The Cayenne MQTT mbed Library provides functions to easily connect to the Cayenne IoT project builder.
Dependents: Cayenne-ESP8266Interface Cayenne-WIZnet_Library Cayenne-WIZnetInterface Cayenne-X-NUCLEO-IDW01M1 ... more
MQTTNetwork.h
00001 /* 00002 The MIT License(MIT) 00003 00004 Cayenne MQTT Client Library 00005 Copyright (c) 2016 myDevices 00006 00007 Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated 00008 documentation files(the "Software"), to deal in the Software without restriction, including without limitation 00009 the rights to use, copy, modify, merge, publish, distribute, sublicense, and / or sell copies of the Software, 00010 and to permit persons to whom the Software is furnished to do so, subject to the following conditions : 00011 The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. 00012 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE 00013 WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.IN NO EVENT SHALL THE AUTHORS OR 00014 COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00015 ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00016 */ 00017 00018 #ifndef _MQTTNETWORK_h 00019 #define _MQTTNETWORK_h 00020 00021 #include "mbed.h" 00022 #include "TCPSocketConnection.h" 00023 00024 /** 00025 * Networking class for use with MQTTClient. 00026 * @param Interface A network interface class with the methods: init, connect and disconnect. 00027 */ 00028 template<class Interface> 00029 class MQTTNetwork 00030 { 00031 public: 00032 /** 00033 * Construct the network interface. 00034 * @param[in] interface The network interface to use 00035 */ 00036 MQTTNetwork(Interface& interface) : _interface(interface) { 00037 } 00038 00039 /** 00040 * Connect the network interface. 00041 * @param[in] hostname Host for connection 00042 * @param[in] port Port for connection 00043 * @param[in] timeout_ms Timeout for the read operation, in milliseconds 00044 * @return 0 on success, -1 on failure. 00045 */ 00046 int connect(char* hostname, int port, int timeout_ms = 1000) { 00047 _interface.connect(); 00048 _socket.set_blocking(false, timeout_ms); 00049 return _socket.connect(hostname, port); 00050 } 00051 00052 /** 00053 * Disconnect the network interface. 00054 * @return true if disconnect was successful, false otherwise 00055 */ 00056 bool disconnect() 00057 { 00058 _socket.close(); 00059 return _interface.disconnect(); 00060 } 00061 00062 /** 00063 * Check if connected. 00064 * @return true if connected, false otherwise 00065 */ 00066 bool connected() 00067 { 00068 return _socket.is_connected(); 00069 } 00070 00071 /** 00072 * Read data from the network. 00073 * @param[out] buffer Buffer that receives the data 00074 * @param[in] len Buffer length 00075 * @param[in] timeout_ms Timeout for the read operation, in milliseconds 00076 * @return Number of bytes read, or a negative value if there was an error 00077 */ 00078 int read(unsigned char* buffer, int len, int timeout_ms) { 00079 _socket.set_blocking(false, timeout_ms); 00080 return _socket.receive((char*)buffer, len); 00081 } 00082 00083 /** 00084 * Write data to the network. 00085 * @param[in] buffer Buffer that contains data to write 00086 * @param[in] len Number of bytes to write 00087 * @param[in] timeout_ms Timeout for the write operation, in milliseconds 00088 * @return Number of bytes written, or a negative value if there was an error 00089 */ 00090 int write(unsigned char* buffer, int len, int timeout_ms) { 00091 _socket.set_blocking(false, timeout_ms); 00092 return _socket.send((char*)buffer, len); 00093 } 00094 00095 private: 00096 Interface& _interface; 00097 TCPSocketConnection _socket; 00098 }; 00099 00100 #endif
Generated on Wed Jul 13 2022 15:49:28 by
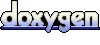