mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
base64.h
Go to the documentation of this file.
00001 /** 00002 * \file base64.h 00003 * 00004 * \brief RFC 1521 base64 encoding/decoding 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_BASE64_H 00024 #define MBEDTLS_BASE64_H 00025 00026 #include <stddef.h> 00027 00028 #define MBEDTLS_ERR_BASE64_BUFFER_TOO_SMALL -0x002A /**< Output buffer too small. */ 00029 #define MBEDTLS_ERR_BASE64_INVALID_CHARACTER -0x002C /**< Invalid character in input. */ 00030 00031 #ifdef __cplusplus 00032 extern "C" { 00033 #endif 00034 00035 /** 00036 * \brief Encode a buffer into base64 format 00037 * 00038 * \param dst destination buffer 00039 * \param dlen size of the destination buffer 00040 * \param olen number of bytes written 00041 * \param src source buffer 00042 * \param slen amount of data to be encoded 00043 * 00044 * \return 0 if successful, or MBEDTLS_ERR_BASE64_BUFFER_TOO_SMALL. 00045 * *olen is always updated to reflect the amount 00046 * of data that has (or would have) been written. 00047 * If that length cannot be represented, then no data is 00048 * written to the buffer and *olen is set to the maximum 00049 * length representable as a size_t. 00050 * 00051 * \note Call this function with dlen = 0 to obtain the 00052 * required buffer size in *olen 00053 */ 00054 int mbedtls_base64_encode( unsigned char *dst, size_t dlen, size_t *olen, 00055 const unsigned char *src, size_t slen ); 00056 00057 /** 00058 * \brief Decode a base64-formatted buffer 00059 * 00060 * \param dst destination buffer (can be NULL for checking size) 00061 * \param dlen size of the destination buffer 00062 * \param olen number of bytes written 00063 * \param src source buffer 00064 * \param slen amount of data to be decoded 00065 * 00066 * \return 0 if successful, MBEDTLS_ERR_BASE64_BUFFER_TOO_SMALL, or 00067 * MBEDTLS_ERR_BASE64_INVALID_CHARACTER if the input data is 00068 * not correct. *olen is always updated to reflect the amount 00069 * of data that has (or would have) been written. 00070 * 00071 * \note Call this function with *dst = NULL or dlen = 0 to obtain 00072 * the required buffer size in *olen 00073 */ 00074 int mbedtls_base64_decode( unsigned char *dst, size_t dlen, size_t *olen, 00075 const unsigned char *src, size_t slen ); 00076 00077 /** 00078 * \brief Checkup routine 00079 * 00080 * \return 0 if successful, or 1 if the test failed 00081 */ 00082 int mbedtls_base64_self_test( int verbose ); 00083 00084 #ifdef __cplusplus 00085 } 00086 #endif 00087 00088 #endif /* base64.h */
Generated on Tue Jul 12 2022 12:52:41 by
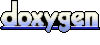