mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
arc4.h
Go to the documentation of this file.
00001 /** 00002 * \file arc4.h 00003 * 00004 * \brief The ARCFOUR stream cipher 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_ARC4_H 00024 #define MBEDTLS_ARC4_H 00025 00026 #if !defined(MBEDTLS_CONFIG_FILE) 00027 #include "config.h" 00028 #else 00029 #include MBEDTLS_CONFIG_FILE 00030 #endif 00031 00032 #include <stddef.h> 00033 00034 #if !defined(MBEDTLS_ARC4_ALT) 00035 // Regular implementation 00036 // 00037 00038 #ifdef __cplusplus 00039 extern "C" { 00040 #endif 00041 00042 /** 00043 * \brief ARC4 context structure 00044 */ 00045 typedef struct 00046 { 00047 int x ; /*!< permutation index */ 00048 int y ; /*!< permutation index */ 00049 unsigned char m[256]; /*!< permutation table */ 00050 } 00051 mbedtls_arc4_context; 00052 00053 /** 00054 * \brief Initialize ARC4 context 00055 * 00056 * \param ctx ARC4 context to be initialized 00057 */ 00058 void mbedtls_arc4_init( mbedtls_arc4_context *ctx ); 00059 00060 /** 00061 * \brief Clear ARC4 context 00062 * 00063 * \param ctx ARC4 context to be cleared 00064 */ 00065 void mbedtls_arc4_free( mbedtls_arc4_context *ctx ); 00066 00067 /** 00068 * \brief ARC4 key schedule 00069 * 00070 * \param ctx ARC4 context to be setup 00071 * \param key the secret key 00072 * \param keylen length of the key, in bytes 00073 */ 00074 void mbedtls_arc4_setup( mbedtls_arc4_context *ctx, const unsigned char *key, 00075 unsigned int keylen ); 00076 00077 /** 00078 * \brief ARC4 cipher function 00079 * 00080 * \param ctx ARC4 context 00081 * \param length length of the input data 00082 * \param input buffer holding the input data 00083 * \param output buffer for the output data 00084 * 00085 * \return 0 if successful 00086 */ 00087 int mbedtls_arc4_crypt( mbedtls_arc4_context *ctx, size_t length, const unsigned char *input, 00088 unsigned char *output ); 00089 00090 #ifdef __cplusplus 00091 } 00092 #endif 00093 00094 #else /* MBEDTLS_ARC4_ALT */ 00095 #include "arc4_alt.h" 00096 #endif /* MBEDTLS_ARC4_ALT */ 00097 00098 #ifdef __cplusplus 00099 extern "C" { 00100 #endif 00101 00102 /** 00103 * \brief Checkup routine 00104 * 00105 * \return 0 if successful, or 1 if the test failed 00106 */ 00107 int mbedtls_arc4_self_test( int verbose ); 00108 00109 #ifdef __cplusplus 00110 } 00111 #endif 00112 00113 #endif /* arc4.h */
Generated on Tue Jul 12 2022 12:52:41 by
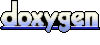