v1
Fork of Fork_Boss_Communication_Robot by
Embed:
(wiki syntax)
Show/hide line numbers
communication.h
00001 #ifndef ANDANTE_COMMUNICATION_H 00002 #define ANDANTE_COMMUNICATION_H 00003 00004 #include "mbed.h" 00005 #include "iSerial.h" 00006 #include "protocol.h" 00007 #include "Utilities.h" 00008 00009 #define ANDANTE_DEBUG 00010 00011 00012 class COMMUNICATION 00013 { 00014 private: 00015 Serial *pc; 00016 iSerial *serialCom; 00017 00018 public: 00019 /** Send the MX28 packet over the serial half duplex connection. 00020 * 00021 * @param packet The MX28 packet. 00022 * @return MX28_ERRBIT_NONE if succeeded, error code otherwise. 00023 */ 00024 uint8_t sendCommunicatePacket(ANDANTE_PROTOCOL_PACKET *packet); 00025 uint8_t receiveCommunicatePacket(ANDANTE_PROTOCOL_PACKET *packet); 00026 00027 /** Create an MX28 servo object connected to the specified serial half duplex pins, 00028 * with the specified baudrate. 00029 * 00030 * @param tx Send pin. 00031 * @param rx Receive pin. 00032 * @param baudrate The bus speed. 00033 */ 00034 COMMUNICATION(PinName tx, PinName rx, uint32_t baudRate, uint16_t tx_buff=1000, uint16_t rx_buff=1000 ); 00035 00036 /** Destroy an MX28 servo object 00037 */ 00038 ~COMMUNICATION(); 00039 }; 00040 00041 #endif // MX28_H
Generated on Tue Jul 12 2022 16:58:34 by
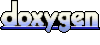