 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00005 * SPDX-License-Identifier: Apache-2.0 00006 * 00007 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00008 * not use this file except in compliance with the License. 00009 * You may obtain a copy of the License at 00010 * 00011 * http://www.apache.org/licenses/LICENSE-2.0 00012 * 00013 * Unless required by applicable law or agreed to in writing, software 00014 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00015 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00016 * See the License for the specific language governing permissions and 00017 * limitations under the License. 00018 * 00019 * This file is part of mbed TLS (https://tls.mbed.org) 00020 */ 00021 /* 00022 * The Camellia block cipher was designed by NTT and Mitsubishi Electric 00023 * Corporation. 00024 * 00025 * http://info.isl.ntt.co.jp/crypt/eng/camellia/dl/01espec.pdf 00026 */ 00027 00028 #if !defined(MBEDTLS_CONFIG_FILE) 00029 #include "mbedtls/config.h" 00030 #else 00031 #include MBEDTLS_CONFIG_FILE 00032 #endif 00033 00034 #if defined(MBEDTLS_CAMELLIA_C) 00035 00036 #include "mbedtls/camellia.h" 00037 00038 #include <string.h> 00039 00040 #if defined(MBEDTLS_SELF_TEST) 00041 #if defined(MBEDTLS_PLATFORM_C) 00042 #include "mbedtls/platform.h" 00043 #else 00044 #include <stdio.h> 00045 #define mbedtls_printf printf 00046 #endif /* MBEDTLS_PLATFORM_C */ 00047 #endif /* MBEDTLS_SELF_TEST */ 00048 00049 #if !defined(MBEDTLS_CAMELLIA_ALT) 00050 00051 /* Implementation that should never be optimized out by the compiler */ 00052 static void mbedtls_zeroize( void *v, size_t n ) { 00053 volatile unsigned char *p = v; while( n-- ) *p++ = 0; 00054 } 00055 00056 /* 00057 * 32-bit integer manipulation macros (big endian) 00058 */ 00059 #ifndef GET_UINT32_BE 00060 #define GET_UINT32_BE(n,b,i) \ 00061 { \ 00062 (n) = ( (uint32_t) (b)[(i) ] << 24 ) \ 00063 | ( (uint32_t) (b)[(i) + 1] << 16 ) \ 00064 | ( (uint32_t) (b)[(i) + 2] << 8 ) \ 00065 | ( (uint32_t) (b)[(i) + 3] ); \ 00066 } 00067 #endif 00068 00069 #ifndef PUT_UINT32_BE 00070 #define PUT_UINT32_BE(n,b,i) \ 00071 { \ 00072 (b)[(i) ] = (unsigned char) ( (n) >> 24 ); \ 00073 (b)[(i) + 1] = (unsigned char) ( (n) >> 16 ); \ 00074 (b)[(i) + 2] = (unsigned char) ( (n) >> 8 ); \ 00075 (b)[(i) + 3] = (unsigned char) ( (n) ); \ 00076 } 00077 #endif 00078 00079 static const unsigned char SIGMA_CHARS[6][8] = 00080 { 00081 { 0xa0, 0x9e, 0x66, 0x7f, 0x3b, 0xcc, 0x90, 0x8b }, 00082 { 0xb6, 0x7a, 0xe8, 0x58, 0x4c, 0xaa, 0x73, 0xb2 }, 00083 { 0xc6, 0xef, 0x37, 0x2f, 0xe9, 0x4f, 0x82, 0xbe }, 00084 { 0x54, 0xff, 0x53, 0xa5, 0xf1, 0xd3, 0x6f, 0x1c }, 00085 { 0x10, 0xe5, 0x27, 0xfa, 0xde, 0x68, 0x2d, 0x1d }, 00086 { 0xb0, 0x56, 0x88, 0xc2, 0xb3, 0xe6, 0xc1, 0xfd } 00087 }; 00088 00089 #if defined(MBEDTLS_CAMELLIA_SMALL_MEMORY) 00090 00091 static const unsigned char FSb[256] = 00092 { 00093 112,130, 44,236,179, 39,192,229,228,133, 87, 53,234, 12,174, 65, 00094 35,239,107,147, 69, 25,165, 33,237, 14, 79, 78, 29,101,146,189, 00095 134,184,175,143,124,235, 31,206, 62, 48,220, 95, 94,197, 11, 26, 00096 166,225, 57,202,213, 71, 93, 61,217, 1, 90,214, 81, 86,108, 77, 00097 139, 13,154,102,251,204,176, 45,116, 18, 43, 32,240,177,132,153, 00098 223, 76,203,194, 52,126,118, 5,109,183,169, 49,209, 23, 4,215, 00099 20, 88, 58, 97,222, 27, 17, 28, 50, 15,156, 22, 83, 24,242, 34, 00100 254, 68,207,178,195,181,122,145, 36, 8,232,168, 96,252,105, 80, 00101 170,208,160,125,161,137, 98,151, 84, 91, 30,149,224,255,100,210, 00102 16,196, 0, 72,163,247,117,219,138, 3,230,218, 9, 63,221,148, 00103 135, 92,131, 2,205, 74,144, 51,115,103,246,243,157,127,191,226, 00104 82,155,216, 38,200, 55,198, 59,129,150,111, 75, 19,190, 99, 46, 00105 233,121,167,140,159,110,188,142, 41,245,249,182, 47,253,180, 89, 00106 120,152, 6,106,231, 70,113,186,212, 37,171, 66,136,162,141,250, 00107 114, 7,185, 85,248,238,172, 10, 54, 73, 42,104, 60, 56,241,164, 00108 64, 40,211,123,187,201, 67,193, 21,227,173,244,119,199,128,158 00109 }; 00110 00111 #define SBOX1(n) FSb[(n)] 00112 #define SBOX2(n) (unsigned char)((FSb[(n)] >> 7 ^ FSb[(n)] << 1) & 0xff) 00113 #define SBOX3(n) (unsigned char)((FSb[(n)] >> 1 ^ FSb[(n)] << 7) & 0xff) 00114 #define SBOX4(n) FSb[((n) << 1 ^ (n) >> 7) &0xff] 00115 00116 #else /* MBEDTLS_CAMELLIA_SMALL_MEMORY */ 00117 00118 static const unsigned char FSb[256] = 00119 { 00120 112, 130, 44, 236, 179, 39, 192, 229, 228, 133, 87, 53, 234, 12, 174, 65, 00121 35, 239, 107, 147, 69, 25, 165, 33, 237, 14, 79, 78, 29, 101, 146, 189, 00122 134, 184, 175, 143, 124, 235, 31, 206, 62, 48, 220, 95, 94, 197, 11, 26, 00123 166, 225, 57, 202, 213, 71, 93, 61, 217, 1, 90, 214, 81, 86, 108, 77, 00124 139, 13, 154, 102, 251, 204, 176, 45, 116, 18, 43, 32, 240, 177, 132, 153, 00125 223, 76, 203, 194, 52, 126, 118, 5, 109, 183, 169, 49, 209, 23, 4, 215, 00126 20, 88, 58, 97, 222, 27, 17, 28, 50, 15, 156, 22, 83, 24, 242, 34, 00127 254, 68, 207, 178, 195, 181, 122, 145, 36, 8, 232, 168, 96, 252, 105, 80, 00128 170, 208, 160, 125, 161, 137, 98, 151, 84, 91, 30, 149, 224, 255, 100, 210, 00129 16, 196, 0, 72, 163, 247, 117, 219, 138, 3, 230, 218, 9, 63, 221, 148, 00130 135, 92, 131, 2, 205, 74, 144, 51, 115, 103, 246, 243, 157, 127, 191, 226, 00131 82, 155, 216, 38, 200, 55, 198, 59, 129, 150, 111, 75, 19, 190, 99, 46, 00132 233, 121, 167, 140, 159, 110, 188, 142, 41, 245, 249, 182, 47, 253, 180, 89, 00133 120, 152, 6, 106, 231, 70, 113, 186, 212, 37, 171, 66, 136, 162, 141, 250, 00134 114, 7, 185, 85, 248, 238, 172, 10, 54, 73, 42, 104, 60, 56, 241, 164, 00135 64, 40, 211, 123, 187, 201, 67, 193, 21, 227, 173, 244, 119, 199, 128, 158 00136 }; 00137 00138 static const unsigned char FSb2[256] = 00139 { 00140 224, 5, 88, 217, 103, 78, 129, 203, 201, 11, 174, 106, 213, 24, 93, 130, 00141 70, 223, 214, 39, 138, 50, 75, 66, 219, 28, 158, 156, 58, 202, 37, 123, 00142 13, 113, 95, 31, 248, 215, 62, 157, 124, 96, 185, 190, 188, 139, 22, 52, 00143 77, 195, 114, 149, 171, 142, 186, 122, 179, 2, 180, 173, 162, 172, 216, 154, 00144 23, 26, 53, 204, 247, 153, 97, 90, 232, 36, 86, 64, 225, 99, 9, 51, 00145 191, 152, 151, 133, 104, 252, 236, 10, 218, 111, 83, 98, 163, 46, 8, 175, 00146 40, 176, 116, 194, 189, 54, 34, 56, 100, 30, 57, 44, 166, 48, 229, 68, 00147 253, 136, 159, 101, 135, 107, 244, 35, 72, 16, 209, 81, 192, 249, 210, 160, 00148 85, 161, 65, 250, 67, 19, 196, 47, 168, 182, 60, 43, 193, 255, 200, 165, 00149 32, 137, 0, 144, 71, 239, 234, 183, 21, 6, 205, 181, 18, 126, 187, 41, 00150 15, 184, 7, 4, 155, 148, 33, 102, 230, 206, 237, 231, 59, 254, 127, 197, 00151 164, 55, 177, 76, 145, 110, 141, 118, 3, 45, 222, 150, 38, 125, 198, 92, 00152 211, 242, 79, 25, 63, 220, 121, 29, 82, 235, 243, 109, 94, 251, 105, 178, 00153 240, 49, 12, 212, 207, 140, 226, 117, 169, 74, 87, 132, 17, 69, 27, 245, 00154 228, 14, 115, 170, 241, 221, 89, 20, 108, 146, 84, 208, 120, 112, 227, 73, 00155 128, 80, 167, 246, 119, 147, 134, 131, 42, 199, 91, 233, 238, 143, 1, 61 00156 }; 00157 00158 static const unsigned char FSb3[256] = 00159 { 00160 56, 65, 22, 118, 217, 147, 96, 242, 114, 194, 171, 154, 117, 6, 87, 160, 00161 145, 247, 181, 201, 162, 140, 210, 144, 246, 7, 167, 39, 142, 178, 73, 222, 00162 67, 92, 215, 199, 62, 245, 143, 103, 31, 24, 110, 175, 47, 226, 133, 13, 00163 83, 240, 156, 101, 234, 163, 174, 158, 236, 128, 45, 107, 168, 43, 54, 166, 00164 197, 134, 77, 51, 253, 102, 88, 150, 58, 9, 149, 16, 120, 216, 66, 204, 00165 239, 38, 229, 97, 26, 63, 59, 130, 182, 219, 212, 152, 232, 139, 2, 235, 00166 10, 44, 29, 176, 111, 141, 136, 14, 25, 135, 78, 11, 169, 12, 121, 17, 00167 127, 34, 231, 89, 225, 218, 61, 200, 18, 4, 116, 84, 48, 126, 180, 40, 00168 85, 104, 80, 190, 208, 196, 49, 203, 42, 173, 15, 202, 112, 255, 50, 105, 00169 8, 98, 0, 36, 209, 251, 186, 237, 69, 129, 115, 109, 132, 159, 238, 74, 00170 195, 46, 193, 1, 230, 37, 72, 153, 185, 179, 123, 249, 206, 191, 223, 113, 00171 41, 205, 108, 19, 100, 155, 99, 157, 192, 75, 183, 165, 137, 95, 177, 23, 00172 244, 188, 211, 70, 207, 55, 94, 71, 148, 250, 252, 91, 151, 254, 90, 172, 00173 60, 76, 3, 53, 243, 35, 184, 93, 106, 146, 213, 33, 68, 81, 198, 125, 00174 57, 131, 220, 170, 124, 119, 86, 5, 27, 164, 21, 52, 30, 28, 248, 82, 00175 32, 20, 233, 189, 221, 228, 161, 224, 138, 241, 214, 122, 187, 227, 64, 79 00176 }; 00177 00178 static const unsigned char FSb4[256] = 00179 { 00180 112, 44, 179, 192, 228, 87, 234, 174, 35, 107, 69, 165, 237, 79, 29, 146, 00181 134, 175, 124, 31, 62, 220, 94, 11, 166, 57, 213, 93, 217, 90, 81, 108, 00182 139, 154, 251, 176, 116, 43, 240, 132, 223, 203, 52, 118, 109, 169, 209, 4, 00183 20, 58, 222, 17, 50, 156, 83, 242, 254, 207, 195, 122, 36, 232, 96, 105, 00184 170, 160, 161, 98, 84, 30, 224, 100, 16, 0, 163, 117, 138, 230, 9, 221, 00185 135, 131, 205, 144, 115, 246, 157, 191, 82, 216, 200, 198, 129, 111, 19, 99, 00186 233, 167, 159, 188, 41, 249, 47, 180, 120, 6, 231, 113, 212, 171, 136, 141, 00187 114, 185, 248, 172, 54, 42, 60, 241, 64, 211, 187, 67, 21, 173, 119, 128, 00188 130, 236, 39, 229, 133, 53, 12, 65, 239, 147, 25, 33, 14, 78, 101, 189, 00189 184, 143, 235, 206, 48, 95, 197, 26, 225, 202, 71, 61, 1, 214, 86, 77, 00190 13, 102, 204, 45, 18, 32, 177, 153, 76, 194, 126, 5, 183, 49, 23, 215, 00191 88, 97, 27, 28, 15, 22, 24, 34, 68, 178, 181, 145, 8, 168, 252, 80, 00192 208, 125, 137, 151, 91, 149, 255, 210, 196, 72, 247, 219, 3, 218, 63, 148, 00193 92, 2, 74, 51, 103, 243, 127, 226, 155, 38, 55, 59, 150, 75, 190, 46, 00194 121, 140, 110, 142, 245, 182, 253, 89, 152, 106, 70, 186, 37, 66, 162, 250, 00195 7, 85, 238, 10, 73, 104, 56, 164, 40, 123, 201, 193, 227, 244, 199, 158 00196 }; 00197 00198 #define SBOX1(n) FSb[(n)] 00199 #define SBOX2(n) FSb2[(n)] 00200 #define SBOX3(n) FSb3[(n)] 00201 #define SBOX4(n) FSb4[(n)] 00202 00203 #endif /* MBEDTLS_CAMELLIA_SMALL_MEMORY */ 00204 00205 static const unsigned char shifts[2][4][4] = 00206 { 00207 { 00208 { 1, 1, 1, 1 }, /* KL */ 00209 { 0, 0, 0, 0 }, /* KR */ 00210 { 1, 1, 1, 1 }, /* KA */ 00211 { 0, 0, 0, 0 } /* KB */ 00212 }, 00213 { 00214 { 1, 0, 1, 1 }, /* KL */ 00215 { 1, 1, 0, 1 }, /* KR */ 00216 { 1, 1, 1, 0 }, /* KA */ 00217 { 1, 1, 0, 1 } /* KB */ 00218 } 00219 }; 00220 00221 static const signed char indexes[2][4][20] = 00222 { 00223 { 00224 { 0, 1, 2, 3, 8, 9, 10, 11, 38, 39, 00225 36, 37, 23, 20, 21, 22, 27, -1, -1, 26 }, /* KL -> RK */ 00226 { -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, 00227 -1, -1, -1, -1, -1, -1, -1, -1, -1, -1 }, /* KR -> RK */ 00228 { 4, 5, 6, 7, 12, 13, 14, 15, 16, 17, 00229 18, 19, -1, 24, 25, -1, 31, 28, 29, 30 }, /* KA -> RK */ 00230 { -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, 00231 -1, -1, -1, -1, -1, -1, -1, -1, -1, -1 } /* KB -> RK */ 00232 }, 00233 { 00234 { 0, 1, 2, 3, 61, 62, 63, 60, -1, -1, 00235 -1, -1, 27, 24, 25, 26, 35, 32, 33, 34 }, /* KL -> RK */ 00236 { -1, -1, -1, -1, 8, 9, 10, 11, 16, 17, 00237 18, 19, -1, -1, -1, -1, 39, 36, 37, 38 }, /* KR -> RK */ 00238 { -1, -1, -1, -1, 12, 13, 14, 15, 58, 59, 00239 56, 57, 31, 28, 29, 30, -1, -1, -1, -1 }, /* KA -> RK */ 00240 { 4, 5, 6, 7, 65, 66, 67, 64, 20, 21, 00241 22, 23, -1, -1, -1, -1, 43, 40, 41, 42 } /* KB -> RK */ 00242 } 00243 }; 00244 00245 static const signed char transposes[2][20] = 00246 { 00247 { 00248 21, 22, 23, 20, 00249 -1, -1, -1, -1, 00250 18, 19, 16, 17, 00251 11, 8, 9, 10, 00252 15, 12, 13, 14 00253 }, 00254 { 00255 25, 26, 27, 24, 00256 29, 30, 31, 28, 00257 18, 19, 16, 17, 00258 -1, -1, -1, -1, 00259 -1, -1, -1, -1 00260 } 00261 }; 00262 00263 /* Shift macro for 128 bit strings with rotation smaller than 32 bits (!) */ 00264 #define ROTL(DEST, SRC, SHIFT) \ 00265 { \ 00266 (DEST)[0] = (SRC)[0] << (SHIFT) ^ (SRC)[1] >> (32 - (SHIFT)); \ 00267 (DEST)[1] = (SRC)[1] << (SHIFT) ^ (SRC)[2] >> (32 - (SHIFT)); \ 00268 (DEST)[2] = (SRC)[2] << (SHIFT) ^ (SRC)[3] >> (32 - (SHIFT)); \ 00269 (DEST)[3] = (SRC)[3] << (SHIFT) ^ (SRC)[0] >> (32 - (SHIFT)); \ 00270 } 00271 00272 #define FL(XL, XR, KL, KR) \ 00273 { \ 00274 (XR) = ((((XL) & (KL)) << 1) | (((XL) & (KL)) >> 31)) ^ (XR); \ 00275 (XL) = ((XR) | (KR)) ^ (XL); \ 00276 } 00277 00278 #define FLInv(YL, YR, KL, KR) \ 00279 { \ 00280 (YL) = ((YR) | (KR)) ^ (YL); \ 00281 (YR) = ((((YL) & (KL)) << 1) | (((YL) & (KL)) >> 31)) ^ (YR); \ 00282 } 00283 00284 #define SHIFT_AND_PLACE(INDEX, OFFSET) \ 00285 { \ 00286 TK[0] = KC[(OFFSET) * 4 + 0]; \ 00287 TK[1] = KC[(OFFSET) * 4 + 1]; \ 00288 TK[2] = KC[(OFFSET) * 4 + 2]; \ 00289 TK[3] = KC[(OFFSET) * 4 + 3]; \ 00290 \ 00291 for( i = 1; i <= 4; i++ ) \ 00292 if( shifts[(INDEX)][(OFFSET)][i -1] ) \ 00293 ROTL(TK + i * 4, TK, ( 15 * i ) % 32); \ 00294 \ 00295 for( i = 0; i < 20; i++ ) \ 00296 if( indexes[(INDEX)][(OFFSET)][i] != -1 ) { \ 00297 RK[indexes[(INDEX)][(OFFSET)][i]] = TK[ i ]; \ 00298 } \ 00299 } 00300 00301 static void camellia_feistel( const uint32_t x[2], const uint32_t k[2], 00302 uint32_t z[2]) 00303 { 00304 uint32_t I0, I1; 00305 I0 = x[0] ^ k[0]; 00306 I1 = x[1] ^ k[1]; 00307 00308 I0 = ((uint32_t) SBOX1((I0 >> 24) & 0xFF) << 24) | 00309 ((uint32_t) SBOX2((I0 >> 16) & 0xFF) << 16) | 00310 ((uint32_t) SBOX3((I0 >> 8) & 0xFF) << 8) | 00311 ((uint32_t) SBOX4((I0 ) & 0xFF) ); 00312 I1 = ((uint32_t) SBOX2((I1 >> 24) & 0xFF) << 24) | 00313 ((uint32_t) SBOX3((I1 >> 16) & 0xFF) << 16) | 00314 ((uint32_t) SBOX4((I1 >> 8) & 0xFF) << 8) | 00315 ((uint32_t) SBOX1((I1 ) & 0xFF) ); 00316 00317 I0 ^= (I1 << 8) | (I1 >> 24); 00318 I1 ^= (I0 << 16) | (I0 >> 16); 00319 I0 ^= (I1 >> 8) | (I1 << 24); 00320 I1 ^= (I0 >> 8) | (I0 << 24); 00321 00322 z[0] ^= I1; 00323 z[1] ^= I0; 00324 } 00325 00326 void mbedtls_camellia_init( mbedtls_camellia_context *ctx ) 00327 { 00328 memset( ctx, 0, sizeof( mbedtls_camellia_context ) ); 00329 } 00330 00331 void mbedtls_camellia_free( mbedtls_camellia_context *ctx ) 00332 { 00333 if( ctx == NULL ) 00334 return; 00335 00336 mbedtls_zeroize( ctx, sizeof( mbedtls_camellia_context ) ); 00337 } 00338 00339 /* 00340 * Camellia key schedule (encryption) 00341 */ 00342 int mbedtls_camellia_setkey_enc( mbedtls_camellia_context *ctx, const unsigned char *key, 00343 unsigned int keybits ) 00344 { 00345 int idx; 00346 size_t i; 00347 uint32_t *RK; 00348 unsigned char t[64]; 00349 uint32_t SIGMA[6][2]; 00350 uint32_t KC[16]; 00351 uint32_t TK[20]; 00352 00353 RK = ctx->rk ; 00354 00355 memset( t, 0, 64 ); 00356 memset( RK, 0, sizeof(ctx->rk ) ); 00357 00358 switch( keybits ) 00359 { 00360 case 128: ctx->nr = 3; idx = 0; break; 00361 case 192: 00362 case 256: ctx->nr = 4; idx = 1; break; 00363 default : return( MBEDTLS_ERR_CAMELLIA_INVALID_KEY_LENGTH ); 00364 } 00365 00366 for( i = 0; i < keybits / 8; ++i ) 00367 t[i] = key[i]; 00368 00369 if( keybits == 192 ) { 00370 for( i = 0; i < 8; i++ ) 00371 t[24 + i] = ~t[16 + i]; 00372 } 00373 00374 /* 00375 * Prepare SIGMA values 00376 */ 00377 for( i = 0; i < 6; i++ ) { 00378 GET_UINT32_BE( SIGMA[i][0], SIGMA_CHARS[i], 0 ); 00379 GET_UINT32_BE( SIGMA[i][1], SIGMA_CHARS[i], 4 ); 00380 } 00381 00382 /* 00383 * Key storage in KC 00384 * Order: KL, KR, KA, KB 00385 */ 00386 memset( KC, 0, sizeof(KC) ); 00387 00388 /* Store KL, KR */ 00389 for( i = 0; i < 8; i++ ) 00390 GET_UINT32_BE( KC[i], t, i * 4 ); 00391 00392 /* Generate KA */ 00393 for( i = 0; i < 4; ++i ) 00394 KC[8 + i] = KC[i] ^ KC[4 + i]; 00395 00396 camellia_feistel( KC + 8, SIGMA[0], KC + 10 ); 00397 camellia_feistel( KC + 10, SIGMA[1], KC + 8 ); 00398 00399 for( i = 0; i < 4; ++i ) 00400 KC[8 + i] ^= KC[i]; 00401 00402 camellia_feistel( KC + 8, SIGMA[2], KC + 10 ); 00403 camellia_feistel( KC + 10, SIGMA[3], KC + 8 ); 00404 00405 if( keybits > 128 ) { 00406 /* Generate KB */ 00407 for( i = 0; i < 4; ++i ) 00408 KC[12 + i] = KC[4 + i] ^ KC[8 + i]; 00409 00410 camellia_feistel( KC + 12, SIGMA[4], KC + 14 ); 00411 camellia_feistel( KC + 14, SIGMA[5], KC + 12 ); 00412 } 00413 00414 /* 00415 * Generating subkeys 00416 */ 00417 00418 /* Manipulating KL */ 00419 SHIFT_AND_PLACE( idx, 0 ); 00420 00421 /* Manipulating KR */ 00422 if( keybits > 128 ) { 00423 SHIFT_AND_PLACE( idx, 1 ); 00424 } 00425 00426 /* Manipulating KA */ 00427 SHIFT_AND_PLACE( idx, 2 ); 00428 00429 /* Manipulating KB */ 00430 if( keybits > 128 ) { 00431 SHIFT_AND_PLACE( idx, 3 ); 00432 } 00433 00434 /* Do transpositions */ 00435 for( i = 0; i < 20; i++ ) { 00436 if( transposes[idx][i] != -1 ) { 00437 RK[32 + 12 * idx + i] = RK[transposes[idx][i]]; 00438 } 00439 } 00440 00441 return( 0 ); 00442 } 00443 00444 /* 00445 * Camellia key schedule (decryption) 00446 */ 00447 int mbedtls_camellia_setkey_dec( mbedtls_camellia_context *ctx, const unsigned char *key, 00448 unsigned int keybits ) 00449 { 00450 int idx, ret; 00451 size_t i; 00452 mbedtls_camellia_context cty; 00453 uint32_t *RK; 00454 uint32_t *SK; 00455 00456 mbedtls_camellia_init( &cty ); 00457 00458 /* Also checks keybits */ 00459 if( ( ret = mbedtls_camellia_setkey_enc( &cty, key, keybits ) ) != 0 ) 00460 goto exit; 00461 00462 ctx->nr = cty.nr ; 00463 idx = ( ctx->nr == 4 ); 00464 00465 RK = ctx->rk ; 00466 SK = cty.rk + 24 * 2 + 8 * idx * 2; 00467 00468 *RK++ = *SK++; 00469 *RK++ = *SK++; 00470 *RK++ = *SK++; 00471 *RK++ = *SK++; 00472 00473 for( i = 22 + 8 * idx, SK -= 6; i > 0; i--, SK -= 4 ) 00474 { 00475 *RK++ = *SK++; 00476 *RK++ = *SK++; 00477 } 00478 00479 SK -= 2; 00480 00481 *RK++ = *SK++; 00482 *RK++ = *SK++; 00483 *RK++ = *SK++; 00484 *RK++ = *SK++; 00485 00486 exit: 00487 mbedtls_camellia_free( &cty ); 00488 00489 return( ret ); 00490 } 00491 00492 /* 00493 * Camellia-ECB block encryption/decryption 00494 */ 00495 int mbedtls_camellia_crypt_ecb( mbedtls_camellia_context *ctx, 00496 int mode, 00497 const unsigned char input[16], 00498 unsigned char output[16] ) 00499 { 00500 int NR; 00501 uint32_t *RK, X[4]; 00502 00503 ( (void) mode ); 00504 00505 NR = ctx->nr ; 00506 RK = ctx->rk ; 00507 00508 GET_UINT32_BE( X[0], input, 0 ); 00509 GET_UINT32_BE( X[1], input, 4 ); 00510 GET_UINT32_BE( X[2], input, 8 ); 00511 GET_UINT32_BE( X[3], input, 12 ); 00512 00513 X[0] ^= *RK++; 00514 X[1] ^= *RK++; 00515 X[2] ^= *RK++; 00516 X[3] ^= *RK++; 00517 00518 while( NR ) { 00519 --NR; 00520 camellia_feistel( X, RK, X + 2 ); 00521 RK += 2; 00522 camellia_feistel( X + 2, RK, X ); 00523 RK += 2; 00524 camellia_feistel( X, RK, X + 2 ); 00525 RK += 2; 00526 camellia_feistel( X + 2, RK, X ); 00527 RK += 2; 00528 camellia_feistel( X, RK, X + 2 ); 00529 RK += 2; 00530 camellia_feistel( X + 2, RK, X ); 00531 RK += 2; 00532 00533 if( NR ) { 00534 FL(X[0], X[1], RK[0], RK[1]); 00535 RK += 2; 00536 FLInv(X[2], X[3], RK[0], RK[1]); 00537 RK += 2; 00538 } 00539 } 00540 00541 X[2] ^= *RK++; 00542 X[3] ^= *RK++; 00543 X[0] ^= *RK++; 00544 X[1] ^= *RK++; 00545 00546 PUT_UINT32_BE( X[2], output, 0 ); 00547 PUT_UINT32_BE( X[3], output, 4 ); 00548 PUT_UINT32_BE( X[0], output, 8 ); 00549 PUT_UINT32_BE( X[1], output, 12 ); 00550 00551 return( 0 ); 00552 } 00553 00554 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00555 /* 00556 * Camellia-CBC buffer encryption/decryption 00557 */ 00558 int mbedtls_camellia_crypt_cbc( mbedtls_camellia_context *ctx, 00559 int mode, 00560 size_t length, 00561 unsigned char iv[16], 00562 const unsigned char *input, 00563 unsigned char *output ) 00564 { 00565 int i; 00566 unsigned char temp[16]; 00567 00568 if( length % 16 ) 00569 return( MBEDTLS_ERR_CAMELLIA_INVALID_INPUT_LENGTH ); 00570 00571 if( mode == MBEDTLS_CAMELLIA_DECRYPT ) 00572 { 00573 while( length > 0 ) 00574 { 00575 memcpy( temp, input, 16 ); 00576 mbedtls_camellia_crypt_ecb( ctx, mode, input, output ); 00577 00578 for( i = 0; i < 16; i++ ) 00579 output[i] = (unsigned char)( output[i] ^ iv[i] ); 00580 00581 memcpy( iv, temp, 16 ); 00582 00583 input += 16; 00584 output += 16; 00585 length -= 16; 00586 } 00587 } 00588 else 00589 { 00590 while( length > 0 ) 00591 { 00592 for( i = 0; i < 16; i++ ) 00593 output[i] = (unsigned char)( input[i] ^ iv[i] ); 00594 00595 mbedtls_camellia_crypt_ecb( ctx, mode, output, output ); 00596 memcpy( iv, output, 16 ); 00597 00598 input += 16; 00599 output += 16; 00600 length -= 16; 00601 } 00602 } 00603 00604 return( 0 ); 00605 } 00606 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 00607 00608 #if defined(MBEDTLS_CIPHER_MODE_CFB) 00609 /* 00610 * Camellia-CFB128 buffer encryption/decryption 00611 */ 00612 int mbedtls_camellia_crypt_cfb128( mbedtls_camellia_context *ctx, 00613 int mode, 00614 size_t length, 00615 size_t *iv_off, 00616 unsigned char iv[16], 00617 const unsigned char *input, 00618 unsigned char *output ) 00619 { 00620 int c; 00621 size_t n = *iv_off; 00622 00623 if( mode == MBEDTLS_CAMELLIA_DECRYPT ) 00624 { 00625 while( length-- ) 00626 { 00627 if( n == 0 ) 00628 mbedtls_camellia_crypt_ecb( ctx, MBEDTLS_CAMELLIA_ENCRYPT, iv, iv ); 00629 00630 c = *input++; 00631 *output++ = (unsigned char)( c ^ iv[n] ); 00632 iv[n] = (unsigned char) c; 00633 00634 n = ( n + 1 ) & 0x0F; 00635 } 00636 } 00637 else 00638 { 00639 while( length-- ) 00640 { 00641 if( n == 0 ) 00642 mbedtls_camellia_crypt_ecb( ctx, MBEDTLS_CAMELLIA_ENCRYPT, iv, iv ); 00643 00644 iv[n] = *output++ = (unsigned char)( iv[n] ^ *input++ ); 00645 00646 n = ( n + 1 ) & 0x0F; 00647 } 00648 } 00649 00650 *iv_off = n; 00651 00652 return( 0 ); 00653 } 00654 #endif /* MBEDTLS_CIPHER_MODE_CFB */ 00655 00656 #if defined(MBEDTLS_CIPHER_MODE_CTR) 00657 /* 00658 * Camellia-CTR buffer encryption/decryption 00659 */ 00660 int mbedtls_camellia_crypt_ctr( mbedtls_camellia_context *ctx, 00661 size_t length, 00662 size_t *nc_off, 00663 unsigned char nonce_counter[16], 00664 unsigned char stream_block[16], 00665 const unsigned char *input, 00666 unsigned char *output ) 00667 { 00668 int c, i; 00669 size_t n = *nc_off; 00670 00671 while( length-- ) 00672 { 00673 if( n == 0 ) { 00674 mbedtls_camellia_crypt_ecb( ctx, MBEDTLS_CAMELLIA_ENCRYPT, nonce_counter, 00675 stream_block ); 00676 00677 for( i = 16; i > 0; i-- ) 00678 if( ++nonce_counter[i - 1] != 0 ) 00679 break; 00680 } 00681 c = *input++; 00682 *output++ = (unsigned char)( c ^ stream_block[n] ); 00683 00684 n = ( n + 1 ) & 0x0F; 00685 } 00686 00687 *nc_off = n; 00688 00689 return( 0 ); 00690 } 00691 #endif /* MBEDTLS_CIPHER_MODE_CTR */ 00692 #endif /* !MBEDTLS_CAMELLIA_ALT */ 00693 00694 #if defined(MBEDTLS_SELF_TEST) 00695 00696 /* 00697 * Camellia test vectors from: 00698 * 00699 * http://info.isl.ntt.co.jp/crypt/eng/camellia/technology.html: 00700 * http://info.isl.ntt.co.jp/crypt/eng/camellia/dl/cryptrec/intermediate.txt 00701 * http://info.isl.ntt.co.jp/crypt/eng/camellia/dl/cryptrec/t_camellia.txt 00702 * (For each bitlength: Key 0, Nr 39) 00703 */ 00704 #define CAMELLIA_TESTS_ECB 2 00705 00706 static const unsigned char camellia_test_ecb_key[3][CAMELLIA_TESTS_ECB][32] = 00707 { 00708 { 00709 { 0x01, 0x23, 0x45, 0x67, 0x89, 0xab, 0xcd, 0xef, 00710 0xfe, 0xdc, 0xba, 0x98, 0x76, 0x54, 0x32, 0x10 }, 00711 { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 00712 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 } 00713 }, 00714 { 00715 { 0x01, 0x23, 0x45, 0x67, 0x89, 0xab, 0xcd, 0xef, 00716 0xfe, 0xdc, 0xba, 0x98, 0x76, 0x54, 0x32, 0x10, 00717 0x00, 0x11, 0x22, 0x33, 0x44, 0x55, 0x66, 0x77 }, 00718 { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 00719 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 00720 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 } 00721 }, 00722 { 00723 { 0x01, 0x23, 0x45, 0x67, 0x89, 0xab, 0xcd, 0xef, 00724 0xfe, 0xdc, 0xba, 0x98, 0x76, 0x54, 0x32, 0x10, 00725 0x00, 0x11, 0x22, 0x33, 0x44, 0x55, 0x66, 0x77, 00726 0x88, 0x99, 0xaa, 0xbb, 0xcc, 0xdd, 0xee, 0xff }, 00727 { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 00728 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 00729 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 00730 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 } 00731 }, 00732 }; 00733 00734 static const unsigned char camellia_test_ecb_plain[CAMELLIA_TESTS_ECB][16] = 00735 { 00736 { 0x01, 0x23, 0x45, 0x67, 0x89, 0xab, 0xcd, 0xef, 00737 0xfe, 0xdc, 0xba, 0x98, 0x76, 0x54, 0x32, 0x10 }, 00738 { 0x00, 0x00, 0x00, 0x00, 0x02, 0x00, 0x00, 0x00, 00739 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 } 00740 }; 00741 00742 static const unsigned char camellia_test_ecb_cipher[3][CAMELLIA_TESTS_ECB][16] = 00743 { 00744 { 00745 { 0x67, 0x67, 0x31, 0x38, 0x54, 0x96, 0x69, 0x73, 00746 0x08, 0x57, 0x06, 0x56, 0x48, 0xea, 0xbe, 0x43 }, 00747 { 0x38, 0x3C, 0x6C, 0x2A, 0xAB, 0xEF, 0x7F, 0xDE, 00748 0x25, 0xCD, 0x47, 0x0B, 0xF7, 0x74, 0xA3, 0x31 } 00749 }, 00750 { 00751 { 0xb4, 0x99, 0x34, 0x01, 0xb3, 0xe9, 0x96, 0xf8, 00752 0x4e, 0xe5, 0xce, 0xe7, 0xd7, 0x9b, 0x09, 0xb9 }, 00753 { 0xD1, 0x76, 0x3F, 0xC0, 0x19, 0xD7, 0x7C, 0xC9, 00754 0x30, 0xBF, 0xF2, 0xA5, 0x6F, 0x7C, 0x93, 0x64 } 00755 }, 00756 { 00757 { 0x9a, 0xcc, 0x23, 0x7d, 0xff, 0x16, 0xd7, 0x6c, 00758 0x20, 0xef, 0x7c, 0x91, 0x9e, 0x3a, 0x75, 0x09 }, 00759 { 0x05, 0x03, 0xFB, 0x10, 0xAB, 0x24, 0x1E, 0x7C, 00760 0xF4, 0x5D, 0x8C, 0xDE, 0xEE, 0x47, 0x43, 0x35 } 00761 } 00762 }; 00763 00764 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00765 #define CAMELLIA_TESTS_CBC 3 00766 00767 static const unsigned char camellia_test_cbc_key[3][32] = 00768 { 00769 { 0x2B, 0x7E, 0x15, 0x16, 0x28, 0xAE, 0xD2, 0xA6, 00770 0xAB, 0xF7, 0x15, 0x88, 0x09, 0xCF, 0x4F, 0x3C } 00771 , 00772 { 0x8E, 0x73, 0xB0, 0xF7, 0xDA, 0x0E, 0x64, 0x52, 00773 0xC8, 0x10, 0xF3, 0x2B, 0x80, 0x90, 0x79, 0xE5, 00774 0x62, 0xF8, 0xEA, 0xD2, 0x52, 0x2C, 0x6B, 0x7B } 00775 , 00776 { 0x60, 0x3D, 0xEB, 0x10, 0x15, 0xCA, 0x71, 0xBE, 00777 0x2B, 0x73, 0xAE, 0xF0, 0x85, 0x7D, 0x77, 0x81, 00778 0x1F, 0x35, 0x2C, 0x07, 0x3B, 0x61, 0x08, 0xD7, 00779 0x2D, 0x98, 0x10, 0xA3, 0x09, 0x14, 0xDF, 0xF4 } 00780 }; 00781 00782 static const unsigned char camellia_test_cbc_iv[16] = 00783 00784 { 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 00785 0x08, 0x09, 0x0A, 0x0B, 0x0C, 0x0D, 0x0E, 0x0F } 00786 ; 00787 00788 static const unsigned char camellia_test_cbc_plain[CAMELLIA_TESTS_CBC][16] = 00789 { 00790 { 0x6B, 0xC1, 0xBE, 0xE2, 0x2E, 0x40, 0x9F, 0x96, 00791 0xE9, 0x3D, 0x7E, 0x11, 0x73, 0x93, 0x17, 0x2A }, 00792 { 0xAE, 0x2D, 0x8A, 0x57, 0x1E, 0x03, 0xAC, 0x9C, 00793 0x9E, 0xB7, 0x6F, 0xAC, 0x45, 0xAF, 0x8E, 0x51 }, 00794 { 0x30, 0xC8, 0x1C, 0x46, 0xA3, 0x5C, 0xE4, 0x11, 00795 0xE5, 0xFB, 0xC1, 0x19, 0x1A, 0x0A, 0x52, 0xEF } 00796 00797 }; 00798 00799 static const unsigned char camellia_test_cbc_cipher[3][CAMELLIA_TESTS_CBC][16] = 00800 { 00801 { 00802 { 0x16, 0x07, 0xCF, 0x49, 0x4B, 0x36, 0xBB, 0xF0, 00803 0x0D, 0xAE, 0xB0, 0xB5, 0x03, 0xC8, 0x31, 0xAB }, 00804 { 0xA2, 0xF2, 0xCF, 0x67, 0x16, 0x29, 0xEF, 0x78, 00805 0x40, 0xC5, 0xA5, 0xDF, 0xB5, 0x07, 0x48, 0x87 }, 00806 { 0x0F, 0x06, 0x16, 0x50, 0x08, 0xCF, 0x8B, 0x8B, 00807 0x5A, 0x63, 0x58, 0x63, 0x62, 0x54, 0x3E, 0x54 } 00808 }, 00809 { 00810 { 0x2A, 0x48, 0x30, 0xAB, 0x5A, 0xC4, 0xA1, 0xA2, 00811 0x40, 0x59, 0x55, 0xFD, 0x21, 0x95, 0xCF, 0x93 }, 00812 { 0x5D, 0x5A, 0x86, 0x9B, 0xD1, 0x4C, 0xE5, 0x42, 00813 0x64, 0xF8, 0x92, 0xA6, 0xDD, 0x2E, 0xC3, 0xD5 }, 00814 { 0x37, 0xD3, 0x59, 0xC3, 0x34, 0x98, 0x36, 0xD8, 00815 0x84, 0xE3, 0x10, 0xAD, 0xDF, 0x68, 0xC4, 0x49 } 00816 }, 00817 { 00818 { 0xE6, 0xCF, 0xA3, 0x5F, 0xC0, 0x2B, 0x13, 0x4A, 00819 0x4D, 0x2C, 0x0B, 0x67, 0x37, 0xAC, 0x3E, 0xDA }, 00820 { 0x36, 0xCB, 0xEB, 0x73, 0xBD, 0x50, 0x4B, 0x40, 00821 0x70, 0xB1, 0xB7, 0xDE, 0x2B, 0x21, 0xEB, 0x50 }, 00822 { 0xE3, 0x1A, 0x60, 0x55, 0x29, 0x7D, 0x96, 0xCA, 00823 0x33, 0x30, 0xCD, 0xF1, 0xB1, 0x86, 0x0A, 0x83 } 00824 } 00825 }; 00826 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 00827 00828 #if defined(MBEDTLS_CIPHER_MODE_CTR) 00829 /* 00830 * Camellia-CTR test vectors from: 00831 * 00832 * http://www.faqs.org/rfcs/rfc5528.html 00833 */ 00834 00835 static const unsigned char camellia_test_ctr_key[3][16] = 00836 { 00837 { 0xAE, 0x68, 0x52, 0xF8, 0x12, 0x10, 0x67, 0xCC, 00838 0x4B, 0xF7, 0xA5, 0x76, 0x55, 0x77, 0xF3, 0x9E }, 00839 { 0x7E, 0x24, 0x06, 0x78, 0x17, 0xFA, 0xE0, 0xD7, 00840 0x43, 0xD6, 0xCE, 0x1F, 0x32, 0x53, 0x91, 0x63 }, 00841 { 0x76, 0x91, 0xBE, 0x03, 0x5E, 0x50, 0x20, 0xA8, 00842 0xAC, 0x6E, 0x61, 0x85, 0x29, 0xF9, 0xA0, 0xDC } 00843 }; 00844 00845 static const unsigned char camellia_test_ctr_nonce_counter[3][16] = 00846 { 00847 { 0x00, 0x00, 0x00, 0x30, 0x00, 0x00, 0x00, 0x00, 00848 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x01 }, 00849 { 0x00, 0x6C, 0xB6, 0xDB, 0xC0, 0x54, 0x3B, 0x59, 00850 0xDA, 0x48, 0xD9, 0x0B, 0x00, 0x00, 0x00, 0x01 }, 00851 { 0x00, 0xE0, 0x01, 0x7B, 0x27, 0x77, 0x7F, 0x3F, 00852 0x4A, 0x17, 0x86, 0xF0, 0x00, 0x00, 0x00, 0x01 } 00853 }; 00854 00855 static const unsigned char camellia_test_ctr_pt[3][48] = 00856 { 00857 { 0x53, 0x69, 0x6E, 0x67, 0x6C, 0x65, 0x20, 0x62, 00858 0x6C, 0x6F, 0x63, 0x6B, 0x20, 0x6D, 0x73, 0x67 }, 00859 00860 { 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 00861 0x08, 0x09, 0x0A, 0x0B, 0x0C, 0x0D, 0x0E, 0x0F, 00862 0x10, 0x11, 0x12, 0x13, 0x14, 0x15, 0x16, 0x17, 00863 0x18, 0x19, 0x1A, 0x1B, 0x1C, 0x1D, 0x1E, 0x1F }, 00864 00865 { 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 00866 0x08, 0x09, 0x0A, 0x0B, 0x0C, 0x0D, 0x0E, 0x0F, 00867 0x10, 0x11, 0x12, 0x13, 0x14, 0x15, 0x16, 0x17, 00868 0x18, 0x19, 0x1A, 0x1B, 0x1C, 0x1D, 0x1E, 0x1F, 00869 0x20, 0x21, 0x22, 0x23 } 00870 }; 00871 00872 static const unsigned char camellia_test_ctr_ct[3][48] = 00873 { 00874 { 0xD0, 0x9D, 0xC2, 0x9A, 0x82, 0x14, 0x61, 0x9A, 00875 0x20, 0x87, 0x7C, 0x76, 0xDB, 0x1F, 0x0B, 0x3F }, 00876 { 0xDB, 0xF3, 0xC7, 0x8D, 0xC0, 0x83, 0x96, 0xD4, 00877 0xDA, 0x7C, 0x90, 0x77, 0x65, 0xBB, 0xCB, 0x44, 00878 0x2B, 0x8E, 0x8E, 0x0F, 0x31, 0xF0, 0xDC, 0xA7, 00879 0x2C, 0x74, 0x17, 0xE3, 0x53, 0x60, 0xE0, 0x48 }, 00880 { 0xB1, 0x9D, 0x1F, 0xCD, 0xCB, 0x75, 0xEB, 0x88, 00881 0x2F, 0x84, 0x9C, 0xE2, 0x4D, 0x85, 0xCF, 0x73, 00882 0x9C, 0xE6, 0x4B, 0x2B, 0x5C, 0x9D, 0x73, 0xF1, 00883 0x4F, 0x2D, 0x5D, 0x9D, 0xCE, 0x98, 0x89, 0xCD, 00884 0xDF, 0x50, 0x86, 0x96 } 00885 }; 00886 00887 static const int camellia_test_ctr_len[3] = 00888 { 16, 32, 36 }; 00889 #endif /* MBEDTLS_CIPHER_MODE_CTR */ 00890 00891 /* 00892 * Checkup routine 00893 */ 00894 int mbedtls_camellia_self_test( int verbose ) 00895 { 00896 int i, j, u, v; 00897 unsigned char key[32]; 00898 unsigned char buf[64]; 00899 unsigned char src[16]; 00900 unsigned char dst[16]; 00901 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00902 unsigned char iv[16]; 00903 #endif 00904 #if defined(MBEDTLS_CIPHER_MODE_CTR) 00905 size_t offset, len; 00906 unsigned char nonce_counter[16]; 00907 unsigned char stream_block[16]; 00908 #endif 00909 00910 mbedtls_camellia_context ctx; 00911 00912 memset( key, 0, 32 ); 00913 00914 for( j = 0; j < 6; j++ ) { 00915 u = j >> 1; 00916 v = j & 1; 00917 00918 if( verbose != 0 ) 00919 mbedtls_printf( " CAMELLIA-ECB-%3d (%s): ", 128 + u * 64, 00920 (v == MBEDTLS_CAMELLIA_DECRYPT) ? "dec" : "enc"); 00921 00922 for( i = 0; i < CAMELLIA_TESTS_ECB; i++ ) { 00923 memcpy( key, camellia_test_ecb_key[u][i], 16 + 8 * u ); 00924 00925 if( v == MBEDTLS_CAMELLIA_DECRYPT ) { 00926 mbedtls_camellia_setkey_dec( &ctx, key, 128 + u * 64 ); 00927 memcpy( src, camellia_test_ecb_cipher[u][i], 16 ); 00928 memcpy( dst, camellia_test_ecb_plain[i], 16 ); 00929 } else { /* MBEDTLS_CAMELLIA_ENCRYPT */ 00930 mbedtls_camellia_setkey_enc( &ctx, key, 128 + u * 64 ); 00931 memcpy( src, camellia_test_ecb_plain[i], 16 ); 00932 memcpy( dst, camellia_test_ecb_cipher[u][i], 16 ); 00933 } 00934 00935 mbedtls_camellia_crypt_ecb( &ctx, v, src, buf ); 00936 00937 if( memcmp( buf, dst, 16 ) != 0 ) 00938 { 00939 if( verbose != 0 ) 00940 mbedtls_printf( "failed\n" ); 00941 00942 return( 1 ); 00943 } 00944 } 00945 00946 if( verbose != 0 ) 00947 mbedtls_printf( "passed\n" ); 00948 } 00949 00950 if( verbose != 0 ) 00951 mbedtls_printf( "\n" ); 00952 00953 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00954 /* 00955 * CBC mode 00956 */ 00957 for( j = 0; j < 6; j++ ) 00958 { 00959 u = j >> 1; 00960 v = j & 1; 00961 00962 if( verbose != 0 ) 00963 mbedtls_printf( " CAMELLIA-CBC-%3d (%s): ", 128 + u * 64, 00964 ( v == MBEDTLS_CAMELLIA_DECRYPT ) ? "dec" : "enc" ); 00965 00966 memcpy( src, camellia_test_cbc_iv, 16 ); 00967 memcpy( dst, camellia_test_cbc_iv, 16 ); 00968 memcpy( key, camellia_test_cbc_key[u], 16 + 8 * u ); 00969 00970 if( v == MBEDTLS_CAMELLIA_DECRYPT ) { 00971 mbedtls_camellia_setkey_dec( &ctx, key, 128 + u * 64 ); 00972 } else { 00973 mbedtls_camellia_setkey_enc( &ctx, key, 128 + u * 64 ); 00974 } 00975 00976 for( i = 0; i < CAMELLIA_TESTS_CBC; i++ ) { 00977 00978 if( v == MBEDTLS_CAMELLIA_DECRYPT ) { 00979 memcpy( iv , src, 16 ); 00980 memcpy( src, camellia_test_cbc_cipher[u][i], 16 ); 00981 memcpy( dst, camellia_test_cbc_plain[i], 16 ); 00982 } else { /* MBEDTLS_CAMELLIA_ENCRYPT */ 00983 memcpy( iv , dst, 16 ); 00984 memcpy( src, camellia_test_cbc_plain[i], 16 ); 00985 memcpy( dst, camellia_test_cbc_cipher[u][i], 16 ); 00986 } 00987 00988 mbedtls_camellia_crypt_cbc( &ctx, v, 16, iv, src, buf ); 00989 00990 if( memcmp( buf, dst, 16 ) != 0 ) 00991 { 00992 if( verbose != 0 ) 00993 mbedtls_printf( "failed\n" ); 00994 00995 return( 1 ); 00996 } 00997 } 00998 00999 if( verbose != 0 ) 01000 mbedtls_printf( "passed\n" ); 01001 } 01002 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 01003 01004 if( verbose != 0 ) 01005 mbedtls_printf( "\n" ); 01006 01007 #if defined(MBEDTLS_CIPHER_MODE_CTR) 01008 /* 01009 * CTR mode 01010 */ 01011 for( i = 0; i < 6; i++ ) 01012 { 01013 u = i >> 1; 01014 v = i & 1; 01015 01016 if( verbose != 0 ) 01017 mbedtls_printf( " CAMELLIA-CTR-128 (%s): ", 01018 ( v == MBEDTLS_CAMELLIA_DECRYPT ) ? "dec" : "enc" ); 01019 01020 memcpy( nonce_counter, camellia_test_ctr_nonce_counter[u], 16 ); 01021 memcpy( key, camellia_test_ctr_key[u], 16 ); 01022 01023 offset = 0; 01024 mbedtls_camellia_setkey_enc( &ctx, key, 128 ); 01025 01026 if( v == MBEDTLS_CAMELLIA_DECRYPT ) 01027 { 01028 len = camellia_test_ctr_len[u]; 01029 memcpy( buf, camellia_test_ctr_ct[u], len ); 01030 01031 mbedtls_camellia_crypt_ctr( &ctx, len, &offset, nonce_counter, stream_block, 01032 buf, buf ); 01033 01034 if( memcmp( buf, camellia_test_ctr_pt[u], len ) != 0 ) 01035 { 01036 if( verbose != 0 ) 01037 mbedtls_printf( "failed\n" ); 01038 01039 return( 1 ); 01040 } 01041 } 01042 else 01043 { 01044 len = camellia_test_ctr_len[u]; 01045 memcpy( buf, camellia_test_ctr_pt[u], len ); 01046 01047 mbedtls_camellia_crypt_ctr( &ctx, len, &offset, nonce_counter, stream_block, 01048 buf, buf ); 01049 01050 if( memcmp( buf, camellia_test_ctr_ct[u], len ) != 0 ) 01051 { 01052 if( verbose != 0 ) 01053 mbedtls_printf( "failed\n" ); 01054 01055 return( 1 ); 01056 } 01057 } 01058 01059 if( verbose != 0 ) 01060 mbedtls_printf( "passed\n" ); 01061 } 01062 01063 if( verbose != 0 ) 01064 mbedtls_printf( "\n" ); 01065 #endif /* MBEDTLS_CIPHER_MODE_CTR */ 01066 01067 return( 0 ); 01068 } 01069 01070 #endif /* MBEDTLS_SELF_TEST */ 01071 01072 #endif /* MBEDTLS_CAMELLIA_C */
Generated on Tue Jul 12 2022 12:52:41 by
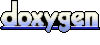