nexpaq module interface library
Fork of nexpaq_mdk by
Embed:
(wiki syntax)
Show/hide line numbers
nexpaq_mdk.h
00001 /* 00002 * np_module_mdk_v1.h 00003 * developer call the API of MDK by the include file. 00004 * Created on: July 14, 2016 00005 * Author: Alan.Lin 00006 * 00007 * Copyright: NexPack Ltd. 00008 */ 00009 00010 #ifndef NP_MODULE_MDK_V1_H_ 00011 #define NP_MODULE_MDK_V1_H_ 00012 00013 #include <stdint.h> 00014 00015 #ifdef __cplusplus 00016 extern "C" { 00017 #endif 00018 00019 typedef void (*my_VOID_UCUC)(uint8_t*, uint8_t); 00020 typedef void (*app_function)(void); 00021 00022 typedef struct { 00023 uint16_t command; 00024 my_VOID_UCUC function; 00025 } MDK_REGISTER_CMD; 00026 00027 #define MDK_REGISTER_SUCCESS 0x00 00028 #define MDK_REGISTER_FAILD 0x01 00029 00030 void delay_ms(uint32_t t_ms); 00031 /* 00032 * Description: API to make the app initial by the api 00033 * Parameter: np_app_setup-the initial function of APP 00034 * Return: null 00035 */ 00036 void np_api_setup(app_function np_app_setup); 00037 00038 /* 00039 * Description: initialize mdk 00040 * Parameter: null 00041 * Return: null 00042 */ 00043 void np_api_init(); 00044 00045 /* 00046 * Description: signal ready to start 00047 * Parameter: null 00048 * Return: null 00049 */ 00050 void np_api_start(); 00051 00052 /* 00053 * Description: check for bootloader request 00054 * Parameter: null 00055 * Return: null 00056 */ 00057 void np_api_bsl_chk(); 00058 00059 /* 00060 * Description: API to run the loop function of APP, "np_app_loop()" will run on while(1),running forever when software on app mode 00061 * Parameter: np_app_loop-the loop function of APP 00062 * Return: null 00063 */ 00064 void np_api_loop(app_function np_app_loop); 00065 00066 /* 00067 * Description: API to set app firmware version 00068 * Parameter: null 00069 * Return: null 00070 */ 00071 void np_api_set_app_version(uint8_t HV, uint8_t MV, uint8_t LV); 00072 /* 00073 * Description: API to register developer's command callback function 00074 * Parameter : 00075 * cmd_func_table:the callback function about the command. 00076 * The callback function will be called when MDK get the corresponding command. 00077 * num :The callback function number 00078 * Return : 0-success; 1-fail 00079 */ 00080 uint8_t np_api_register(MDK_REGISTER_CMD* cmd_func_table, uint8_t num); 00081 00082 /* 00083 * Description: API to upload data to Phone 00084 * Parameter: 00085 * rcmd : The command of the message. 00086 * pData: Pointer of the space for hold the parameter of the message. 00087 * pLen : The length of the "pData" 00088 * Return : 0-success; 1-fail 00089 */ 00090 uint8_t np_api_upload(uint16_t rcmd,uint8_t *pData, uint8_t pLen); 00091 00092 /* 00093 * Description: API to upload data to Gateway 00094 * rcmd : The command of the message. 00095 * pData: Pointer of the space for hold the parameter of the message. 00096 * pLen : The length of the "pData" 00097 * Return : 0-success; 1-fail 00098 */ 00099 uint8_t np_api_upload_to_station(uint16_t rcmd, uint8_t *pData, uint8_t pLen); 00100 00101 /* 00102 * Description: API to set a manually output address for next POST message 00103 * Parameter: address-the source address of next message 00104 * Return: null 00105 */ 00106 void np_api_set_post_address(uint8_t address); 00107 00108 #ifdef __cplusplus 00109 } 00110 #endif 00111 00112 #endif /* NP_MODULE_MDK_V1_H_ */ 00113
Generated on Wed Jul 13 2022 02:24:49 by
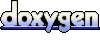